A brief analysis of what is a decorator? How to use decorators in Vue?
What is a decorator? This article will introduce you to decorators and briefly introduce how to use decorators in js and vue. I hope it will be helpful to you!
# I believe that you must have encountered the need for secondary pop-up confirmation during development. Whether you are using the secondary pop-up component of the UI framework or your own encapsulated pop-up component. All of them cannot avoid the problem of a large amount of repeated code when used multiple times. The accumulation of these codes results in poor readability of the project. The code quality of the project has also become very poor. So how do we solve the problem of duplicate pop-up codes? Using Decorators
What are decorators?
Decorator
is a new syntax for ES7
. Decorator
Decorate classes, objects, methods, and properties. Add some additional behavior to it. In layman's terms: it is a secondary packaging of a piece of code.
The use of decorators
The method of use is very simple. We define a function
const decorator = (target, name, descriptor) => { var oldValue = descriptor.value; descriptor.value = function(){ alert('哈哈') return oldValue.apply(this,agruments) } return descriptor } // 然后直接@decorator到函数、类或者对象上即可。
The purpose of the decorator is to reuse the code. Let's take a small example first to see
Using decorators in js
//定义一个装饰器 const log = (target, name, descriptor) => { var oldValue = descriptor.value; descriptor.value = function() { console.log(`Calling ${name} with`, arguments); return oldValue.apply(this, arguments); }; return descriptor; } //计算类 class Calculate { //使用装饰器 @log() function subtraction(a,b){ return a - b } } const operate = new Calculate() operate.subtraction(5,2)
Not using decorators
const log = (func) => { if(typeof(func) !== 'function') { throw new Error(`the param must be a function`); } return (...arguments) => { console.info(`${func.name} invoke with ${arguments.join(',')}`); func(...arguments); } } const subtraction = (a, b) => a + b; const subtractionLog = log(subtraction); subtractionLog(10,3);
In this comparison, you will find that the code after using decorators Readability has become stronger. Decorators don't care about the implementation of your inner code.
Using decorators in vue
If your project is built with vue-cli and the version of vue-cli is greater than 2.5, you can use it without any configuration. If your project also contains eslit, then you need to enable support for decorator-related syntax detection in eslit. [Related recommendations: vue.js video tutorial]
//在 eslintignore中添加或者修改如下代码: parserOptions: { ecmaFeatures:{ // 支持装饰器 legacyDecorators: true } }
After adding this code, eslit will support decorator syntax.
Usually in projects, we often use secondary pop-up boxes for deletion operations:
//decorator.js //假设项目中已经安装了 element-ui import { MessageBox, Message } from 'element-ui' /** * 确认框 * @param {String} title - 标题 * @param {String} content - 内容 * @param {String} confirmButtonText - 确认按钮名称 * @param {Function} callback - 确认按钮名称 * @returns **/ export function confirm(title, content, confirmButtonText = '确定') { return function(target, name, descriptor) { const originValue = descriptor.value descriptor.value = function(...args) { MessageBox.confirm(content, title, { dangerouslyUseHTMLString: true, distinguishCancelAndClose: true, confirmButtonText: confirmButtonText }).then(originValue.bind(this, ...args)).catch(error => { if (error === 'close' || error === 'cancel') { Message.info('用户取消操作')) } else { Message.info(error) } }) } return descriptor } }
The above code confirm method executes a MessageBox
component in element-ui When the user cancels, the Message
component will prompt the user to cancel the operation.
Let’s decorate the test() method with a decorator
import { confirm } from '@/util/decorator' import axios form 'axios' export default { name:'test', data(){ return { delList: '/merchant/storeList/commitStore' } } }, methods:{ @confirm('删除门店','请确认是否删除门店?') test(id){ const {res,data} = axios.post(this.delList,{id}) if(res.rspCd + '' === '00000') this.$message.info('操作成功!') } }
At this time, the user clicks on a store to delete it. The decorator will work. The pop-up is as shown below:
When I click cancel:
tips: The user canceled Operation. The modified test method will not execute .
When we click OK:
The interface is called and the message pops up
Summary
The decorator is used To repackage a piece of code. Add some behavioral operations and attributes to the code. Using decorators can greatly reduce code duplication. Improve code readability.
Finally
If there are any shortcomings in the article, please criticize and point it out.
For more programming-related knowledge, please visit: Introduction to Programming! !
The above is the detailed content of A brief analysis of what is a decorator? How to use decorators in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
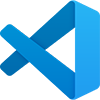
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
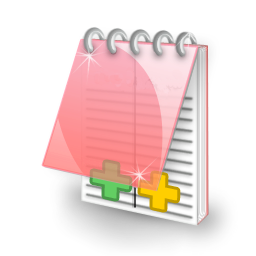
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function