The following is the tutorial column of golang to introduce to you the implementation of Golang channel. I hope it will be helpful to friends in need!
1. Introduction
Channel is the communication method between goroutines provided by Go language at the language level. Channels can be used to pass messages between two or more goroutines. Channel is an intra-process communication method, so the process of passing objects through channels is more consistent with the parameter passing behavior when calling functions. For example, pointers can also be passed. If cross-process communication is required, it is recommended to use a distributed system to solve the problem, such as using communication protocols such as Socket or HTTP.
Channel is type-related, that is to say, a channel can only pass one type of value, and this type needs to be specified when declaring the channel. Note that channel itself is also a native type in the Go language, with the same status as types such as map, so the channel itself can also be passed through the channel after being defined.
2. Underlying implementation
2.1 hchan structure
type hchan struct {
qcount uint // 队列中当前数据的个数
dataqsiz uint // size of the circular queue
buf unsafe.Pointer // 数据缓冲区,存放数据的环形数组
elemsize uint16 // channel中数据类型的大小(单个元素的大小)
closed uint32 // 表示channel是否关闭标识位
elemtype *_type // 队列中的元素类型
sendx uint // 当前发送元素的索引
recvx uint // 当前接收元素的索引
recvq waitq // 接受等待队列,由recv行为(也就是<-ch)阻塞在channel上的goroutine队列
sendq waitq // 发送等待队列, 由send行为(也就是ch<-)阻塞在channel上的goroutine队列
//lock保护chann中的所有字段,以及在此通道上阻塞的sudoG中的几个字段。
//保持此锁时不要更改另一个G状态(特别是没准备好G),因为这可能会因堆栈收缩而死锁
lock mutex
}
//发送及接收队列的·1结构体
type waitq struct {
first *sudog
last *sudog
}
-
qcount uint //The number of elements remaining in the current queue.
-
dataqsiz uint // Ring queue length, that is, the size of the buffer, that is, make(chan T, N), N.
-
buf unsafe.Pointer // Ring queue pointer.
-
elemsize uint16 // The size of each element.
-
closed uint32 // Indicates whether the current channel is closed. When a channel is created, this field is set to 0, which means the channel is open; by setting it to 1 by calling close, the channel is closed.
-
elemtype *_type // Element type, used for assignment during data transfer.
-
sendx uint and recvx uint is the status field of the ring buffer, which indicates the current index of the buffer - supports arrays from which it can send and receive data .
-
recvq waitq // Goroutine queue waiting to read messages.
-
sendq waitq // Goroutine queue waiting to write messages.
-
lock mutex // Mutex lock, locks the channel for each read and write operation, because sending and receiving must be mutually exclusive operations.
2.2 Creation process
2.2.1 Writing operation
1. Create a channel with buffer
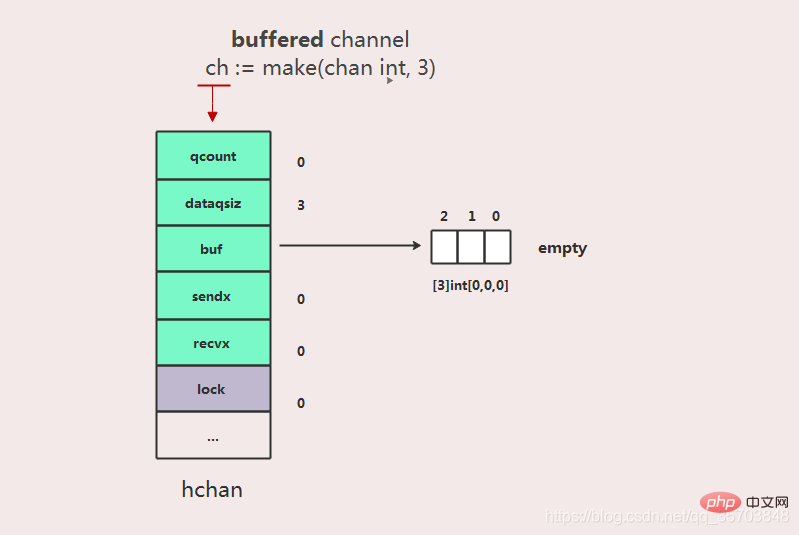
2. Write data to the channel
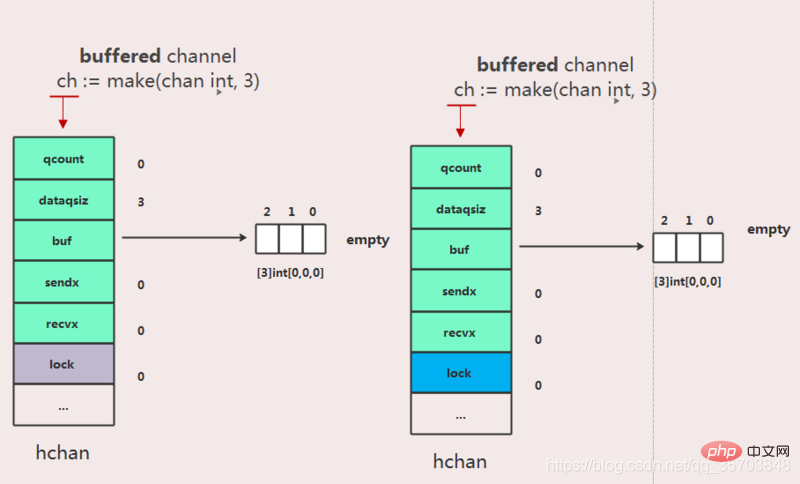
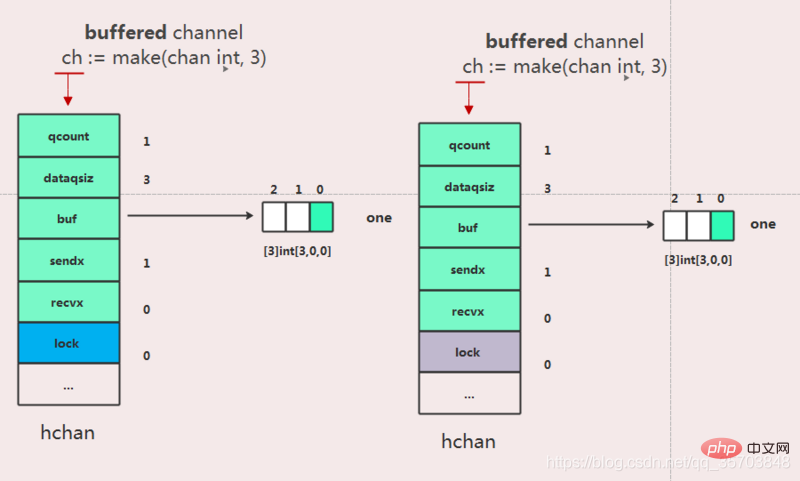
3.3 Writing process As follows:
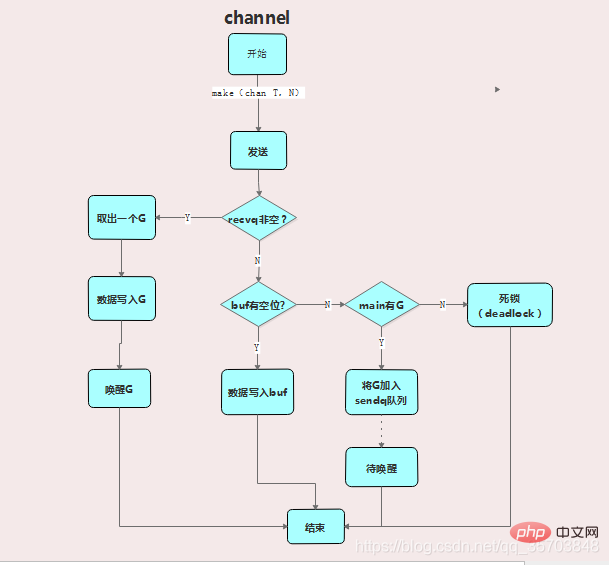
- Lock the entire pipeline structure.
- Confirm the writing, try to wait for the goroutine from the queue, and then write the elements directly to the goroutine.
- If recvq is empty, determine whether the buffer is available. If available, copies data from the current goroutine to the buffer.
