var is a built-in keyword in javascript, used to declare variables and optionally initialize them to a value; the syntax format is "var varname [=value];", the parameter value can be any legal expression, the default value is "undefined".
The operating environment of this tutorial: windows7 system, javascript version 1.8.5, Dell G3 computer.
Declaring (Creating) JavaScript Variables
Creating a variable in JavaScript is called "declaring" a variable.
You can declare JavaScript variables through the var
keyword; the var statement declares a variable and optionally initializes it to a value:
var varname1 [= value1] [, varname2 [= value2] ... [, varnameN [= valueN]]];
-
varname: variable name. Variable names can be defined as any legal identifier.
value: The initialization value of the variable. The value can be any legal expression. The default value is undefined.
Description:
Variable declarations, wherever they occur, are processed before any code is executed. The scope of a variable declared with var is its current execution context, which can be a nested function, or globally for variables declared outside any function. If you redeclare a JavaScript variable, it will not lose its value.
When assigning a value to an undeclared variable, after the assignment is performed, the variable will be implicitly created as a global variable (it will become a property of the global object).
Example:
1. Declare and initialize two variables:
var a = 0, b = 0;
Assign the two variables to string values:
var a = "A"; var b = a; // 等效于: var a, b = a = "A";
Pay attention to the order:
var x = y, y = 'A'; console.log(x + y); // undefinedA
Here, x and y are created before the code is executed, and the assignment operation occurs after creation. When "x = y" is executed, y already exists, so no ReferenceError is thrown, and its value is 'undefined'. So x is assigned the value undefined. Then, y is assigned 'A'. Therefore, after executing the first line, x === undefined && y === 'A'
resulted in this result.
[Related recommendations: javascript learning tutorial]
The difference between declared and undeclared variables is:
1. The scope of a declared variable is limited to the context of its declaration location, while an undeclared variable is always global.
function x() { y = 1; // 在严格模式(strict mode)下会抛出 ReferenceError 异常 var z = 2; } x(); console.log(y); // 打印 "1" console.log(z); // 抛出 ReferenceError: z 未在 x 外部声明
2. Declared variables are created before any code is executed, while undeclared variables are only created when assignment operations are performed.
console.log(a); // 抛出ReferenceError。 console.log('still going...'); // 打印"still going..."。 Copy to Clipboard var a; console.log(a); // 打印"undefined"或""(不同浏览器实现不同)。 console.log('still going...'); // 打印"still going..."。
3. Declared variables are non-configurable properties of the context in which they are located, while non-declared variables are configurable (for example, non-declared variables can be deleted).
var a = 1; b = 2; delete this.a; // 在严格模式(strict mode)下抛出TypeError,其他情况下执行失败并无任何提示。 delete this.b; console.log(a, b); // 抛出ReferenceError。 // 'b'属性已经被删除。
Because of these three differences, failure to declare a variable will most likely lead to unexpected results. Therefore, it is recommended to always declare variables, whether they are within a function or global scope. Also, in ECMAScript 5 strict mode, assigning to an undeclared variable throws an error.
For more programming related knowledge, please visit: Programming Video! !
The above is the detailed content of what is javascript var. For more information, please follow other related articles on the PHP Chinese website!
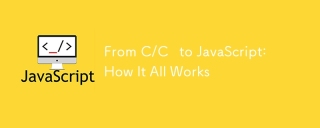
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
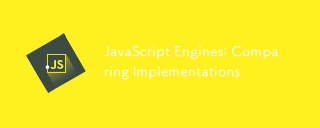
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
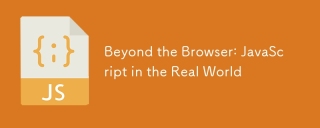
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
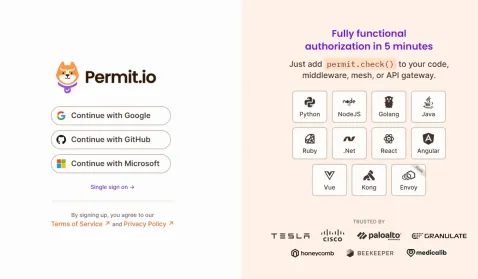
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
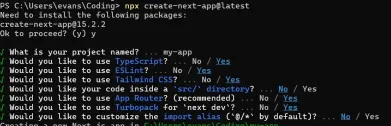
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
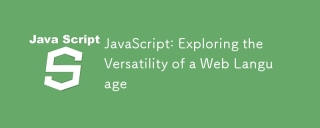
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
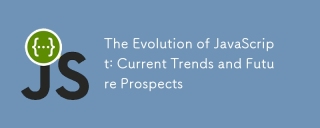
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
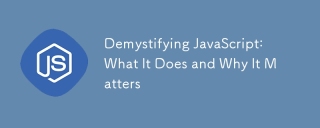
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
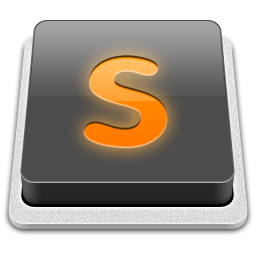
SublimeText3 Mac version
God-level code editing software (SublimeText3)
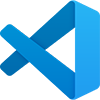
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft