In es6, the three dots "..." refer to the "expansion operator", which can expand array expressions or strings at the syntax level during function calls or array construction; it can also be used When constructing a literal object, expand the object expression in a "key-value" manner.
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
What do the three dots in es6 mean?
The real name of the three dots (...
) is the expansion operation Symbol is a newly added content in ES6. It can expand array expressions or strings at the syntactic level during function call/array construction; it can also expand object expressions according to key- when constructing literal objects. Expand value
Literals generally refer to [1,2,3] or {name:'chuichui'}, a simple construction method, three points of multi-layer nested arrays and objects There's nothing you can do
To put it bluntly, it means taking off your clothes, whether it's braces ([]) or curly braces ({}), it doesn't matter, take them all off!
// 数组 var number = [1,2,3,4,5,6] console.log(...number) //1 2 3 4 5 6 //对象 var man = {name:'chuichui',height:176} console.log({...man}) / {name:'chuichui',height:176}
8 ways to use the spread operator
1. Copy array object
Using the expander to copy an array is a commonly used operation in ES6:
const years = [2018, 2019, 2020, 2021]; const copyYears = [...years]; console.log(copyYears); // [ 2018, 2019, 2020, 2021 ]
The expansion operator copies the array, only the first layer is a deep copy, that is, used for one-dimensional arrays Extension operator copy is a deep copy, look at the following code:
const miniCalendar = [2021, [1, 2, 3, 4, 5, 6, 7], 1]; const copyArray = [...miniCalendar]; console.log(copyArray); // [ 2021, [ 1, 2, 3, 4, 5, 6, 7 ], 1 ] copyArray[1][0] = 0; copyArray[1].push(8); copyArray[2] = 2; console.log(copyArray); // [ 2021, [ 0, 2, 3, 4, 5, 6, 7, 8 ], 2 ] console.log(miniCalendar); // [ 2021, [ 0, 2, 3, 4, 5, 6, 7, 8 ], 1 ]
Put the printed results together for clearer comparison, as follows:
Variable description | Result | Operation |
---|---|---|
##copyArray
|
[ 2021, [ 1, 2, 3, 4, 5, 6, 7 ], 1 ]
| Copy ArrayminiCalendar
|
copyArray
|
[ 2021, [ 0, 2, 3, 4, 5, 6, 7, 8 ], 2 ]
| 1. Reassign the first element of the second element of the array to 0; 2. Add an element 8 to the second element of the array; 3. Reassign the third element of the array to 2|
miniCalendar
|
[ 2021, [ 0, 2, 3, 4, 5, 6, 7, 8 ], 1 ]
| From the results, the second element of the array is an array, which is larger than 1 dimension. Changes in the elements inside will cause the value of the original variable to change accordingly
const time = { year: 2021, month: 7, day: { value: 1, }, }; const copyTime = { ...time }; console.log(copyTime); // { year: 2021, month: 7, day: { value: 1 } }The expansion operator copy object will only perform deep copy on one layer. The following code is based on the above code:
copyTime.day.value = 2; copyTime.month = 6; console.log(copyTime); // { year: 2021, month: 6, day: { value: 2 } } console.log(time); // { year: 2021, month: 7, day: { value: 2 } }From the printed result, The spread operator only makes a deep copy of the first level of the object.
Strictly speaking, the spread operator does not perform deep copy
2. Merge operation
Let’s first look at the merging of arrays, as follows:const halfMonths1 = [1, 2, 3, 4, 5, 6]; const halfMonths2 = [7, 8, 9, 10, 11, 12]; const allMonths = [...halfMonths1, ...halfMonths2]; console.log(allMonths); // [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 ]Merge objects. When merging objects, if a key already exists, it will be replaced by the last object with the same key.
const time1 = { month: 7, day: { value: 1, }, }; const time2 = { year: 2021, month: 8, day: { value: 10, }, }; const time = { ...time1, ...time2 }; console.log(time); // { month: 8, day: { value: 10 }, year: 2021 }
3. Parameter passing
const sum = (num1, num2) => num1 + num2; console.log(sum(...[6, 7])); // 13 console.log(sum(...[6, 7, 8])); // 13From the above code, we can see how many parameters the function defines and the value passed in by the expansion operator Just how many. is used together with the
math function, as follows:
const arrayNumbers = [1, 5, 9, 3, 5, 7, 10]; const min = Math.min(...arrayNumbers); const max = Math.max(...arrayNumbers); console.log(min); // 1 console.log(max); // 10
4. Array deduplication
Use withSet to eliminate duplicates from an array, as follows:
const arrayNumbers = [1, 5, 9, 3, 5, 7, 10, 4, 5, 2, 5]; const newNumbers = [...new Set(arrayNumbers)]; console.log(newNumbers); // [ 1, 5, 9, 3, 7, 10, 4, 2 ]
5. String to character array
String is also an iterable object, so you can also use the spread operator
... to convert it into a character array, as follows:
const title = "china"; const charts = [...title]; console.log(charts); // [ 'c', 'h', 'i', 'n', 'a' ]Then you can simply character String interception, as follows:
const title = "china"; const short = [...title]; short.length = 2; console.log(short.join("")); // ch
6. NodeList Convert to array
NodeList
An object is a collection of nodes, usually returned by properties such as
Node.childNodesand methods such as
document.querySelectorAll.
NodeList is similar to an array, but not an array. It does not have all the methods of
Array, such as
find,
map,
filter, etc., but can be iterated using
forEach().
const nodeList = document.querySelectorAll(".row"); const nodeArray = [...nodeList]; console.log(nodeList); console.log(nodeArray);
7. Destructuring variables
Deconstruct the array, as follows:const [currentMonth, ...others] = [7, 8, 9, 10, 11, 12]; console.log(currentMonth); // 7 console.log(others); // [ 8, 9, 10, 11, 12 ]Deconstruct the object, as follows:
const userInfo = { name: "Crayon", province: "Guangdong", city: "Shenzhen" }; const { name, ...location } = userInfo; console.log(name); // Crayon console.log(location); // { province: 'Guangdong', city: 'Shenzhen' }
8. Print log
When printing iterable objects, you need to use expansion characters for each item, as follows:const years = [2018, 2019, 2020, 2021]; console.log(...years); // 2018 2019 2020 2021
Summary##Extension Operator... makes the code more concise and should be the more popular operator in ES6.
【Related recommendations:
javascript video tutorialThe above is the detailed content of What do three dots mean in es6. For more information, please follow other related articles on the PHP Chinese website!

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
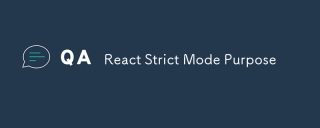
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
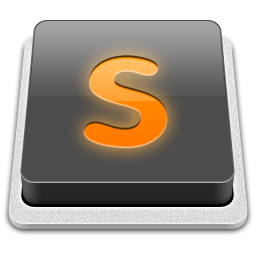
SublimeText3 Mac version
God-level code editing software (SublimeText3)
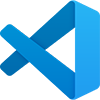
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft