vuex composition
vuex mainly consists of the following five parts:
- State // Storage of variables and data
- Getter // Similar to computed properties
- Mutation // The only way to modify state
- Action // Call Mutation asynchronously
- Module // Modularize the store
vuex modules use
to create directories
In this example, I created two store files, which are profile.js
and custom.js
, a root file index.js
custom.js
const customs = { namespaced: true, // 创建命名空间 state: { // 存储变量 showAlert: false }, mutations: { // 定义修改state方法 CHANGESHOW: (state, params) => { state.showAlert = !state.showAlert } }, actions: { // 异步调用mutations setShow: ({ commit }) => { commit('CHANGESHOW') } }, getters: { // 将数据过滤输出 bodyShow: state => state.showAlert }}export default customs
profile.js
const profile = { namespaced: true, state: { name: 'common name', age: 18, bool: false }, mutations: { CHANGEMSG: (state, params) => { state.name = params }, CHANGEAGE: (state, params) => { state.name = params }, CHANGEBOOL: (state) => { state.bool = !state.bool } }, actions: { setName: ({ commit }) => { commit('CHANGEMSG', 'Vuex common name') }, setAge: ({ commit }) => { commit('CHANGEAGE', 81) }, setBool: ({ commit }) => { commit('CHANGEBOOL') } }, getters: { vuexName: state => state.name, vuexAge: state => state.age, vuexBool: state => state.bool }}export default common
index.js
import Vue from 'vue' import Vuex from 'vuex' // 引入子store import profile from './modules/profile' import customs from './modules/customs' // Vue.use(Vuex) const store = new Vuex.Store({ modules: { profile, customs } }) export default store // 导出store,以便于后续使用
Use it in the .vue file you need to use. The method is as follows
index.vue
<template> <div> name: <h5 id="vuexName">{{vuexName}}</h5> <button @click='setName'>chenge name</button> age: <h5 id="vuexAge">{{vuexAge}}</h5> <button @click='setAge'>chenge age</button> bool: <h5 id="vuexBool">{{vuexBool}}</h5> <button @click='setBool'>chenge bool</button> <br/> <span @click='setShow' style='display:inline-block;width:200px;height:30px;border:1px solid #999;border-radius:5px;text-align:center;line-height:30px;cursor: pointer;'>click me ,change showAlert</span> <em>{{bodyShow}}</em> </div> </template> <script> import { mapActions, mapGetters } from 'vuex' export default { computed: { ...mapGetters('profile', ['vuexName', 'vuexAge', 'vuexBool']), ...mapGetters('customs', ['bodyShow']) }, methods: { ...mapActions('customs', ['setShow']), ...mapActions('profile', ['setName', 'setAge', 'setBool']), } </script> <style> </style>
app.js
import Vue from 'vue'; import VueRouter from 'vue-router'; // style import './../../sass/app.scss'; // Components import Main from './Main.vue'; import routes from './routes'; // store import store from './store'; // 将store挂载到Vue Vue.use(VueRouter); const router = new VueRouter({ routes, saveScrollPosition: true, }); new Vue({ router, store, ...Main }).$mount('#app');
Initial rendering ⬇️
After clicking the button, the rendering ⬇️
At this point, the module usage process demonstration is completed! [Related recommendations: vue.js video tutorial]
The above is the detailed content of Vuex Module-Introduction to the use of state warehouse partitioning. For more information, please follow other related articles on the PHP Chinese website!
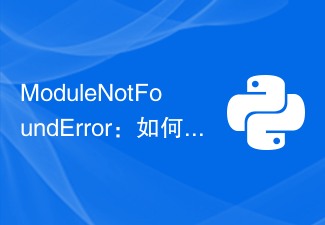
在Python的开发过程中,经常会遇到找不到模块的错误。这个错误的具体表现就是Python在导入模块的时候报出ModuleNotFoundError或者ImportError这两个错误之一。这种错误很困扰,会导致程序无法正常运行,因此在这篇文章里,我们将会探究这个错误的原因及其解决方法。ModuleNotFoundError和ImportError在Pyth
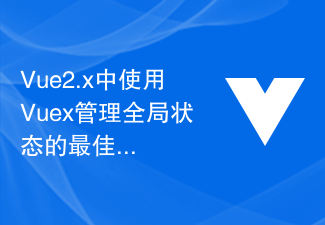
Vue2.x是目前最流行的前端框架之一,它提供了Vuex作为管理全局状态的解决方案。使用Vuex能够使得状态管理更加清晰、易于维护,下面将介绍Vuex的最佳实践,帮助开发者更好地使用Vuex以及提高代码质量。1.使用模块化组织状态Vuex使用单一状态树管理应用的全部状态,将状态从组件中抽离出来,使得状态管理更加清晰易懂。在具有较多状态的应用中,必须使用模块
![在Vue应用中使用vuex时出现“Error: [vuex] do not mutate vuex store state outside mutation handlers.”怎么解决?](https://img.php.cn/upload/article/000/000/164/168760467048976.jpg)
在Vue应用中,使用vuex是常见的状态管理方式。然而,在使用vuex时,我们有时可能会遇到这样的错误提示:“Error:[vuex]donotmutatevuexstorestateoutsidemutationhandlers.”这个错误提示是什么意思呢?为什么会出现这个错误提示?如何解决这个错误?本文将详细介绍这个问题。错误提示的含
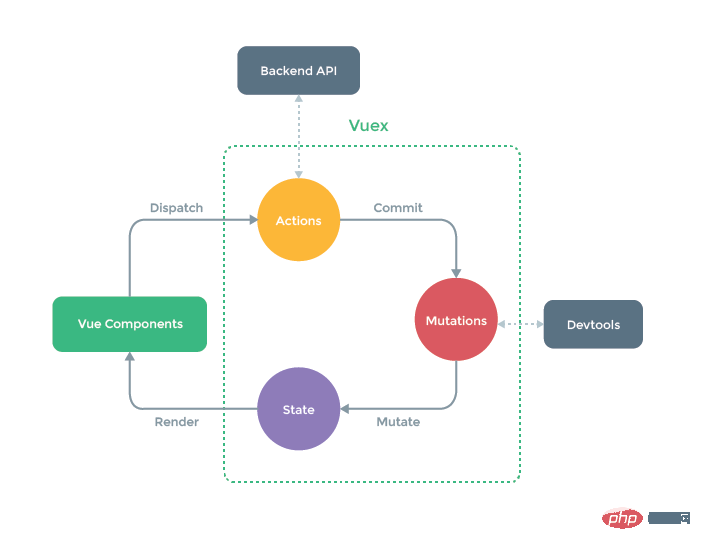
Vuex是做什么的?Vue官方:状态管理工具状态管理是什么?需要在多个组件中共享的状态、且是响应式的、一个变,全都改变。例如一些全局要用的的状态信息:用户登录状态、用户名称、地理位置信息、购物车中商品、等等这时候我们就需要这么一个工具来进行全局的状态管理,Vuex就是这样的一个工具。单页面的状态管理View–>Actions—>State视图层(view)触发操作(action)更改状态(state)响应回视图层(view)vuex(Vue3.
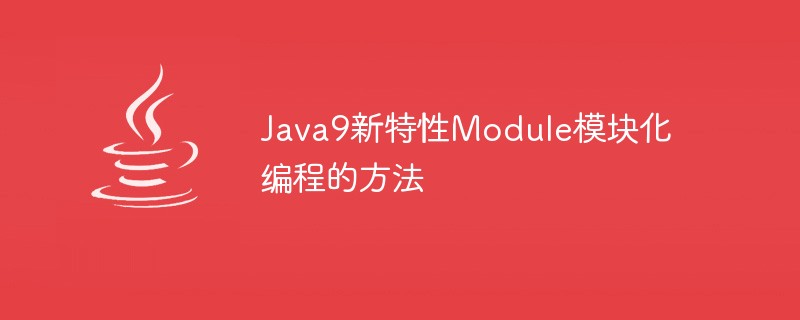
在Java9版本中Java语言引入了一个非常重要的概念:模块(module)。如果对javascript代码模块化管理比较熟悉的小伙伴,看到Java9的模块化管理,应该有似曾相识的感觉。一、什么是Javamodule?与Java中的package有些类似,module引入了Java代码分组的另一个级别。每个这样的分组(module)都包含许多子package包。通过在一个模块的源代码文件package的根部,添加文件module-info.java来声明该文件夹及其子文件夹为一个模块。该文件语法
![在Vue应用中使用vuex时出现“Error: [vuex] unknown action type: xxx”怎么解决?](https://img.php.cn/upload/article/000/887/227/168766615217161.jpg)
在Vue.js项目中,vuex是一个非常有用的状态管理工具。它可以帮助我们在多个组件之间共享状态,并提供了一种可靠的方式来管理状态的变化。但在使用vuex时,有时会遇到“Error:[vuex]unknownactiontype:xxx”的错误。这篇文章将介绍该错误的原因及解决方法。1.错误原因在使用vuex时,我们需要定义一些actions和mu
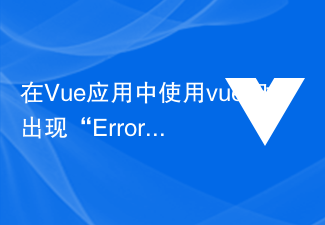
在Vue应用的开发过程中,使用vuex来管理应用状态是非常常见的做法。然而,在使用vuex的过程中,有时我们可能会遇到这样的错误提示:“Error:'xxx'hasalreadybeendeclaredasadataproperty.”这个错误提示看起来很莫名其妙,但其实是由于在Vue组件中,使用了重复的变量名来定义data属性和vuex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
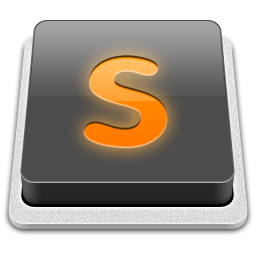
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
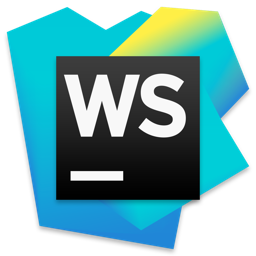
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
