The database is an important part of network programming. It is used to store and manage large amounts of data. In network applications, user information, product information and other data information need to be stored and managed, so database operation is an essential part of developing a website. As a widely used back-end server programming language, PHP also provides us with a series of database operation functions, allowing us to easily add, delete, and modify the database.
This article will briefly introduce the commonly used database addition, deletion and modification operations in PHP, including SQL statements, PDO classes and MySQLi classes. I hope that the introduction in this article can help developers better understand the operation of databases under PHP.
1. SQL statement
SQL statement is a general query language in the database, which is used to operate the database through statements to complete the addition, deletion and modification of data. , check and other operations. To operate the database through SQL statements in PHP, you can use the mysql_query() function, mysqli_query() function, and PDO preprocessing statements.
1.mysql_query() function
The mysql_query() function is a function used to execute SQL statements in PHP. To use this function, you need to connect to MySQL data, and then pass in SQL statements through this function to operate on the data. For example, insert data using the INSERT command:
<?php $con = mysql_connect("localhost","root","password"); mysql_select_db("my_db", $con); mysql_query("INSERT INTO Persons (FirstName, LastName, Age) VALUES ('Peter', 'Griffin', '35')"); mysql_close($con); ?>
2.mysqli_query() function
mysqli_query() function is a function in PHP for SQL statements with parameter bindings. Unlike the mysql_query() function, the mysqli_query() function supports SQL statements with parameter bindings. For example, inserting data using the INSERT command can be written as:
<?php $mysqli = new mysqli("localhost", "root", "password", "my_db"); $stmt = $mysqli->prepare("INSERT INTO Persons (FirstName, LastName, Age) VALUES (?, ?, ?)"); $stmt->bind_param("ssi", $fname, $lname, $age); $fname = "Peter"; $lname = "Griffin"; $age = 35; $stmt->execute(); $stmt->close(); $mysqli->close(); ?>
3.PDO prepared statement
PDO (PHP Data Objects) is a PHP extension that can be used to access and operate different types of Databases, including MySQL, Oracle and SQL Server, etc. Through PDO prepared statements, we can execute SQL statements based on the parameters passed in. For example, to insert data, use the INSERT command:
<?php $pdo = new PDO("mysql:host=localhost;port=3306;dbname=my_db", "root", "password"); $statement = $pdo->prepare("INSERT INTO Persons (FirstName, LastName, Age) VALUES (:fname, :lname, :age)"); $statement->bindParam(':fname', $fname); $statement->bindParam(':lname', $lname); $statement->bindParam(':age', $age); $fname = "Peter"; $lname = "Griffin"; $age = 35; $statement->execute(); $pdo = null; ?>
2. PDO class
The PDO class is a package in PHP used to interact with the database. To connect to the database through the PDO class, we can use the prepare() method to create a prepared statement, add parameters to the SQL statement, and then provide the SQL statement and data to the execute() method. The PDO class also provides methods such as beginTransaction() and commit() for opening and submitting transactions.
<?php $pdo = new PDO("mysql:host=localhost;port=3306;dbname=my_db", "root", "password"); $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $pdo->beginTransaction(); try { $statement = $pdo->prepare("INSERT INTO Persons (FirstName, LastName, Age) VALUES (?, ?, ?)"); $statement->bindParam(1, $fname); $statement->bindParam(2, $lname); $statement->bindParam(3, $age); $fname = "Peter"; $lname = "Griffin"; $age = 35; $statement->execute(); $pdo->commit(); } catch(PDOException $e) { $pdo->rollback(); echo "Error: " . $e->getMessage(); } $pdo = null; ?>
3. MySQLi class
The MySQLi class is an extension library for using MySQL in PHP. The functions it provides are more powerful and safer than the mysql_* function. It supports New MySQL server features such as transactions and stored procedures. To use the MySQLi class, you need to create an instance of the mysqli class through the new keyword, and then call the connect() method to connect to the MySQL server.
<?php $mysqli = new mysqli("localhost", "root", "password", "my_db"); $mysqli->query("INSERT INTO Persons (FirstName, LastName, Age) VALUES ('Peter', 'Griffin', '35')"); $mysqli->close(); ?>
Summary
This article mainly introduces the common database addition, deletion and modification operations in PHP, including SQL statements, PDO classes and MySQLi classes. Through the introduction of this article, we can learn about different operation methods and their advantages and disadvantages, and also note the role of parameter binding in security. Of course, in actual development, it is also necessary to use the most appropriate way to operate the database according to project needs to maximize user needs.
The above is the detailed content of A brief analysis of how PHP operates the database. For more information, please follow other related articles on the PHP Chinese website!
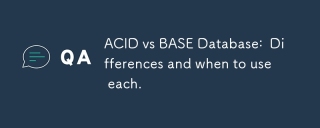
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
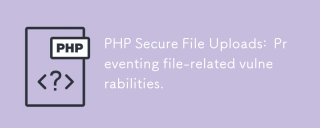
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
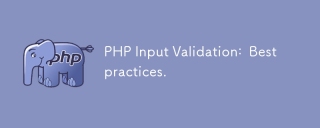
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
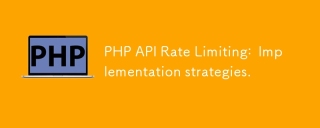
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
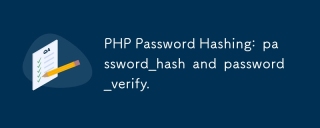
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
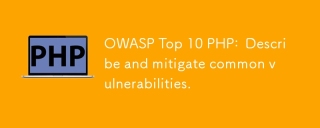
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
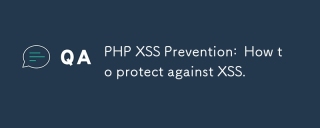
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
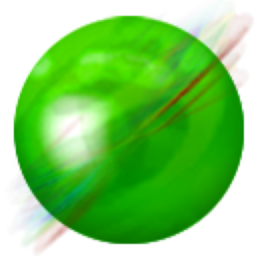
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor