What is Clean Architecture? How to implement it with Node?
What is Clean Architecture? This article will take you through Clean Architecture and talk about how to implement Clean Architecture using Node.js. I hope it will be helpful to you!
Clean Architecture
Clean Architecture is a software architecture pattern proposed by Robert C. Martin to divide the system into layer to achieve separation of concerns, making the system easier to understand, maintain and expand. This architecture divides the system into four levels, from inside to outside: entity layer, use case layer, presentation layer, infrastructure (repository, framework, etc.).
In this article, we will introduce how to implement Clean Architecture using Node.js and provide some sample code to demonstrate the key concepts of the architecture.
Next we will use the TypeScript project example (github.com/lulusir/cle…). The project uses a Monorepo structure and is managed using Rush.js. The server folder contains three sub-projects, namely core, koa and nestjs-app. Core is the core business logic, koa uses koa prisma as the underlying framework web project, and nestjs-app uses nestjs typeorm as the underlying framework. s project. The purpose is to demonstrate how the same business logic can bridge different frameworks. [Related tutorial recommendations: nodejs video tutorial, Programming teaching]
In this project, the entity layer contains entity objects and related business rules and logic, and the use case layer Contains the use cases and business logic of the system, the repository layer is responsible for saving and retrieving data, and the presentation layer is the http interface exposed to the outside.
Project function:
Realize a post publishing and browsing function
User creation and query
Publish, edit, query, delete posts
Project structure
├── server │ ├── core // 核心业务逻辑 │ │ └── src │ │ ├── domain │ │ ├── repository │ │ └── useCase │ ├── koa │ │ └── src │ │ ├── post │ │ └── user │ └── nestjs-app │ ├── src │ ├── post │ │ ├── dto │ │ └── entities │ └── user │ └── entities └── web
-
core: core is the code of the core business logic
- Domain: stores entity-related code, such as business-specific models, etc.
- Use Cases: stores business logic-related code Code, such as processing business logic, data validation, calling Repository, etc.
- Repository: Storage and related interfaces for external storage systems
- ##koa/nestjs-app: The actual consumer of core
- Implements the specific Router and Repository based on the interface of core
Use the ideas of DDD and Clean Architecture to separate business logic from framework implementation.
- Use the monorepo project structure to facilitate the management of multiple related projects.
- Provides multiple sample applications to make it easy to get started quickly.
- Based on TypeScript, improving code readability and maintainability.
- In core, we have the core business logic code. This level contains domains, repository interfaces, and use cases. Domains contain code related to entities, such as a specific business model. The repository contains relevant interfaces to external storage systems. Use cases contain code related to business logic, such as handling business logic, data validation, and calling repositories.
At the koa/nestjs-app level, we have actual consumers at the core level. They implement specific routers and repositories based on the interfaces provided by the core layer. One of the main advantages of using Clean Architecture is that it separates business logic from technical implementation. This means you can easily switch between different frameworks and libraries without changing the core business logic. In our example, we can switch between koa and nestjs-app while keeping the same core business logic.
Code implementationDefine entity layer
// server/core/src/domain/post.ts
import { User } from "./user";
export class Post {
author: User | null = null;
content: string = "";
updateAt: Date = new Date(); // timestamp;
createdAt: Date = new Date(); // timestamp;
title: string = "";
id: number = -1;
}
// server/core/src/domain/user.ts
export class User {
name: string = ''
email: string = ''
id: number = -1
}
Define storage interfaceimport { Post } from "../domain/post";
export interface IPostRepository {
create(post: Post): Promise<boolean>;
find(id: number): Promise<Post>;
update(post: Post): Promise<boolean>;
delete(post: Post): Promise<boolean>;
findMany(options: { authorId: number }): Promise<Post[]>;
}
...
