Laravel Livewire is a great tool for implementing dynamic behavior on your page without writing JavaScript code directly. And, like any tool, it has many "hidden gems", including official documentation and useful extra tips from the developers. I decided to compile some of them in this article. let's start!
1. No need for render()
The typical render()
method looks like this:
// app/Http/Livewire/PostsShow.php class PostsShow extends Component { public function render() { return view('livewire.posts-show'); } }
However, if your render()
method is just a single line to render the default view, you can remove that render()
from the component method, it will still work, the method loading from the provider defaults to render()
. [Related recommendations: laravel video tutorial]
class PostsShow extends Component { //这个空组件仍将工作并加载Blade文件 }
2. Components in subfolders
If you want to Generate a component in the folder, such as app/Http/Livewire/Folder/Component.php
, you have two methods:
php artisan make:livewire Folder/Component
or
php artisan make:livewire folder.component
Please note that the One way is to capitalize the first letter, and the second way is to lowercase. In both cases, two files are generated:
- app/Http/Livewire/Folder/Component.php
- resources/views/livewire/folder/component.blade .php
If subfolders do not exist, they will be automatically created.
3. Components in non-default folders
If you use some external packages with Livewire components, your Livewire components may be located in In a different folder than the default app/Http/Livewire
. You may need to bind its name to an actual location.
This can usually be done in the app/Providers/AppServiceProvider.php
(or any service provider) method boot()
:
class AppServiceProvider extends ServiceProvider { public function boot() { Livewire::component('shopping-cart', \Modules\Shop\Http\Livewire\Cart::class); } }
4. Easily rename or move components
Don't worry if you make a typo when generating a component using make:livewire
. You don't need to rename the two files manually, there is a command for us to use.
For example, if you wrote php artisan make:livewire Prduct
, but you want "Product" and also decide to put it into a subfolder, you can use the following command Make improvements:
php artisan livewire:move Prduct Products/Show
The result will be like this:
COMPONENT MOVED CLASS: app/Http/Livewire/Prduct.php => app/Http/Livewire/Products/Show.php VIEW: resources/views/livewire/prduct.blade.php => resources/views/livewire/products/show.blade.php
5. Change the default component template
The Livewire component is using the default template Generated, the so-called "stub". They are hidden in the "vendor" folder of the Livewire package, but you can publish and edit them as needed.
Run this command:
php artisan livewire:stubs
You will find a new folder /stubs
containing some files. stubs/livewire.stub
Example:
<?php namespace [namespace]; use Livewire\Component; class [class] extends Component { public function render() { return view('[view]'); } }
For example, if you want to generate a component that does not use the render()
method, just change it from Delete it from the stub file, and then every time you run php artisan make:livewire Component
, it will get the "public stub" from your updated template.
6. Don’t create a method just to set a value
If you have a Click event that sets a value of a property, you can do this :
<button wire:click="showText">Show</button>
Then
class Show extends Component { public $showText = false; public function showText() { $this->showText = true; } }
But actually, you can assign a new value to the Livewire property directly from the vendor file without having a separate method in the Livewire component.
Here is the code:
<button wire:click="$set('showText', true)">Show</button>
So if your property is a boolean variable and you want to have a SHOW/FALSE button, you need to call $set
and Provide two parameters: your property name and the new value.
7. Go one step further: set true/false easily
After the previous tip, if your property is a boolean with true/false variable, and you want to have a show/hide button, you would do this:
<button wire:click="$toggle('showText')">Show/Hide</button>
NOTE: I would personally avoid using Livewire for this simple toggle effect because it will Add additional requests to the server.
Instead, it is better to use JavaScript, such as Alpine.js:
<div x-data="{ open: false }"> <button @click="open = true">Expand</button> <span x-show="open"> Content... </span> </div>
8. Three ways to minimize server requests
One of the main criticisms of Livewire is that it places too many requests on the server. If you have wire:model
in an input field, every keystroke may call the server to re-render the component. It would be very convenient if you have some real-time effects, such as Live Search. But generally, server requests can be very expensive in terms of performance.
However, it is very easy to customize this behavior of wire:model
.
wire:model.debounce
: By default, Livewire waits 150 milliseconds after a keystroke is entered before performing a request to the server. But you can override it:<input type="text" wire:model.debounce.1000ms="propertyName">
wire:model.lazy
:默认情况下,Livewire 会监听输入上的所有**事件,然后执行服务器请求。 通过提供lazy
指令,您可以告诉 Livewire 仅监听change
事件。 这意味着用户可以继续输入和更改,并且只有当用户点击离开该字段时才会触发服务器请求。wire:model.defer
:这不会在输入更改时触发服务器请求。 它将在内部保存新值并将其传递给下一个网络请求,该请求可能来自其他输入字段或其他按钮的点击。
9.自定义验证属性
Livewire 验证的工作方式与 Laravel 验证引擎非常相似,但有一些不同之处。在 Laravel 中,如果你想自定义属性的名称,你可以定义 attributes()
方法 在表单请求类中。
在 Livewire 中,方法不同。 在组件中,您需要定义一个名为「$validationAttributes」的属性并在那里分配键值数组:
class ContactForm extends Component { protected $validationAttributes = [ 'email' => 'email address' ]; // ... }
这对于常见的错误消息很有用,例如「需要字段 XYZ」。默认情况下,该 XYZ 被替换为字段名称,用户可能不会理解这个的词,因此应该将其替换为更清晰的错误消息。
10. 加载提示
从我所见,官方文档中描述但很少使用的东西。如果某些动作在屏幕上需要一段时间,则应该显示一些加载指示符,例如旋转的 gif,或者只是「正在加载数据…」的文本
在 Livewire 中,它不仅易于实现,而且还易于定制。
处理数据的最简单示例:当服务器发出请求时,它将显示「正在处理付款…」文本,直到服务器请求完成并返回结果。
<div> <button wire:click="checkout">Checkout</button> <div wire:loading> Processing Payment... </div> </div>
在实践中,我喜欢仅在需要一段时间时才显示此类加载指示器。在所有可能的情况下,每次都重新渲染 DOM 是没有意义的。 如果我们只在请求时间超过 500 毫秒时才这样做呢?
这很简单:
<div wire:loading.delay.longer>...</div>
还有可能使用 CSS 类来加载状态,将它们附加到特定的操作,等等:阅读 [官方文档](laravel-livewire.com/docs/2.x/load... #states#toggling-elements)。
11. 离线指示器
Livewire 的另一个记录在案但鲜为人知的功能是告诉用户他们的互联网连接是否丢失。如果您的应用程序使用实时数据或屏幕上的多次更新,这将是非常有用的:您可能会模糊网页的某些部分并显示「离线」文本。
这也很简单:
<div wire:offline> You are now offline. </div>
此外,正如我所提到的,您可以通过分配 CSS 类来模糊某些元素,如下所示:
<div wire:offline.class="bg-red-300"></div>
12. 使用 Bootstrap 框架分页
与 Laravel 类似,Livewire 默认使用来自 Tailwind 框架的分页样式。 幸运的是,它很容易覆盖,只需为属性提供不同的值:
class ShowPosts extends Component { use WithPagination; protected $paginationTheme = 'bootstrap';
您可以直接在 Livewire Github 存储库 中查看可用的分页设计。 在浏览时,我没有找到任何关于使用 Bootstrap 4 还是 Bootstrap 5 版本的信息。
13. No Mount:自动路由模型绑定
如果您想将对象传递给 Livewire 组件,这是一种典型的方法,使用 mount()
方法:
class ShowPost extends Component{ public $post; public function mount(Post $post) { $this->post = $post; } }
然后,在 Blade 的某个地方,使用:
@livewire('show-post', $post)
但是您是否知道,如果您为 Livewire 属性提供类型提示,路由模型绑定会自动生效?
class ShowPost extends Component{ public Post $post; }
就是这样,根本不需要mount()
方法。
14.删除时的确认提示
如果您有一个「删除」按钮,并且您想在执行操作之前调用确认弹窗在 JavaScript 中,则此代码将无法在 Livewire 中正常工作:
<button wire:click="delete($post->id)" onclick="return confirm('Are you sure?')">Delete</button>
对此有一些可能的解决方案,可能最优雅的方法是在 Livewire 事件发生之前停止它:
<button onclick="confirm('Are you sure?') || event.stopImmediatePropagation()" wire:click="delete($post->id)">Delete</button>
event.stopImmediatePropagation()
如果确认结果是假的,将停止调用LiveWire方法。
您可以在this Github issue discussion中找到一些其他可能的解决方案.
就是这样,一些LiveWire特征和小提示。希望对大家有用!
原文地址:https://laravel-news.com/laravel-livewire-tips-and-tricks
译文地址:https://learnku.com/laravel/t/66995
更多编程相关知识,请访问:编程教学!!
The above is the detailed content of How to use Laravel Livewire? 14 practical tips to share. For more information, please follow other related articles on the PHP Chinese website!

