


Let's talk about the principle of distributed locks and how Redis implements distributed locks
This article brings you relevant knowledge about redis, which mainly introduces what distributed locks are? How does Redis implement distributed locks? What conditions need to be met? Let’s take a look below, I hope it will be helpful to friends in need.
1. Basic principles of distributed locks
Distributed lock: A lock that is visible and mutually exclusive to multiple processes in a distributed system or cluster mode.
Conditions that distributed locks should meet:
- Visibility: Multiple threads can see the same result. Note: The visibility mentioned here does not refer to the memory visibility in concurrent programming, but only that multiple processes can perceive changes. Meaning
- Mutual exclusion: Mutual exclusion is the most basic condition for distributed locks, making the program execute serially
- High availability: The program is not easy to crash, and high availability is guaranteed at all times
- High performance: Since locking itself reduces performance, all distributed locks require higher locking performance and lock release performance
- Safety: Security is also part of the program An essential link
There are three common distributed locks:
Mysql: mysql itself has a lock mechanism , but due to the average performance of mysql itself, when using distributed locks, it is actually relatively rare to use mysql as a distributed lock
Redis: redis is very common as a distributed lock This way of usage, now enterprise-level development basically uses redis or zookeeper as a distributed lock. Using the setnx method, if the key is inserted successfully, it means that the lock is obtained. If someone inserts successfully, and others fail to insert, it means that the lock cannot be obtained. Lock, use this set of logic to implement distributed locks
Zookeeper: zookeeper is also a better solution for implementing distributed locks in enterprise-level development
2. Implementing distributed locks based on Redis
Two basic methods need to be implemented when implementing distributed locks:
-
Acquire lock:
- Mutual exclusion: ensure that only one thread can acquire the lock
- Non-blocking: try once, return true if successful, false if failed
-
Release lock:
- Manual release
- Timeout release: Add a timeout when acquiring the lock
Principle of distributed lock based on Redis:
SET resource_name my_random_value NX PX 30000
- resource_name: resource name, different locks can be distinguished according to different businesses
- my_random_value: Random value, the random value of each thread is different, used for verification when releasing the lock
- NX: The setting is successful when the key does not exist, and the setting is unsuccessful when the key exists
- PX: Automatic expiration time, if an abnormality occurs, the lock can expire.
Using the atomicity of NX, when multiple threads are concurrent, only one thread can be set successfully. Successful setting means obtaining the lock. Subsequent business processing can be performed; if an exception occurs and the lock validity period expires, the lock is automatically released;
Version 1
1. Define the ILock interface
public interface ILock extends AutoCloseable { /** * 尝试获取锁 * * @param timeoutSec 锁持有的超时时间,过期后自动释放 * @return true代表获取锁成功;false代表获取锁失败 */ boolean tryLock(long timeoutSec); /** * 释放锁 * @return */ void unLock(); }
2. Implement distributed lock based on Redis - RedisLock
public class SimpleRedisLock { private final StringRedisTemplate stringRedisTemplate; private final String name; public SimpleRedisLock(StringRedisTemplate stringRedisTemplate, String name) { this.stringRedisTemplate = stringRedisTemplate; this.name = name; } private static final String KEY_PREFIX = "lock:"; @Override public boolean tryLock(long timeoutSec) { //获取线程标识 String threadId = Thread.currentThread().getId(); //获取锁 Boolean success = stringRedisTemplate.opsForValue() .setIfAbsent(KEY_PREFIX + name, threadId, timeoutSec, TimeUnit.SECONDS); return Boolean.TRUE.equals(success); } @Override public void unLock() { //通过del删除锁 stringRedisTemplate.delete(KEY_PREFIX + name); } @Override public void close() { unLock(); } }
Lock accidental deletion problem
Problem description:
Thread 1 holding the lock is blocked inside the lock. At this time, the lock is automatically released after timeout. At this time, thread 2 tries to obtain the lock. Then, during the execution of thread 2 holding the lock, thread 1 reacts and continues execution. When it comes to deleting the lock logic, the lock that should belong to thread 2 will be deleted. This is the case of accidentally deleting the lock.
