How to determine whether javascript contains a specified string
Judgment method: 1. Use the indexOf() method to obtain the first occurrence position of the specified string value. If the return value is "-1", it is not included; 2. Use the search() method to retrieve the specified string. If the return value is "-1", it is not included; 3. Use the match() method; 4. Use the test() method, etc.
The operating environment of this tutorial: windows7 system, javascript version 1.8.5, Dell G3 computer.
JavaScript determines whether the string contains the specified string
Method 1: indexOf() (recommended)
The indexOf() method returns the position where a specified string value first appears in the string. If the string value to be retrieved does not appear, the method returns -1.
var str ="123"; console.log(str.indexOf("3")!=-1);//true
Method 2: search()
The search() method is used to retrieve the specified substring in the string, or to retrieve it with a regular expression Matching substring, if no matching substring is found, -1 is returned.
var str="123"; console.log(str.search("3")!=-1);//true
Method 3: match()
The match() method can retrieve a specified value within a string, or find one or more regular expressions formula matching.
var str="123"; var reg=RegExp(/3/); if(str.match(reg)){ //包含 }
Method 4: test()
The test() method is used to retrieve the value specified in the string. Returns true and false.
var str="123"; var reg=RegExp(/3/); console.log(reg.test(str));//true
Method 5: exec()
The exec method is used to retrieve matches of regular expressions in a string. Returns an array containing the matching results. If no match is found, the return value is null.
var str="123"; reg=RegExp(/3/); if(reg.exec(str)){ //包含 }
【Related recommendations: javascript learning tutorial】
The above is the detailed content of How to determine whether javascript contains a specified string. For more information, please follow other related articles on the PHP Chinese website!
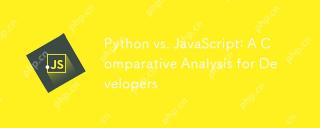
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
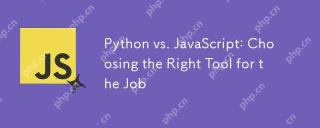
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
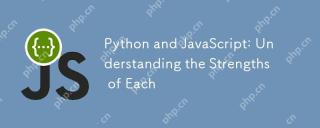
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
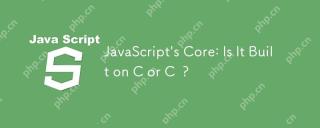
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
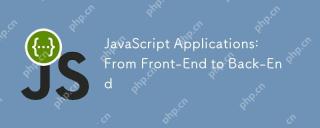
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
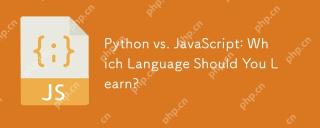
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
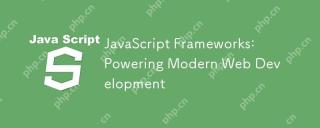
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
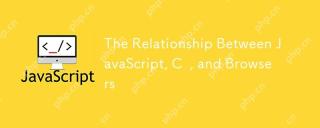
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
