This article brings you relevant knowledge about javascript variable promotion. What is variable promotion and why there is variable promotion? Let’s take a look at it together. I hope it will be helpful to everyone.
Related recommendations: javascript learning tutorial
Let’s look at the code first. What do you think the following code outputs? what's the result?
showName() console.log(myname) var myname = '极客时间' function showName() { console.log('函数showName被执行'); }
Programmers who have used JavaScript development should know that JavaScript is executed in order. If we understand it according to this logic, then:
- When executing line 1, since the function showName has not been defined yet, the execution should report an error;
- Execute the same line In line 2, since the variable myname is not defined, an error will also be reported.
However, the actual execution result is not like this, as shown below:
The first line outputs "function showName is executed", and the second line outputs "undefined", which is the same as The sequential execution as imagined earlier is a bit different!
Through the above execution results, you should already know that functions or variables can be used before they are defined. So if you use undefined variables or functions, can the JavaScript code continue to execute? In order to verify this, we can delete the definition of the variable myname in line 3, as follows:
showName() console.log(myname) function showName() { console.log('函数showName被执行'); }
Then when this code is executed again, the JavaScript engine will report an error, and the result is as follows:
Judging from the execution results of the above two pieces of code, we can draw the following three conclusions:
- During the execution process, if undeclared variables are used, JavaScript execution will report an error .
- Using a variable before it is defined will not cause an error, but the value of the variable will be undefined, not the value when it was defined.
- Use it before a function definition, no error will occur, and the function will execute correctly.
The first conclusion is easy to understand, because the variable is not defined, so when the JavaScript code is executed, the variable cannot be found, so JavaScript will throw an error.
But for the second and third conclusions, it is quite puzzling:
- Why can variables and functions be used before they are defined? This seems to indicate that the JavaScript code is not executed line by line.
- In the same way, why are the processing results of variables and functions different? For example, in the above execution result, the showName function used in advance can print out the complete result, but the value of the myname variable used in advance is undefined, not the value of "geek time" used in the definition.
Variable Hoisting (Hoisting)
To explain these two issues, you need to first understand what variable hoisting is.
But before introducing variable promotion, let’s first look at the following code to see what declaration and assignment in JavaScript are.
var myname = '极客时间'
You can think of this code as consisting of two lines of code:
var myname //声明部分 myname = '极客时间' //赋值部分
As shown below:
The above is the declaration and Assignment, then let’s take a look at the declaration and assignment of functions, combined with the following code:
function foo(){ console.log('foo') } var bar = function(){ console.log('bar') }
The first function foo is a complete function declaration, which means that there is no assignment operation involved; The two functions first declare the variable bar, and then assign function(){console.log('bar')} to bar. For an intuitive understanding, you can refer to the following picture:
Okay, now that we understand the declaration and assignment operations, we can now talk about what variable promotion is.
The so-called variable promotion refers to the "behavior" in which the JavaScript engine promotes the declaration part of variables and the declaration part of functions to the beginning of the code during the execution of JavaScript code. After the variable is promoted, a default value will be set for the variable. This default value is the familiar undefined.
Let’s simulate the implementation:
/* * 变量提升部分 */// 把变量 myname提升到开头,// 同时给myname赋值为undefinedvar myname = undefined// 把函数showName提升到开头function showName() { console.log('showName被调用');}/* * 可执行代码部分 */showName()console.log(myname)// 去掉var声明部分,保留赋值语句myname = '极客时间'
In order to simulate the effect of variable promotion, we have made the following adjustments to the code, as shown below:
As can be seen from the picture, two main adjustments have been made to the original code:
- 第一处是把声明的部分都提升到了代码开头,如变量 myname 和函数 showName,并给变量设置默认值 undefined;
- 第二处是移除原本声明的变量和函数,如var myname = '极客时间’的语句,移除了 var 声明,整个移除 showName 的函数声明。
通过这两步,就可以实现变量提升的效果。你也可以执行这段模拟变量提升的代码,其输出结果和第一段代码应该是完全一样的。
通过这段模拟的变量提升代码,相信你已经明白了可以在定义之前使用变量或者函数的原因——函数和变量在执行之前都提升到了代码开头。
JavaScript 代码的执行流程
从概念的字面意义上来看,“变量提升”意味着变量和函数的声明会在物理层面移动到代码的最前面,正如我们所模拟的那样。但,这并不准确。实际上变量和函数声明在代码里的位置是不会改变的,而且是在编译阶段被 JavaScript 引擎放入内存中。对,你没听错,一段 JavaScript 代码在执行之前需要被 JavaScript 引擎编译,编译完成之后,才会进入执行阶段。大致流程你可以参考下图:
1. 编译阶段
那么编译阶段和变量提升存在什么关系呢?
为了搞清楚这个问题,我们还是回过头来看上面那段模拟变量提升的代码,为了方便介绍,可以把这段代码分成两部分。
第一部分:变量提升部分的代码。
var myname = undefined function showName() { console.log('函数showName被执行'); }
第二部分:执行部分的代码。
showName() console.log(myname) myname = '极客时间'
下面我们就可以把 JavaScript 的执行流程细化,如下图所示:
从上图可以看出,输入一段代码,经过编译后,会生成两部分内容:执行上下文(Execution context)和可执行代码。
执行上下文是 JavaScript 执行一段代码时的运行环境,比如调用一个函数,就会进入这个函数的执行上下文,确定该函数在执行期间用到的诸如 this、变量、对象以及函数等。
关于执行上下文的细节,我会在下一篇文章《08 | 调用栈:为什么 JavaScript 代码会出现栈溢出?》做详细介绍,现在你只需要知道,在执行上下文中存在一个变量环境的对象(Viriable Environment),该对象中保存了变量提升的内容,比如上面代码中的变量 myname 和函数 showName,都保存在该对象中。
你可以简单地把变量环境对象看成是如下结构:
VariableEnvironment: myname -> undefined, showName ->function : {console.log(myname)
了解完变量环境对象的结构后,接下来,我们再结合下面这段代码来分析下是如何生成变量环境对象的。
showName() console.log(myname) var myname = '极客时间' function showName() { console.log('函数showName被执行'); }
我们可以一行一行来分析上述代码:
- 第 1 行和第 2 行,由于这两行代码不是声明操作,所以 JavaScript 引擎不会做任何处理;
- 第 3 行,由于这行是经过 var 声明的,因此 JavaScript 引擎将在环境对象中创建一个名为 myname 的属性,并使用 undefined 对其初始化;
- 第 4 行,JavaScript 引擎发现了一个通过 function 定义的函数,所以它将函数定义存储到堆 (HEAP)中,并在环境对象中创建一个 showName 的属性,然后将该属性值指向堆中函数的位置(不了解堆也没关系,JavaScript 的执行堆和执行栈我会在后续文章中介绍)。
这样就生成了变量环境对象。接下来 JavaScript 引擎会把声明以外的代码编译为字节码,至于字节码的细节,我也会在后面文章中做详细介绍,你可以类比如下的模拟代码:
showName() console.log(myname) myname = '极客时间'
好了,现在有了执行上下文和可执行代码了,那么接下来就到了执行阶段了。
2. 执行阶段
JavaScript 引擎开始执行“可执行代码”,按照顺序一行一行地执行。下面我们就来一行一行分析下这个执行过程:
- 当执行到 showName 函数时,JavaScript 引擎便开始在变量环境对象中查找该函数,由于变量环境对象中存在该函数的引用,所以 JavaScript 引擎便开始执行该函数,并输出“函数 showName 被执行”结果。
- 接下来打印“myname”信息,JavaScript 引擎继续在变量环境对象中查找该对象,由于变量环境存在 myname 变量,并且其值为 undefined,所以这时候就输出 undefined。
- 接下来执行第 3 行,把“极客时间”赋给 myname 变量,赋值后变量环境中的 myname 属性值改变为“极客时间”,变量环境如下所示:
VariableEnvironment: myname -> "极客时间", showName ->function : {console.log(myname)
好了,以上就是一段代码的编译和执行流程 。
代码中出现相同的变量或者函数怎么办?
现在你已经知道了,在执行一段 JavaScript 代码之前,会编译代码,并将代码中的函数和变量保存到执行上下文的变量环境中,那么如果代码中出现了重名的函数或者变量,JavaScript 引擎会如何处理?
我们先看下面这样一段代码:
function showName() { console.log('极客邦'); } showName(); function showName() { console.log('极客时间'); } showName();
在上面代码中,我们先定义了一个 showName 的函数,该函数打印出来“极客邦”;然后调用 showName,并定义了一个 showName 函数,这个 showName 函数打印出来的是“极客时间”;最后接着继续调用 showName。那么你能分析出来这两次调用打印出来的值是什么吗?
我们来分析下其完整执行流程:
- 首先是编译阶段。遇到了第一个 showName 函数,会将该函数体存放到变量环境中。接下来是第二个 showName 函数,继续存放至变量环境中,但是变量环境中已经存在一个 showName 函数了,此时,第二个 showName 函数会将第一个 showName 函数覆盖掉。这样变量环境中就只存在第二个 showName 函数了。
- 接下来是执行阶段。先执行第一个 showName 函数,但由于是从变量环境中查找 showName 函数,而变量环境中只保存了第二个 showName 函数,所以最终调用的是第二个函数,打印的内容是“极客时间”。第二次执行 showName 函数也是走同样的流程,所以输出的结果也是“极客时间”。
综上所述,一段代码如果定义了两个相同名字的函数,那么最终生效的是最后一个函数。
总结
好了,今天就到这里,下面我来简单总结下今天的主要内容:
- JavaScript 代码执行过程中,需要先做变量提升,而之所以需要实现变量提升,是因为 JavaScript 代码在执行之前需要先编译。
- 在编译阶段,变量和函数会被存放到变量环境中,变量的默认值会被设置为 undefined;在代码执行阶段,JavaScript 引擎会从变量环境中去查找自定义的变量和函数。
- 如果在编译阶段,存在两个相同的函数,那么最终存放在变量环境中的是最后定义的那个,这是因为后定义的会覆盖掉之前定义的。
相关推荐:javascript学习教程
The above is the detailed content of JavaScript execution mechanism - variable promotion (detailed examples). For more information, please follow other related articles on the PHP Chinese website!
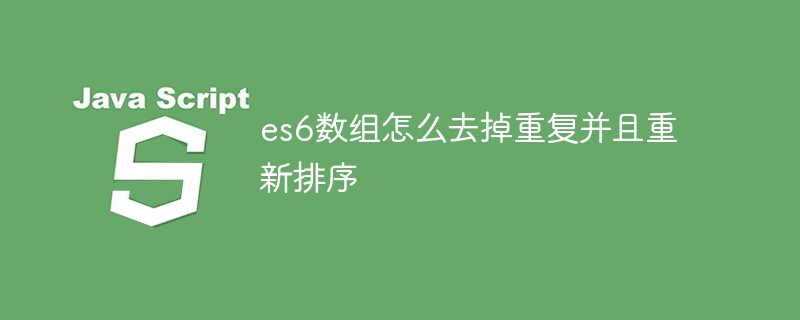