Solution:
When storing the lock, put the identifier of your own thread. When deleting the lock, determine whether the identifier of the current lock is the one you saved. If it is entered, it will be deleted. If it is not, it will not be deleted.
Version 2: Solve the problem of accidental lock deletion
public class SimpleRedisLock { private final StringRedisTemplate stringRedisTemplate; private final String name; public SimpleRedisLock(StringRedisTemplate stringRedisTemplate, String name) { this.stringRedisTemplate = stringRedisTemplate; this.name = name; } private static final String KEY_PREFIX = "lock:"; private static final String ID_PREFIX = UUID.randomUUID().toString(true) + "-"; @Override public boolean tryLock(long timeoutSec) { //获取线程标识 String threadId = ID_PREFIX + Thread.currentThread().getId(); //获取锁 Boolean success = stringRedisTemplate.opsForValue() .setIfAbsent(KEY_PREFIX + name, threadId, timeoutSec, TimeUnit.SECONDS); return Boolean.TRUE.equals(success); } @Override public void unLock() { // 获取线程标示 String threadId = ID_PREFIX + Thread.currentThread().getId(); // 获取锁中的标示 String id = stringRedisTemplate.opsForValue().get(KEY_PREFIX + name); // 判断标示是否一致 if(threadId.equals(id)) { // 释放锁 stringRedisTemplate.delete(KEY_PREFIX + name); } } @Override public void close() { unLock(); } }
The atomicity problem of lock release
Problem analysis:
The above release The lock code still has the problem of accidentally deleting the lock. When thread 1 obtains the thread ID in the lock and determines that it is its own lock based on the ID, the lock is automatically released when it expires. It happens that thread 2 tries to acquire the lock and gets the lock. When thread 1 still performs the operation of releasing the lock, the lock held by thread 2 is accidentally deleted.
The reason is that the lock release process implemented by java code is not an atomic operation and has thread safety issues.
Solution:
Redis provides Lua script function. Writing multiple Redis commands in one script can ensure the atomicity of execution of multiple commands.
Version 3: Call Lua script to transform distributed lock
public class SimpleRedisLock implements ILock { private final StringRedisTemplate stringRedisTemplate; private final String name; public SimpleRedisLock(StringRedisTemplate stringRedisTemplate, String name) { this.stringRedisTemplate = stringRedisTemplate; this.name = name; } private static final String KEY_PREFIX = "lock:"; private static final String ID_PREFIX = UUID.randomUUID().toString(true) + "-"; @Override public boolean tryLock(long timeoutSec) { //获取线程标识 String threadId = ID_PREFIX + Thread.currentThread().getId(); //获取锁 Boolean success = stringRedisTemplate.opsForValue() .setIfAbsent(KEY_PREFIX + name, threadId, timeoutSec, TimeUnit.SECONDS); return Boolean.TRUE.equals(success); } @Override public void unLock() { String script = "if redis.call("get",KEYS[1]) == ARGV[1] then\n" + " return redis.call("del",KEYS[1])\n" + "else\n" + " return 0\n" + "end"; //通过执行lua脚本实现锁删除,可以校验随机值 RedisScript<Boolean> redisScript = RedisScript.of(script, Boolean.class); stringRedisTemplate.execute(redisScript, Collections.singletonList(KEY_PREFIX + name), ID_PREFIX + Thread.currentThread().getId()); } @Override public void close() { unLock(); } }
Recommended learning: "Redis Video Tutorial"
The above is the detailed content of Let's talk about the principle of distributed locks and how Redis implements distributed locks. For more information, please follow other related articles on the PHP Chinese website!
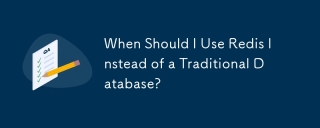
UseRedisinsteadofatraditionaldatabasewhenyourapplicationrequiresspeedandreal-timedataprocessing,suchasforcaching,sessionmanagement,orreal-timeanalytics.Redisexcelsin:1)Caching,reducingloadonprimarydatabases;2)Sessionmanagement,simplifyingdatahandling
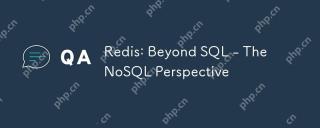
Redis goes beyond SQL databases because of its high performance and flexibility. 1) Redis achieves extremely fast read and write speed through memory storage. 2) It supports a variety of data structures, such as lists and collections, suitable for complex data processing. 3) Single-threaded model simplifies development, but high concurrency may become a bottleneck.
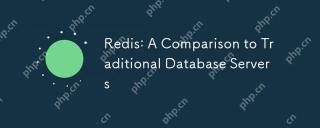
Redis is superior to traditional databases in high concurrency and low latency scenarios, but is not suitable for complex queries and transaction processing. 1.Redis uses memory storage, fast read and write speed, suitable for high concurrency and low latency requirements. 2. Traditional databases are based on disk, support complex queries and transaction processing, and have strong data consistency and persistence. 3. Redis is suitable as a supplement or substitute for traditional databases, but it needs to be selected according to specific business needs.
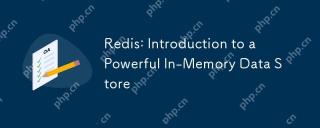
Redisisahigh-performancein-memorydatastructurestorethatexcelsinspeedandversatility.1)Itsupportsvariousdatastructureslikestrings,lists,andsets.2)Redisisanin-memorydatabasewithpersistenceoptions,ensuringfastperformanceanddatasafety.3)Itoffersatomicoper
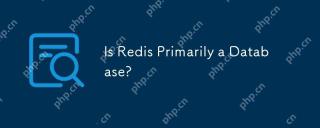
Redis is primarily a database, but it is more than just a database. 1. As a database, Redis supports persistence and is suitable for high-performance needs. 2. As a cache, Redis improves application response speed. 3. As a message broker, Redis supports publish-subscribe mode, suitable for real-time communication.
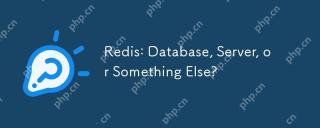
Redisisamultifacetedtoolthatservesasadatabase,server,andmore.Itfunctionsasanin-memorydatastructurestore,supportsvariousdatastructures,andcanbeusedasacache,messagebroker,sessionstorage,andfordistributedlocking.
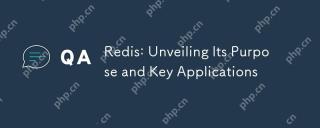
Redisisanopen-source,in-memorydatastructurestoreusedasadatabase,cache,andmessagebroker,excellinginspeedandversatility.Itiswidelyusedforcaching,real-timeanalytics,sessionmanagement,andleaderboardsduetoitssupportforvariousdatastructuresandfastdataacces
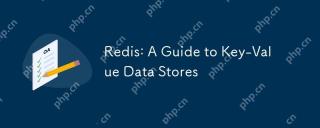
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1.Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing technology to handle requests efficiently. 5. Performance optimization strategies include LRU algorithm and cluster mode.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
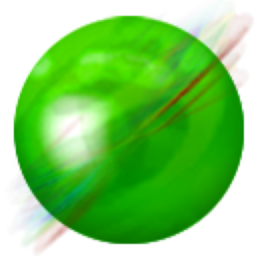
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
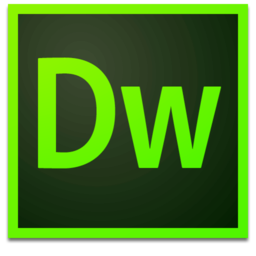
Dreamweaver Mac version
Visual web development tools
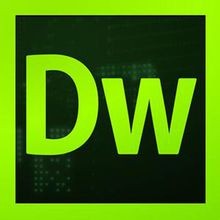
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use
