This article will give you an in-depth understanding of Buffer (buffer) in Node.js. It will introduce various ways to create the Buffer class, methods of writing to the buffer, etc. I hope it will be useful to everyone. Helped!
#The JavaScript language itself only has string data types, not binary data types.
But when processing streams like TCP or file streams, binary data must be used. Therefore, in Node.js, a Buffer class is defined, which is used to create a buffer area specifically for storing binary data.
In Node.js, the Buffer class is a core library released with the Node kernel. The Buffer library brings a method of storing raw data to Node.js, allowing Node.js to process binary data. Whenever you need to process data moved during I/O operations in Node.js, it is possible to use the Buffer library .
The original data is stored in an instance of the Buffer class.
A Buffer is similar to an integer array, but it corresponds to a piece of original memory outside the V8 heap memory.
Creating the Buffer class
The Node Buffer class can be created in a variety of ways.
Method 1
Create a Buffer instance with a length of 10 bytes:
var buf = new Buffer(10);
Method 2
Create a Buffer instance from the given array:
var buf = new Buffer([10, 20, 30, 40, 50]);
Method 3
Create a Buffer instance from a string:
var buf = new Buffer("bianchengsanmei", "utf-8");
utf-8 is the default encoding, and it also supports the following encodings: "ascii", "utf8", "utf16le", "ucs2", "base64" and "hex".
Write buffer
Syntax
The syntax for writing Node buffer is as follows:
buf.write(string[, offset[, length]][, encoding])
Parameters
The parameters are described as follows:
- string - The string written to the buffer.
- offset - The index value at which the buffer starts to be written, default is 0.
- length - Number of bytes written, defaults to buffer.length
- encoding - Encoding used. Defaults to 'utf8' .
Return value
Returns the actual written size. If there is insufficient buffer space, only part of the string will be written.
Example
buf = new Buffer(256); len = buf.write("bi"); len = buf.write("bianchengsanmei"); console.log("写入字节数 : "+ len);
Execute the above code, the output result is:
$node main.js 写入字节数 : 15
Read data from the buffer
Syntax
The syntax for reading Node buffer data is as follows:
buf.toString([encoding[,start[,end]]])
Parameters
The parameters are described as follows:
encoding - the encoding to use. Defaults to 'utf8' .
start - Specifies the index position to start reading, default is 0.
end - End position, defaults to the end of the buffer.
Return Value
Decode the buffer data and return a string using the specified encoding.
Example
buf = new Buffer(26); for (var i = 0 ; i < 26 ; i++) { buf[i] = i + 97; } console.log( buf.toString('ascii')); // 输出: abcdefghijklmnopqrstuvwxyz console.log( buf.toString('ascii',0,5)); // 输出: abcde console.log( buf.toString('utf8',0,5)); // 输出: abcde console.log( buf.toString(undefined,0,5)); // 使用 'utf8' 编码, 并输出: abcde
Execute the above code, the output result is:
$ node main.js abcdefghijklmnopqrstuvwxyz abcde abcde abcde
Convert Buffer to JSON object
Syntax
The function syntax format for converting Node Buffer to JSON object is as follows:
buf.toJSON()
Return value
Returns JSON object.
Example
var buf = new Buffer('bianchengsanmei'); var json = buf.toJSON(buf); console.log(json);
Execute the above code, the output result is:
{ type: 'Buffer', data: [ 98, 105, 97, 110, 99, 104, 101, 110, 103, 115, 97, 110, 109, 101, 105 ] }
Buffer merge
Syntax
The syntax of Node buffer merging is as follows:
Buffer.concat(list[, totalLength])
Parameters
The parameters are described as follows:
- list - an array list of Buffer objects used for merging.
- totalLength - Specifies the total length of the combined Buffer objects.
Return value
Returns a new Buffer object that combines multiple members.
Example
var buffer1 = new Buffer('编程三昧 '); var buffer2 = new Buffer('bi'); var buffer2 = new Buffer('bianchengsanmei'); var buffer3 = Buffer.concat([buffer1,buffer2]); console.log("buffer3 内容: " + buffer3.toString());
Execute the above code, the output result is:
buffer3 内容: 编程三昧 bianchengsanmei
Buffer comparison
Syntax
The function syntax of Node Buffer comparison is as follows. This method was introduced in Node.js v0.12.2 version:
buf.compare(otherBuffer);
Parameters
Parameters The description is as follows:
- otherBuffer - Another Buffer object compared with the buf object.
Return Value
Returns a number indicating that buf is before, after, or the same as otherBuffer.
Example
var buffer1 = new Buffer('ABC'); var buffer2 = new Buffer('ABCD'); var result = buffer1.compare(buffer2); if(result < 0) { console.log(buffer1 + " 在 " + buffer2 + "之前"); }else if(result == 0){ console.log(buffer1 + " 与 " + buffer2 + "相同"); }else { console.log(buffer1 + " 在 " + buffer2 + "之后"); }
Execute the above code, the output result is:
ABC在ABCD之前
Copy buffer
Syntax
Node buffer copy syntax is as follows:
buf.copy(target[, targetStart[, sourceStart[, sourceEnd]]])
Parameters
The parameters are described as follows:
- targetBuffer - The Buffer object to be copied.
- targetStart - number, optional, default: 0
- sourceStart - number, optional, default: 0
- sourceEnd - number, optional, default: buffer.length
Return value
No return value.
Example
var buffer1 = new Buffer('ABC'); // 拷贝一个缓冲区 var buffer2 = new Buffer(3); buffer1.copy(buffer2); console.log("buffer2 content: " + buffer2.toString());
Execute the above code, the output result is:
buffer2 content: ABC
缓冲区裁剪
Node 缓冲区裁剪语法如下所示:
buf.slice([start[, end]])
参数
参数描述如下:
- start - 数字, 可选, 默认: 0
- end - 数字, 可选, 默认: buffer.length
返回值
返回一个新的缓冲区,它和旧缓冲区指向同一块内存,但是从索引 start 到 end 的位置剪切。
实例
var buffer1 = new Buffer('youj'); // 剪切缓冲区 var buffer2 = buffer1.slice(0,2); console.log("buffer2 content: " + buffer2.toString());
执行以上代码,输出结果为:
buffer2 content: yo
缓冲区长度
语法 Node 缓冲区长度计算语法如下所示:
buf.length;
返回值
返回 Buffer 对象所占据的内存长度。
实例
var buffer = new Buffer('bianchengsanmei'); // 缓冲区长度 console.log("buffer length: " + buffer.length);
执行以上代码,输出结果为:
buffer length: 15
~
~本文完,感谢阅读!
更多node相关知识,请访问:nodejs 教程!
The above is the detailed content of A closer look at Buffers in Node.js. For more information, please follow other related articles on the PHP Chinese website!
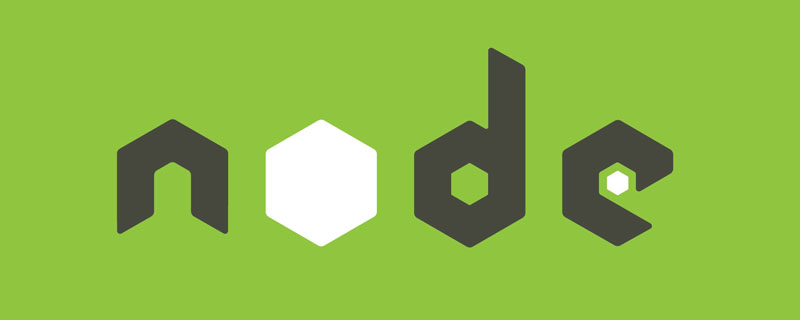
Vercel是什么?本篇文章带大家了解一下Vercel,并介绍一下在Vercel中部署 Node 服务的方法,希望对大家有所帮助!

gm是基于node.js的图片处理插件,它封装了图片处理工具GraphicsMagick(GM)和ImageMagick(IM),可使用spawn的方式调用。gm插件不是node默认安装的,需执行“npm install gm -S”进行安装才可使用。
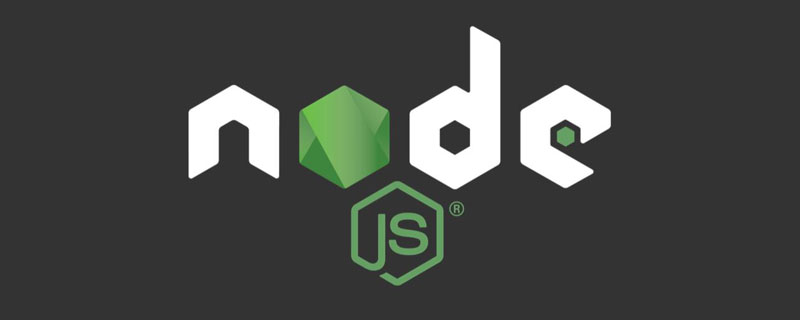
如何用pkg打包nodejs可执行文件?下面本篇文章给大家介绍一下使用pkg将Node.js项目打包为可执行文件的方法,希望对大家有所帮助!
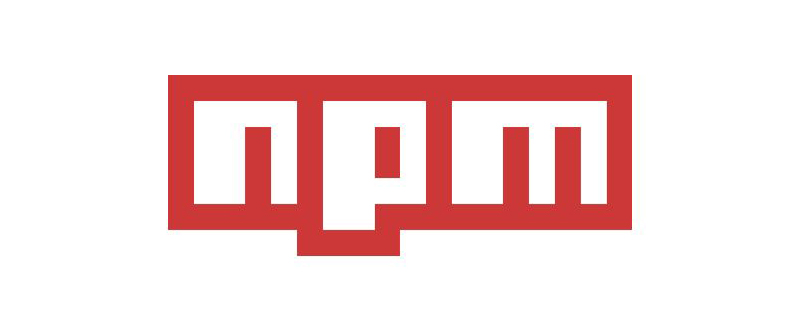
本篇文章带大家详解package.json和package-lock.json文件,希望对大家有所帮助!
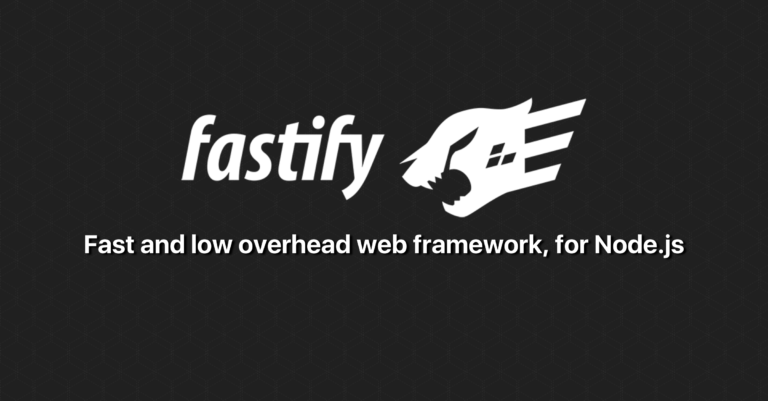
本篇文章给大家分享一个Nodejs web框架:Fastify,简单介绍一下Fastify支持的特性、Fastify支持的插件以及Fastify的使用方法,希望对大家有所帮助!
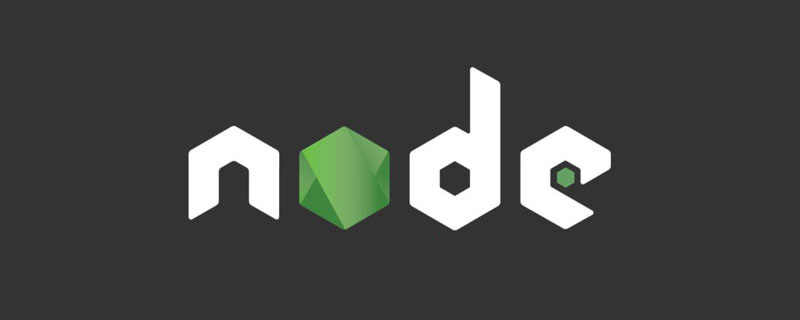
node怎么爬取数据?下面本篇文章给大家分享一个node爬虫实例,聊聊利用node抓取小说章节的方法,希望对大家有所帮助!

本篇文章给大家分享一个Node实战,介绍一下使用Node.js和adb怎么开发一个手机备份小工具,希望对大家有所帮助!
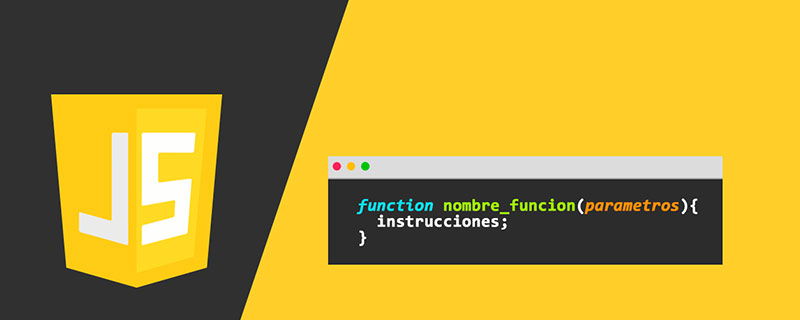
先介绍node.js的安装,再介绍使用node.js构建一个简单的web服务器,最后通过一个简单的示例,演示网页与服务器之间的数据交互的实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
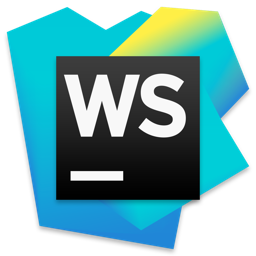
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
