In the Golang programming language, a slice is a data structure that points to the underlying array. In some cases, you may need to remove elements from a slice. Because the slice is a reference type component, we can use the built-in functions of the Go language and the secondary encapsulation method of Golang ReSli to delete elements in the slice.
1. Use append and slice index to delete elements
We can use the append function to connect a new slice to the original slice, and also delete elements through the slice index. In this process, you can merge the parts that need to be deleted with the parts that don't need to be deleted into a new slice and assign it to the original slice variable. This is a common approach, but it can result in less efficient memory usage.
The following is an example that demonstrates how to use the append function and slice index to delete elements:
package main import "fmt" func main() { //定义一个原始切片 s := []int{1,2,3,4,5} //指定需要删除的下标(索引)位置 index := 2 //删除操作 s = append(s[:index], s[index+1:]...) fmt.Println(s) }
In the above code, we define a primitive slice s, which contains five elements. We use the index
variable to specify the subscript position that needs to be deleted, and use the append function to delete the specified element. Finally output the new slice.
2. Use slicing to traverse and delete elements
Using slicing has great advantages for more complex slicing operations. It allows us to add, delete, replace, and move elements as needed while still keeping the application's memory usage efficient. Here is an example that demonstrates how to use range to traverse a slice and delete elements using the slice index:
package main import "fmt" func main() { //定义一个原始切片 s := []int{1,2,3,4,5} //遍历切片 for i, v := range s { if v == 3 { s = append(s[:i], s[i+1:]...) break } } fmt.Println(s) }
In the above code, we use the range function to traverse the slice. In each loop, we check if the current value is equal to 3. If it's equal to 3, we remove it using the slice index.
3. Use Golang ReSli to delete elements
Golang ReSli is a powerful packaging library that provides some useful functions to extend standard Golang slicing. In this library, the ReSliDelSlice function for slice deletion is provided. The following is an example that demonstrates how to use ReSli to delete slice elements:
package main import ( "fmt" "github.com/fvbock/golang-essentials/essentials" ) func main() { //定义一个原始切片 s := []int{1,2,3,4,5} //删除操作 essentials.ReSliDelSlice(&s, 2) fmt.Println(s) }
In the above code, we use the ReSliDelSlice function provided in Golang ReSli to delete elements in the slice. In this function, the first parameter &s
is a pointer to the original slice, and the index specifying the item to be deleted is the second parameter, which is 2 in this example.
Summary
In this article, we introduced three different methods to delete elements in a slice. Regardless of which method you use, you should always pay attention to memory efficiency and code readability when operating on slices. Therefore, when removing elements, we should weigh the pros and cons of different paths and choose the method that best suits the current program.
The above is the detailed content of How to delete array in golang slice. For more information, please follow other related articles on the PHP Chinese website!
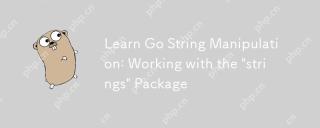
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
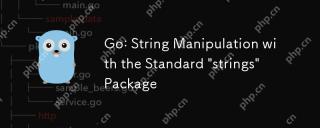
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
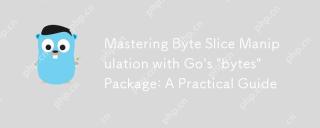
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
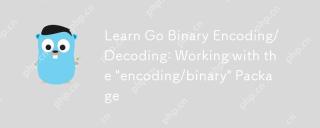
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
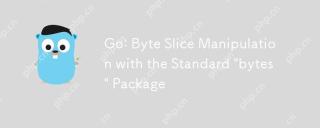
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
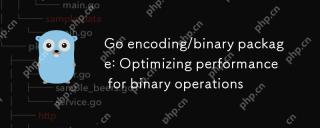
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
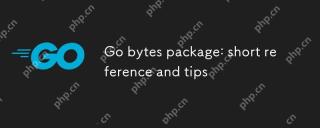
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
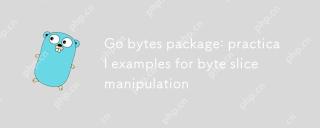
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
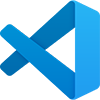
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
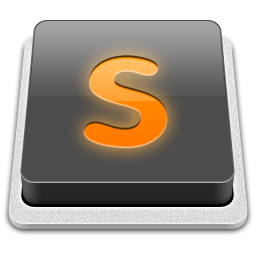
SublimeText3 Mac version
God-level code editing software (SublimeText3)
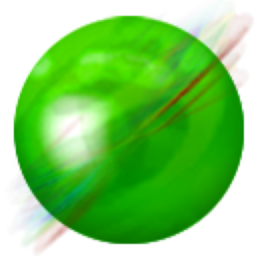
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
