This article brings you relevant knowledge about java, which mainly summarizes and introduces five methods of creating multi-threads, including inheriting the Thread class, implementing the Runnable interface, implementing the Callable interface, Inherit the TimerTask class and start multi-threads through the thread pool. I hope it will be helpful to everyone.
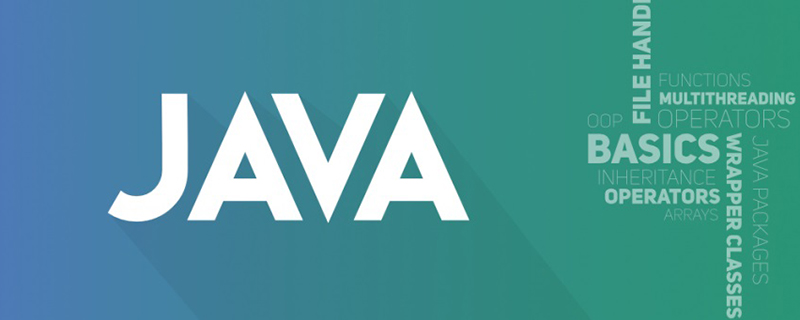
Recommended study: "java Learning Tutorial"
Five ways to create multi-threads in Java
- This is only for communication and learning about Java programming. If you have any questions, please feel free to let me know. Welcome to add and communicate, please indicate when reprinting!
(1) Inherit the Thread class
1. Implementation description
- By inheriting Thread and overriding its run(), the tasks that need to be performed are defined in the run method. The created subclass can execute the thread method by calling the start() method.
- By inheriting the thread class implemented by Thread, instance variables of the thread class cannot be shared between multiple threads. Different Thread objects need to be created, and resources are naturally not shared.
2. Specific steps
1) Define the UserThread class and inherit the Thread class
2) Override the run() method
3) Create a UserThread object
4) Call the start() method
3. Code implementation
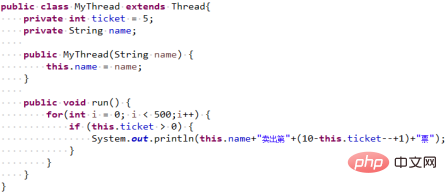
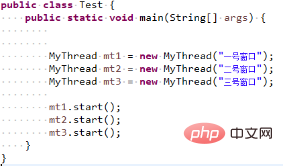
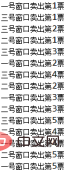
4. Note
- Data resources are not shared, and multiple threads complete their own tasks. For example, if three ticket windows sell tickets at the same time, and each sells its own ticket, there will be a problem of three ticket windows selling the same ticket.
(2) Implement Runnable interface
1. Implementation description
- You need to first define a class to implement the Runnable interface and override the run() method of the interface. This run method is the thread execution body. Then create an object of the Runnable implementation class as the parameter target for creating the Thread object. This Thread object is the real thread object.
- Using the thread class that implements the Runnable interface to create objects can realize resource sharing between threads.
2. Specific steps
1) Define a UserRun class and implement the Runnble interface
2) Override the run() method
3) Create an object of the UserRun class
4) Create an object of the Thread class, The object of the UserRun class is used as a parameter of the Thread class constructor
5) Start the thread
3. Code implementation
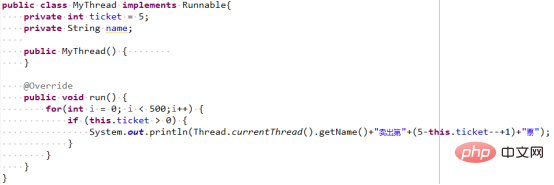
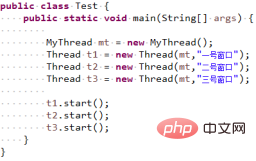

4. Note
- Data resource sharing, multiple threads complete a task together (multiple threads share the resources for creating thread objects). For example, three ticket windows (three threads) sell tickets at the same time (ticket in the MyThread class), and the three threads use resources together.
(3) Implementing the Callable interface
1. Implementation description
- The Callable interface is like an upgraded version of the Runable interface. The call() method it provides will serve as the execution body of the thread and allows a return value.
- Callable objects cannot be directly used as the target of Thread objects because the Callable interface is a new interface in Java5 and is not a sub-interface of the Runnable interface.
- The solution to this problem is to introduce the Future interface. This interface can accept the return value of call(). The RunnableFuture interface is a sub-interface of the Future interface and the Runnable interface and can be used as the target of the Thread object.
2. Specific steps
1) Define the class UserCallable and implement the Callable interface
2) Override the call() method
3) Create a UserCallable object
4) Create a subclass of FutureTask of the RunnableFuture interface Object, the parameter of the constructor is the object of UserCallable
5) Create an object of Thread class, the parameter of the constructor is the object of FutureTask
6) Start the thread
3. Code
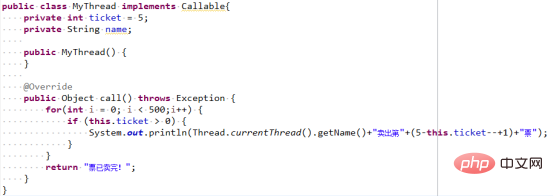
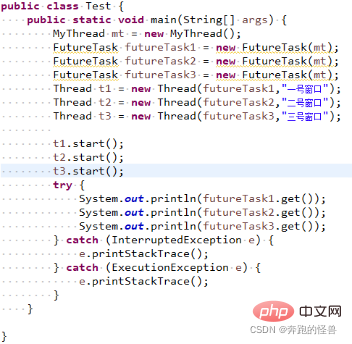
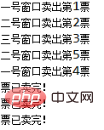
4. Note
- Data resource sharing, multiple threads complete a task together (multiple threads share the resources for creating thread objects). For example, three ticket windows (three threads) sell tickets at the same time (ticket in the MyThread class), and the three threads use resources together. At the same time, there will be a return value after the thread call is completed.
(4) Inherit the TimerTask class
1. Implementation description
- The timer classes Timer and TimerTask can be used as another way to implement threads.
- Timer is a threading facility used to schedule tasks to be executed later in a background thread. The task can be scheduled to be executed once or repeatedly at regular intervals. It can be regarded as a timer and TimerTask can be scheduled.
- TimerTask is an abstract class that implements the Runnable interface, so it has multi-threading capabilities.
2. Specific steps
1) Define the class UserTimerTask and inherit the abstract class TimerTask
2) Create an object of the UserTask class
3) Create an object of the Timer class and set the execution strategy of the task
3. Code implementation
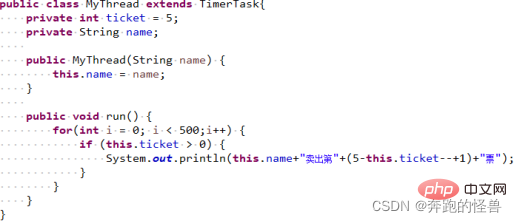
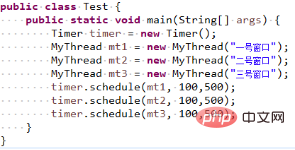
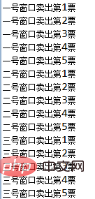
4. Notes
- The thread created by the timer class is more used for the processing of scheduled tasks, and data resources are not shared between threads, and multiple threads complete their own tasks respectively.
(5) Start multi-threads through the thread pool
1. Implementation description
- Thread pools can be created through the Executors tool class.
- Improve the system response speed. When a task arrives, by reusing the existing thread, it can be executed immediately without waiting for the creation of a new thread.
- Reduce system resource consumption and reduce the consumption caused by thread creation and destruction by reusing existing threads.
- Conveniently control the number of concurrent threads. Because if threads are created without limit, it may cause OOM due to excessive memory usage, and may cause excessive CPU switching.
2. Implementation method
1) FixThreadPool(int n) fixed-size thread pool
(1) Specific steps
① Create a fixed-size thread through Executors.newFixedThreadPool(5) Pool
② Override the run( ) method of the Runnable class and use the thread pool to perform tasks
③ Shutdown( ) closes the thread pool
(2) Code implementation
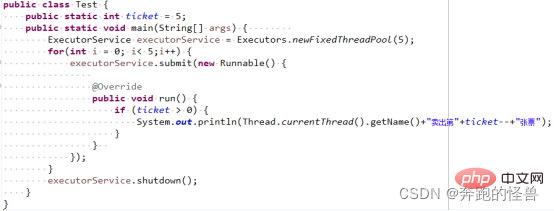
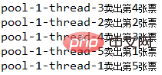
(3) Notes
- Creating a fixed-size thread pool can realize data resource sharing, and multiple threads can complete a task together.
2) SingleThreadExecutor() single-thread pool
(1) Specific steps
① Create a single-thread pool through Executors.newSingleThreadExecutor()
② Repeat Write the run() method of the Runnable class and use the thread pool to perform tasks
③Shutdown() closes the thread pool
(2) Code implementation
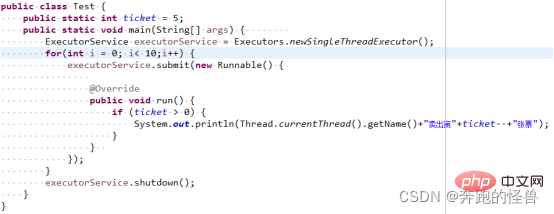
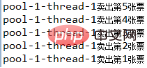
(3) Notes
- The thread pool only creates one thread to perform the task.
3) CachedThreadPool() cache thread pool
(1) Specific steps
① Create as many thread pools as possible through Executors.newCachedThreadPool()
② Rewrite the run( ) method of the Runnable class and use the thread pool to perform tasks
③ Shutdown( ) to close the thread pool
(2) Code implementation
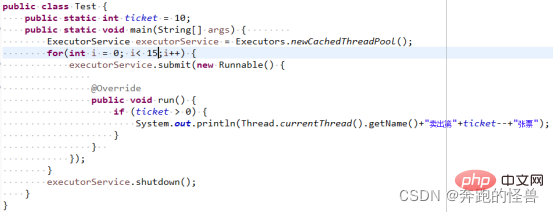
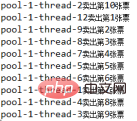
(3) Notes
- This method will create as many threads as possible to complete the task. For example, although there are only 10 tickets in the case, the thread pool At least 12 threads are generated.
4) ScheduledThreadPool(int n) scheduled periodic thread pool
(1) Specific steps
① Create a fixed core thread through Executors.newScheduledThreadPool(5) The number of thread pools (minimum number of threads to maintain, threads will not be recycled after creation), threads are executed regularly as planned.
② Rewrite the run( ) method of the Runnable class and use the thread pool to perform tasks
③ Shutdown( ) closes the thread pool
(2) Code implementation
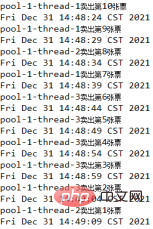
(3) Notes
- Create a periodic thread pool to support scheduled and periodic execution of tasks (the first time parameter is the execution delay time, and the second parameter is the execution interval time).
5) Extension of WorkStealingPool() new thread pool class ForkJoinPool
(1) Specific steps
① Create a thread pool through Executors.newWorkStealingPool()
② Rewrite the run() method of the Runnable class, call the Runnable class object through the Thread class object, and use the thread pool to perform the task
③ Sleep() allows the main thread to wait for the child thread to complete execution, or you can use a counter
④Shutdown( ) closes the thread pool
(2) Code implementation
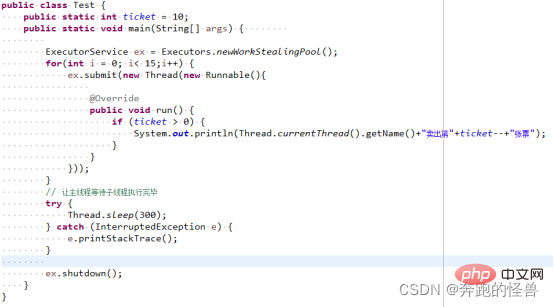
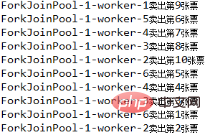
(3) Notes
- Because each thread has its own task queue, because there are many tasks, it may cause CPU load imbalance. This method can effectively take advantage of the advantages of multi-core CPUs. Threads with fewer tasks can "steal" tasks from threads with more tasks, thereby balancing the execution of tasks on each CPU.
Recommended study: "java tutorial"
The above is the detailed content of Five ways! Summary of creating multi-threads in Java. For more information, please follow other related articles on the PHP Chinese website!