Selecting Laravel or Python depends on the project requirements: 1) If you need to quickly develop web applications and use ORM and authentication systems, choose Laravel; 2) If it involves data analysis, machine learning or scientific computing, choose Python.

Laravel is suitable for building web applications quickly, and Python is suitable for projects that require flexibility and versatility. 1) Laravel provides rich features such as ORM and routing, suitable for the PHP ecosystem. 2) Python is known for its concise syntax and a powerful library ecosystem, and is suitable for fields such as web development and data science.

Use Laravel and PHP to create dynamic websites efficiently and fun. 1) Laravel follows the MVC architecture, and the Blade template engine simplifies HTML writing. 2) The routing system and request processing mechanism make URL definition and user input processing simple. 3) EloquentORM simplifies database operations. 4) The use of database migration, CRUD operations and Blade templates are demonstrated through the blog system example. 5) Laravel provides powerful user authentication and authorization functions. 6) Debugging skills include using logging systems and Artisan tools. 7) Performance optimization suggestions include lazy loading and caching.

Laravel realizes full-stack development through the Blade template engine, EloquentORM, Artisan tools and LaravelMix: 1. Blade simplifies front-end development; 2. Eloquent simplifies database operations; 3. Artisan improves development efficiency; 4. LaravelMix manages front-end resources.

Laravel is a modern PHP-based framework that follows the MVC architecture model, provides rich tools and functions, and simplifies the web development process. 1) It contains EloquentORM for database interaction, 2) Artisan command line interface for fast code generation, 3) Blade template engine for efficient view development, 4) Powerful routing system for defining URL structure, 5) Authentication system for user management, 6) Event listening and broadcast for real-time functions, 7) Cache and queue systems for performance optimization, making it easier and more efficient to build and maintain modern web applications.

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
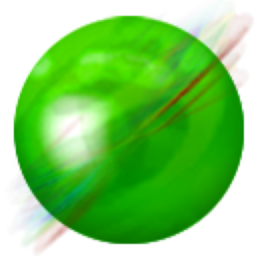
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
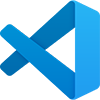
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.