import { User } from "../domain/user";
export interface IUserRepository {
create(user: User): Promise<boolean>;
find(id: number): Promise<User>;
}
Define the use case layerimport { User } from "../domain/user";
import { IUserRepository } from "../repository/user";
export class UCUser {
constructor(public userRepo: IUserRepository) {}
find(id: number) {
return this.userRepo.find(id);
}
create(name: string, email: string) {
if (email.includes("@test.com")) {
const user = new User();
user.email = email;
user.name = name;
return this.userRepo.create(user);
}
throw Error("Please use legal email");
}
}
koa projectImplement the storage layer interface in the koa project
// server/koa/src/user/user.repo.ts
import { PrismaClient } from "@prisma/client";
import { IUserRepository, User } from "core";
export class UserRepository implements IUserRepository {
prisma = new PrismaClient();
async create(user: User): Promise<boolean> {
const d = await this.prisma.user_orm_entity.create({
data: {
email: user.email,
name: user.name,
},
});
return !!d;
}
async find(id: number): Promise<User> {
const d = await this.prisma.user_orm_entity.findFirst({
where: {
id: id,
},
});
if (d) {
const u = new User();
u.email = d?.email;
u.id = d?.id;
u.name = d?.name;
return u;
}
throw Error("user id " + id + "not found");
}
}
Implementing HTTP routing (presentation layer) in koa project// server/koa/src/user/user.controller.ts
import Router from "@koa/router";
import { UCUser } from "core";
import { UserRepository } from "./user.repo";
export const userRouter = new Router({
prefix: "/user",
});
userRouter.get("/:id", async (ctx, next) => {
try {
const service = new UCUser(new UserRepository());
if (ctx.params.id) {
const u = await service.find(+ctx.params.id);
ctx.response.body = JSON.stringify(u);
}
} catch (e) {
ctx.throw(400, "some error on get user", e.message);
}
await next();
});
nest-js projectAn example of nestjs project can be found here Found in the path (
github.com/lulusir/cle…I won’t post the code here Please note that in the actual project, we will not put the core business logic in a separate warehouse (i.e. core), this is just to demonstrate the performance under different frameworks Use the same business logic By decoupling business logic from the framework, you can easily switch between different frameworks and libraries without changing the core business logic. If you want to build scalable and maintainable applications, Clean Architecture is definitely worth considering. If you want to demonstrate how to connect to other frameworks, you can put forward the project address in the comment area (github.com/lulusir/cle... I think it’s a good idea, You can give a star, thank you For more node-related knowledge, please visit:nodejs tutorial!Finally
The above is the detailed content of What is Clean Architecture? How to implement it with Node?. For more information, please follow other related articles on the PHP Chinese website!
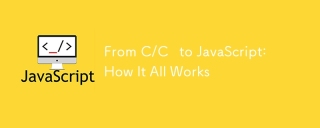
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
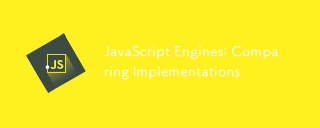
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
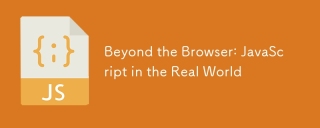
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
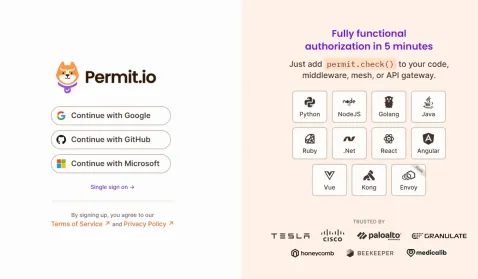
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
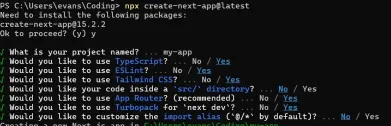
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
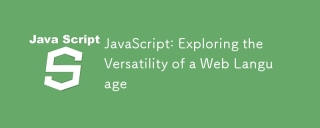
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
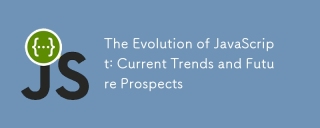
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
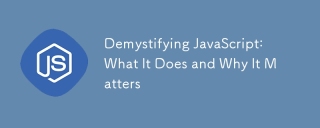
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
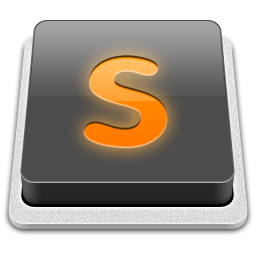
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
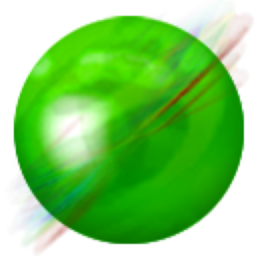
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment