With the new version of V8 engine, Node.js supports async function features starting from 7.6. On October 31 this year, Node.js 8 also became a new long-term support version, so you can use async functions in your code with confidence. In this article, I will briefly introduce what async functions are and how they can change the way we write Node.js applications.
What is async function
Using async function, you can write Promise-based asynchronous code just like synchronous code. Once you define a function using the async keyword, you can use the await keyword within the function. When an async function is called, it returns a Promise. When the async function returns a value, the Promise is fulfilled; if an error is thrown in the function, the Promise is rejected. [Related tutorial recommendations: nodejs video tutorial, Programming teaching]
await keyword can be used to wait for a Promise to be resolved and return its realized value. If the value passed to await is not a Promise, it converts the value to a resolved Promise.
const rp = require('request-promise') async function main () { const result = await rp('https://google.com') const twenty = await 20 // 睡个1秒钟 await new Promise (resolve => { setTimeout(resolve, 1000) }) return result } main() .then(console.log) .catch(console.error)
Migrating to async functions
If your Node.js application is already using Promise, then you only need to rewrite the original chain call to your These Promises await.
If your application is still using callback functions, you should gradually switch to using async functions. You can use this new technology when developing some new features. When you have to call some old code, you can simply wrap it into a Promise and call it in the new way.
To do this, you can use the built-in util.promisify method:
const util = require('util') const {readFile} = require('fs') const readFileAsync = util.promisify(readFile) async function main () { const result = await readFileAsync('.gitignore') return result } main() .then(console.log) .catch(console.error)
Best Practices for Async Functions
Use async functions in express
express originally supports Promise, so using async functions in express is relatively simple:
const express = require('express') const app = express() app.get('/', async (request, response) => { // 在这里等待 Promise // 如果你只是在等待一个单独的 Promise,你其实可以直接将将它作为返回值返回,不需要使用 await 去等待。 const result = await getContent() response.send(result) }) app.listen(process.env.PORT)
But as Keith Smith pointed out, above There is a serious problem with this example - if the Promise is ultimately rejected, since there is no error handling here, the express route handler will hang.
To fix this problem, you should wrap your asynchronous handler in a function that handles errors:
const awaitHandlerFactory = (middleware) => { return async (req, res, next) => { try { await middleware(req, res, next) } catch (err) { next(err) } } } // 然后这样使用: app.get('/', awaitHandlerFactory(async (request, response) => { const result = await getContent() response.send(result) }))
Parallel execution
For example, you are writing a program where an operation requires two inputs, one from the database and the other from an external service:
async function main () { const user = await Users.fetch(userId) const product = await Products.fetch(productId) await makePurchase(user, product) }
In this example, what will happen?
Your code will first get the user,
then get the product,
and finally make the payment.
As you can see, since there is no interdependence between the first two steps, you can actually execute them in parallel. Here, you should use the Promise.all method:
async function main () { const [user, product] = await Promise.all([ Users.fetch(userId), Products.fetch(productId) ]) await makePurchase(user, product) }
And sometimes, you only need the return value of the fastest resolved Promise - in this case, you can use the Promise.race method.
For more node-related knowledge, please visit: nodejs tutorial!
The above is the detailed content of Let's talk about how to use async functions in Node.js. For more information, please follow other related articles on the PHP Chinese website!
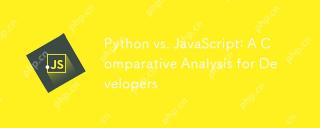
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
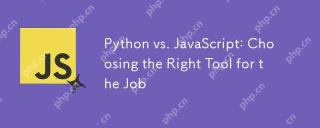
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
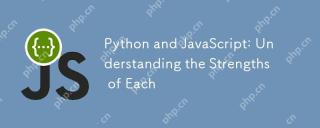
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
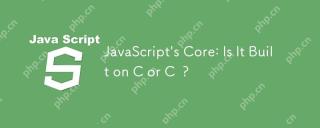
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
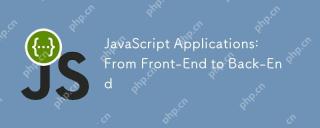
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
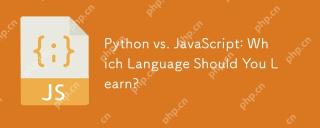
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
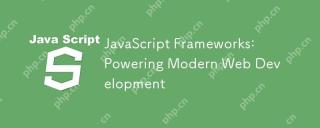
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
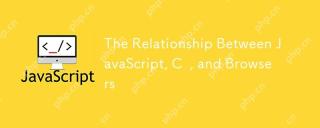
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
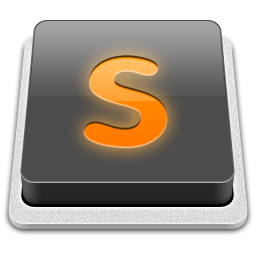
SublimeText3 Mac version
God-level code editing software (SublimeText3)
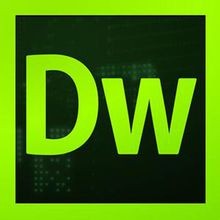
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
