What are the three methods of defining a class in javascript
Three ways to define a class in JavaScript: 1. Use the constructor to define the class, the syntax is "function name(){this.name=value;}"; 2. Use the "Object.create()" method Define the class; 3. Use the "createNew()" method to define the class.
The operating environment of this tutorial: Windows 10 system, JavaScript version 1.8.5, Dell G3 computer.
What are the three methods of defining a class in javascript
In object-oriented programming, a class is a template of an object and defines the same group of objects (also called "instances") ) common properties and methods.
The JavaScript language does not support "classes", but you can use some workarounds to simulate "classes".
1. Constructor method
This is a classic method and a must-teach method in textbooks. It uses a constructor to simulate a "class" and uses the this keyword internally to refer to the instance object.
function Cat(){ this.name = "大毛"; }
When generating an instance, use the new keyword.
var cat1 = new Cat(); alert(cat1.name); //大毛
The attributes and methods of the class can also be defined on the prototype object of the constructor.
Cat.prototype.makeSound = function(){ alert("喵喵喵"); }
For a detailed introduction to this method, please see the series of articles "JavaScript Object-Oriented Programming", I won’t go into details here. Its main disadvantage is that it is complicated to teach, uses this and prototype, and is very laborious to write and read.
2. Object.create() method
In order to solve the shortcomings of the "constructor method" and generate objects more conveniently, JavaScript The fifth edition of the international standard ECMAScript (the third edition is currently popular) proposes a new method Object.create().
Using this method, "class" is an object, not a function.
var Cat = { name: "大毛", makeSound: function(){ alert("喵喵喵"); } };
Then, use Object.create() to generate an instance directly, without using new.
var cat1 = Object.create(Cat); alert(cat1.name); //大毛 cat1.makeSound(); //喵喵喵
Currently, the latest versions of all major browsers (including IE9) have deployed this method. If you encounter an old browser, you can use the following code to deploy it yourself.
if(!Object.create){ Object.create = function(o){ function F(){}; F.prototype = o; return new F(); } }
This method is simpler than the "constructor method", but it cannot implement private properties and private methods, nor can it share data between instance objects, and the simulation of "classes" is not comprehensive enough.
3. Minimalist Method
Dutch programmer Gabor de Mooij proposed a new method that is better than Object.create() method, which it calls the "minimalist approach". This is also the method I recommend.
3.1 Encapsulation
This method does not apply to this and prototype, and the code is very simple to deploy. This is probably why it is called the "minimalist method".
First of all, it also uses an object to simulate a "class". In this class, define a constructor caeateNew() to generate instances.
var Cat = { createNew: function(){ //some code here } };
Then, in carateNew(), define an instance object and use this instance object as the return value.
var Cat = { createNew: function(){ var cat = {}; cat.name = "大毛"; car.makeSound = function(){ alert("喵喵喵"); }; } };
When using it, call the createNew() method to get the instance object.
var cat1 = Cat.createNew(); cat1.makeSound(); //喵喵喵
The advantage of this method is that it is easy to understand, has a clear and elegant structure, and conforms to the traditional "object-oriented programming" construct, so the following features can be easily deployed.
Related recommendations: javascript learning tutorial
The above is the detailed content of What are the three methods of defining a class in javascript. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us

Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as. Data flow management: React's data flow is one-way, passed from the parent component to the child component, ensuring that the data flow is controllable, such as App components passing name to Greeting. Basic usage example: Use map function to render a list, you need to add a key attribute, such as rendering a fruit list. Advanced usage example: Use the useState hook to manage state and implement dynamics

React is the preferred tool for building single-page applications (SPAs) because it provides efficient and flexible ways to build user interfaces. 1) Component development: Split complex UI into independent and reusable parts to improve maintainability and reusability. 2) Virtual DOM: Optimize rendering performance by comparing the differences between virtual DOM and actual DOM. 3) State management: manage data flow through state and attributes to ensure data consistency and predictability.

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
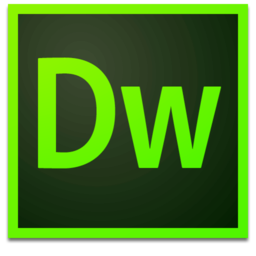
Dreamweaver Mac version
Visual web development tools
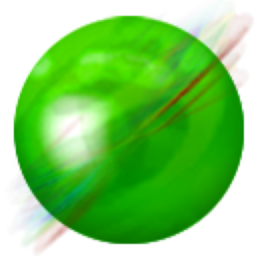
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
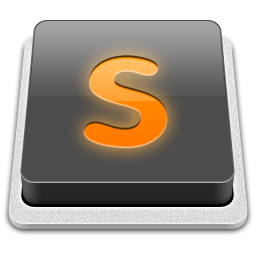
SublimeText3 Mac version
God-level code editing software (SublimeText3)