In the process of writing applications, file deletion is a very common task. In many cases, PHP is a convenient, fast and effective way to delete files. In this article, we will take a deep dive into how to delete files using PHP language.
1. PHP delete files
In PHP, you can use the unlink()
function to delete files. The following is the basic syntax for deleting files using the unlink()
function:
unlink("filename");
where filename
is the name and full path of the file to be deleted.
The following is a sample program that demonstrates how to use the unlink()
function to delete a file:
<?php $file = "C:/xampp/htdocs/test.txt"; // 判断文件是否存在 if (file_exists($file)) { // 删除文件 if (unlink($file)) { echo "$file 删除成功。"; } else { echo "$file 删除失败。"; } } else { echo "$file 不存在。"; } ?>
The program first checks whether the specified file exists. If the file exists, use the unlink()
function to delete it. If the deletion is successful, the program will output a message that the file was deleted successfully. Otherwise, the program will output a message that the file deletion failed.
You can use a relative path or an absolute path to specify the file to be deleted. If you use a relative path, the file will be searched starting in the current working directory.
2. Delete multiple files
In addition to deleting a single file, PHP can also delete multiple files. Here is a sample program that demonstrates how to delete multiple files using PHP:
<?php $files = array("C:/xampp/htdocs/test1.txt", "C:/xampp/htdocs/test2.txt", "C:/xampp/htdocs/test3.txt"); foreach ($files as $file) { if (file_exists($file)) { if (unlink($file)) { echo "$file 删除成功。<br/>"; } else { echo "$file 删除失败。<br>"; } } } ?>
The program uses an array to store the files to be deleted. Then iterate through the array and use the unlink()
function to delete each file. If the file is successfully deleted, the program will output a file deletion success message. Otherwise, the program will output a message that the file deletion failed.
3. Delete a folder
In PHP, in addition to deleting files, you can also delete the entire folder and its contents. To delete a folder, you can use the rmdir()
function. The following is the basic syntax for deleting a folder using the rmdir()
function:
rmdir("dirname");
where dirname
is the name and full path of the folder to be deleted.
The following is a sample program that demonstrates how to delete a folder using the rmdir()
function:
<?php $dir = "C:/xampp/htdocs/mydir"; // 判断文件夹是否存在 if (file_exists($dir)) { // 删除文件夹 if (rmdir($dir)) { echo "$dir 删除成功。"; } else { echo "$dir 删除失败。"; } } else { echo "$dir 不存在。"; } ?>
The program first checks whether the specified folder exists. If the folder exists, use the rmdir()
function to delete it. If the deletion is successful, the program will output a message that the folder was deleted successfully. Otherwise, the program will output a message that the folder deletion failed.
It should be noted that the rmdir()
function can only delete empty folders. If a folder contains files or subfolders, it cannot be deleted. In order to delete a non-empty folder, you can use the unlink()
function to delete the files within it, and use recursive calls to delete subfolders.
4. Summary
In PHP, using the unlink()
function can delete files conveniently, quickly and effectively. If you want to delete multiple files or folders, you need to use a loop or recursive call to do it. Always make sure the file or folder exists before deleting it, otherwise an error will occur.
Hope this article can help you understand how to use PHP language to delete files.
The above is the detailed content of How to use php to delete files. For more information, please follow other related articles on the PHP Chinese website!
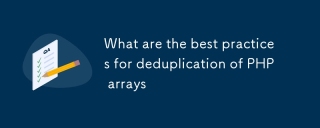
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
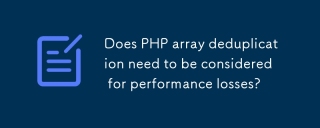
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
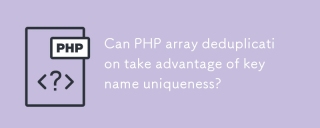
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
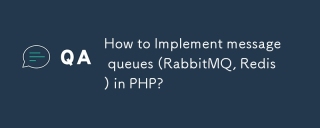
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
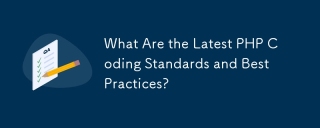
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
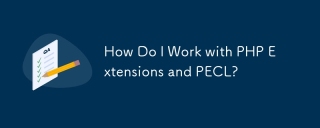
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
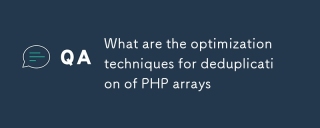
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
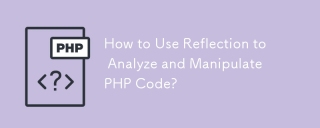
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
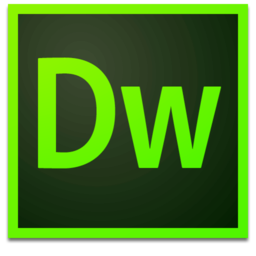
Dreamweaver Mac version
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!
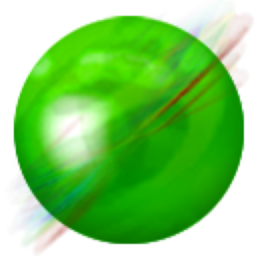
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
