In the Go language, you can use the built-in Len() function of the list to obtain the length of the list. The syntax is "list.Len()" and the return value is the length of the specified list. The Len() function can be used to calculate the length of arrays (including array pointers), slices, maps, channels, strings and other data types; note that structures (structs), integer Boolean, etc. cannot be passed to the len function as parameters .
The operating environment of this tutorial: Windows 7 system, GO version 1.18, Dell G3 computer.
In the Go language, you can use the built-in Len() function of the list to get the list length.
The syntax format for calculating list length:
Len() int
Use the built-in Len() function of the list to get the length of the list.
Example:
package main import ( "container/list" "fmt" ) func main() { //使用列表内置的 Len() 函数,获取列表的长度 listHaiCoder := list.New() listHaiCoder.PushFront("Hello") listHaiCoder.PushFront("HaiCoder") listHaiCoder.PushFront("嗨客网") len := listHaiCoder.Len() fmt.Println("Len =", len) }
Analysis:
We pass the list .New creates a list listHaiCoder, and then uses the PushFront function to insert three consecutive elements at the head of the list. Finally, we use the Len function built into the list to get the length of the list.
Explanation:
The len function is a built-in function in the Go language, so it can be called directly in the Go program.
Its function is to calculate the length of data types such as arrays (including array pointers), slices, maps, channels, strings, etc. Note that structures (structs), integer Boolean, etc. cannot be used as The parameters are passed to the len function.
Array or array pointer: return the number of elements
map and slice: the number of elements
channel: The number of unread elements in the channel
String: the number of bytes, not the number of characters in the string
When The value of V is a nil value, and len returns 0
sl := make([]int,0) sl = nil if sl == nil{ fmt.Println(len(sl)) //当slice类型为nil时,输出0 } s := "欢迎学习Go的len()函数"//14个字符 fmt.Println(len(s))//输入28
When processing strings, it is often necessary to know the number of characters in the string, but len() only counts the number of bytes in the string. So we can customize the function that handles the number of strings.
//rune是32位的int别外,可以代表一个unicode字符,因此,通过将字符串将成rune类型的切片,切片元素个数代表字符个数 func count(str string) int { r := []rune(str) return len(r) } s := "欢迎学习Go的len()函数"//14个字符 fmt.Println(count(s))//14 fmt.Println(len(s))//28
【Related recommendations: Go video tutorial, Programming teaching】
The above is the detailed content of How to get the list length in go language. For more information, please follow other related articles on the PHP Chinese website!
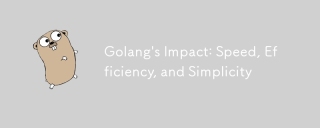
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
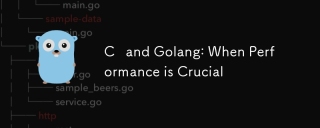
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
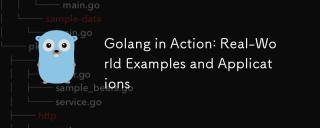
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
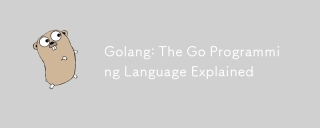
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
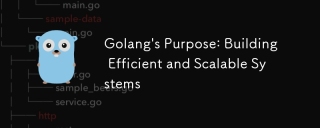
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
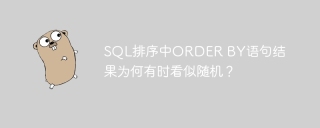
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
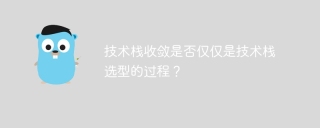
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
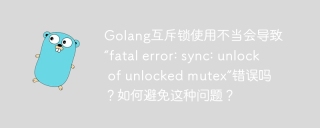
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
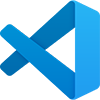
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
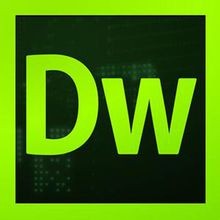
Dreamweaver CS6
Visual web development tools
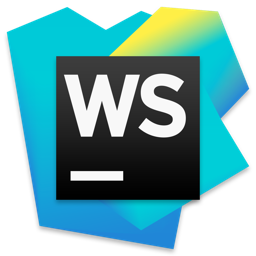
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment