es6 Usage of spread operator: 1. Copy array, syntax "[...array]"; 2. Merge arrays, syntax "[...array1,...array2]"; 3. Add elements to the array, the syntax is "[...array, 'element value']"; 4. Use it with the Math object to calculate the maximum value, minimum value or sum; 5. Pass infinite parameters to the function, the syntax is " const myFunc=(...args)=>{};"; 6. Convert the string to a character array, the syntax is "[...string]".
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
Introduction to the spread operator in es6
The spread operator ... is introduced in ES6 and expands the iterable object to its individual elements , the so-called iterable object is any object that can be traversed using a for of loop, such as: array (common method of array), string, Map (understanding Map), Set (how to use Set?), DOM node, etc.
It is like the inverse operation of the rest parameter, converting an array into a sequence of parameters separated by commas. The spread operator can be used in conjunction with normal function parameters, and an expression can also be placed behind it, but if it is followed by an empty array, it will have no effect.
let arr = []; arr.push(...[1,2,3,4,5]); console.log(arr); //[1,2,3,4,5] console.log(1, ...[2, 3, 4], 5) //1 2 3 4 5 console.log(...(1 > 0 ? ['a'] : [])); //a console.log([...[], 1]); //[1]
Meaning
The apply method of the alternative function
Since the spread operator can expand the array, the apply method is no longer needed and the array is converted to function parameters.
10 ways to use the spread operator (...
)
1. Copying an array
We can use the spread operator to copy an array, but be aware that this is a shallow copy.
const arr1 = [1,2,3]; const arr2 = [...arr1]; console.log(arr2); // [ 1, 2, 3 ]
This way we can copy a basic array, note that it does not work with multi-level arrays or arrays with dates or functions.
2. Merge arrays
Suppose we have two arrays that we want to merge into one. In the early stage, we can use concat
method, but now you can use the spread operator:
const arr1 = [1,2,3]; const arr2 = [4,5,6]; const arr3 = [...arr1, ...arr2]; console.log(arr3); // [ 1, 2, 3, 4, 5, 6 ]
We can also use different arrangements to indicate which should come first.
const arr3 = [...arr2, ...arr1]; console.log(arr3); [4, 5, 6, 1, 2, 3];
In addition, the expansion operator is also suitable for merging multiple arrays:
const output = [...arr1, ...arr2, ...arr3, ...arr4];
3. Add elements to the array
let arr1 = ['this', 'is', 'an']; arr1 = [...arr1, 'array']; console.log(arr1); // [ 'this', 'is', 'an', 'array' ]
4. Add attributes to the object
Suppose you have an object of user
, but it is missing an age
Attributes.
const user = { firstname: 'Chris', lastname: 'Bongers' };
To add age
to this user
object, we can again utilize the spread operator.
const output = {...user, age: 31};
5. Use the Math() function
Suppose we have an array of numbers and we want to get the maximum value among these numbers. Minimum value or sum.
const arr1 = [1, -1, 0, 5, 3];
To get the minimum value, we can use the spread operator and the Math.min
method.
const arr1 = [1, -1, 0, 5, 3]; const min = Math.min(...arr1); console.log(min); // -1
Similarly, to get the maximum value, you can do this:
const arr1 = [1, -1, 0, 5, 3]; const max = Math.max(...arr1); console.log(max); // 5
As you can see, the maximum value is 5
, if we delete 5
, it will return 3
.
You may be wondering, what happens if we don’t use the spread operator?
const arr1 = [1, -1, 0, 5, 3]; const max = Math.max(arr1); console.log(max); // NaN
This returns NaN because JavaScript doesn't know what the maximum value of the array is.
6. Rest parameter
Suppose we have a function with three parameters.
const myFunc(x1, x2, x3) => { console.log(x1); console.log(x2); console.log(x3); }
We can call this function as follows:
myFunc(1, 2, 3);
But what happens if we want to pass an array.
const arr1 = [1, 2, 3];
We can use the spread operator to expand this array into our function.
myFunc(...arr1); // 1 // 2 // 3
Here, we split the array into three separate parameters and pass them to the function.
const myFunc = (x1, x2, x3) => { console.log(x1); console.log(x2); console.log(x3); }; const arr1 = [1, 2, 3]; myFunc(...arr1); // 1 // 2 // 3
7. Pass unlimited parameters to the function
Suppose we have a function that accepts unlimited parameters as follows:
const myFunc = (...args) => { console.log(args); };
If we now call this function with multiple parameters, we will see the following situation:
myFunc(1, 'a', new Date());
Return:
[ 1, 'a', Date { __proto__: Date {} } ]
Then, we can dynamically loop through parameter.
8. Convert nodeList to an array
Suppose we use the spread operator to get all p## on the page #:
const el = [...document.querySelectorAll('p')]; console.log(el); // (3) [p, p, p]Here you can see that we got 3
p from the dom.
const el = [...document.querySelectorAll('p')]; el.forEach(item => { console.log(item); }); // <p></p> // <p></p> // <p></p>
9. Destructuring variables
Deconstructing objects
Suppose we have an objectuser:
const user = { firstname: 'Chris', lastname: 'Bongers', age: 31 };Now we can use the spread operator to break this into individual variables.
const {firstname, ...rest} = user; console.log(firstname); console.log(rest); // 'Chris' // { lastname: 'Bongers', age: 31 }Here, we deconstruct the
user object and
firstname into the
firstname variable and the rest of the object into
restVariables.
解构数组
const [currentMonth, ...others] = [7, 8, 9, 10, 11, 12]; console.log(currentMonth); // 7 console.log(others); // [ 8, 9, 10, 11, 12 ]
10、展开字符串(字符串转字符数组)
String 也是一个可迭代对象,所以也可以使用扩展运算符 ... 将其转为字符数组,如下:
const title = "china"; const charts = [...title]; console.log(charts); // [ 'c', 'h', 'i', 'n', 'a' ]
进而可以简单进行字符串截取,如下:
const title = "china"; const short = [...title]; short.length = 2; console.log(short.join("")); // ch
11、数组去重
与 Set 一起使用消除数组的重复项,如下:
const arrayNumbers = [1, 5, 9, 3, 5, 7, 10, 4, 5, 2, 5]; console.log(arrayNumbers); const newNumbers = [...new Set(arrayNumbers)]; console.log(newNumbers); // [ 1, 5, 9, 3, 7, 10, 4, 2 ]
12、打印日志
在打印可迭代对象的时候,需要打印每一项可以使用扩展符,如下:
const years = [2018, 2019, 2020, 2021]; console.log(...years); // 2018 2019 2020 2021
【相关推荐:web前端开发】
The above is the detailed content of How to use the spread operator in es6. For more information, please follow other related articles on the PHP Chinese website!

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
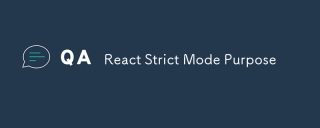
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.
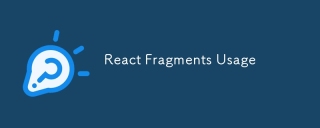
React Fragments allow grouping children without extra DOM nodes, enhancing structure, performance, and accessibility. They support keys for efficient list rendering.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
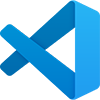
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
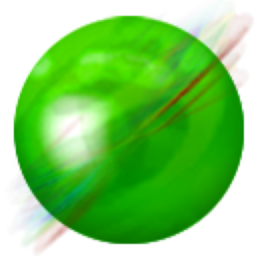
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
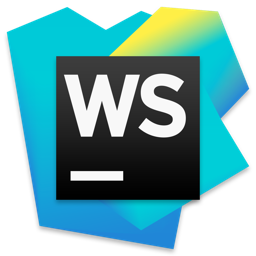
WebStorm Mac version
Useful JavaScript development tools