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
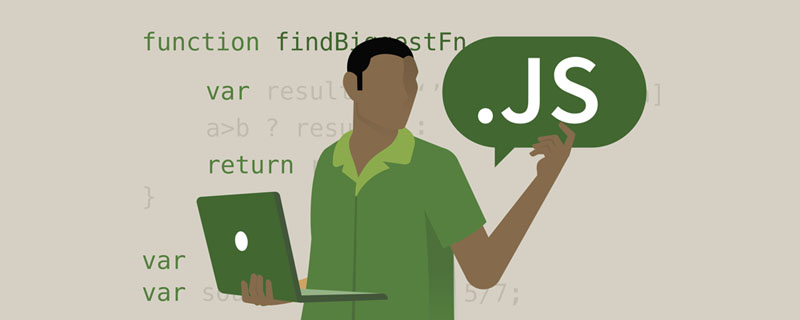
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
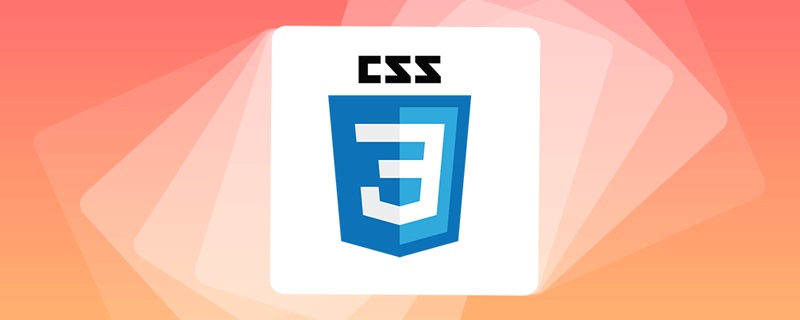
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
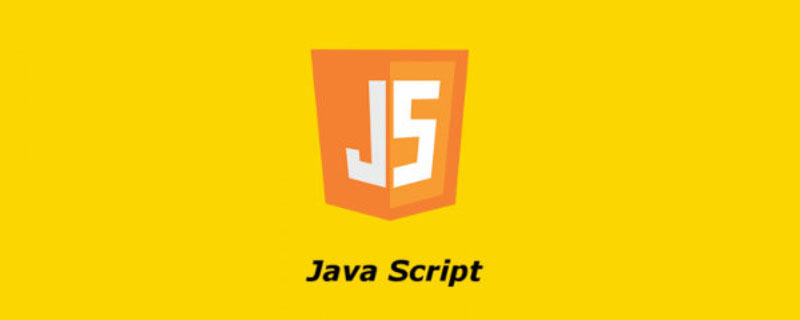
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
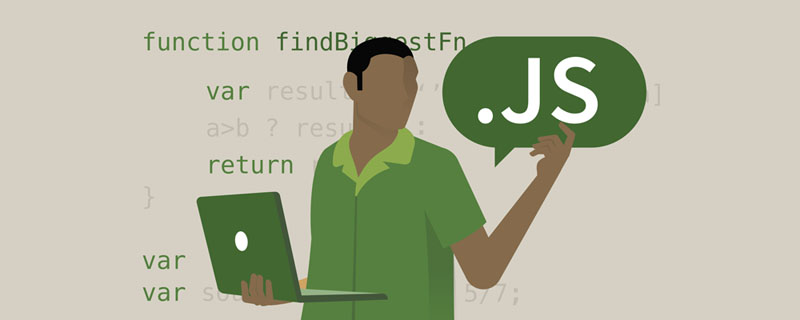
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
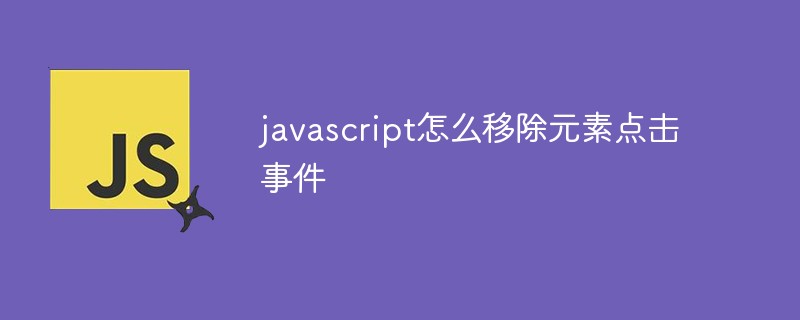
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
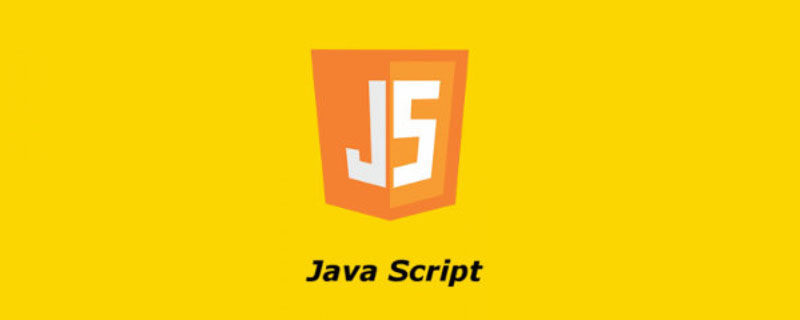
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
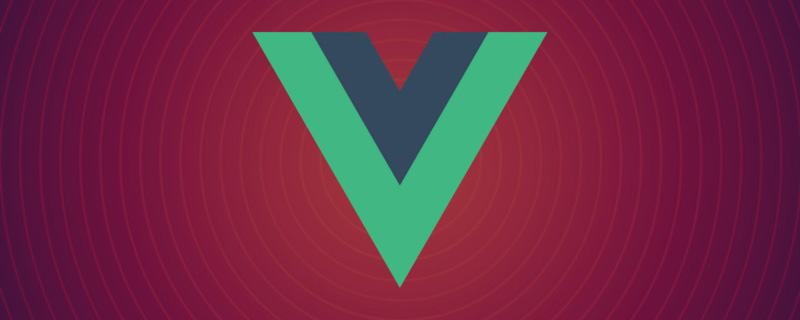
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
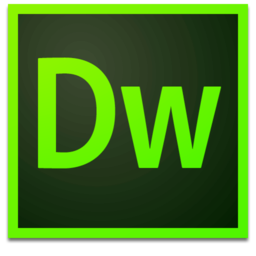
Dreamweaver Mac version
Visual web development tools
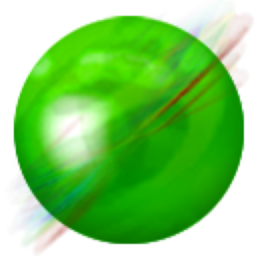
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