- If the buffer is full, the elements to be written will be saved in the currently executing goroutine structure, and the current goroutine will be queued in sendq and suspended from running.
- The write is completed and the lock is released.
2.2.2 Reading process
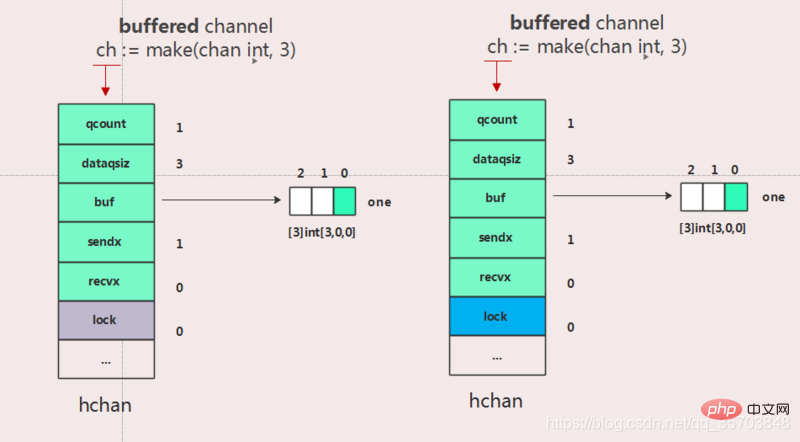
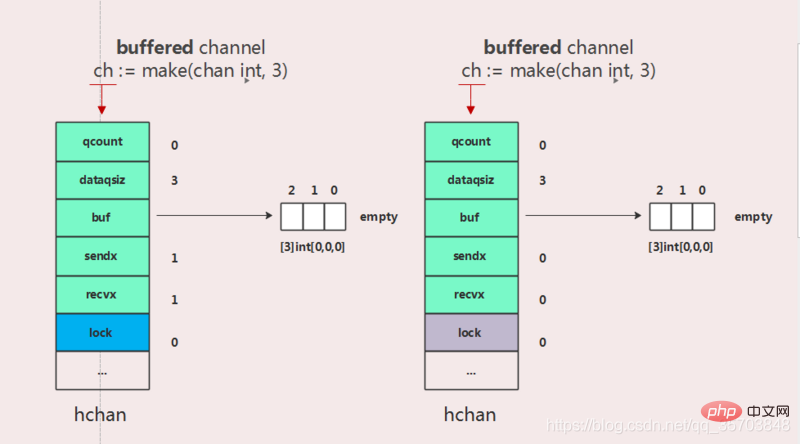
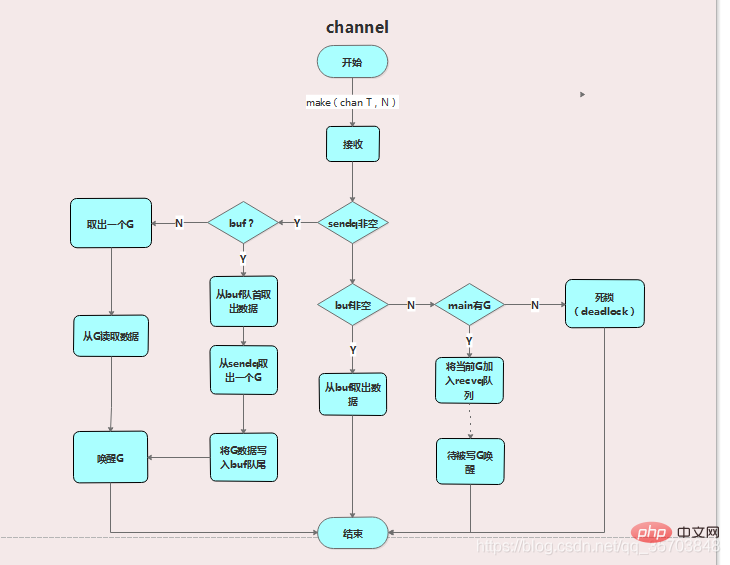
# #Read the channel global lock first. - Try sendq to get the waiting goroutine from the waiting queue.
- If there is a waiting goroutine and a buffer (the buffer is full), take the data from the head of the buffer queue, and then take out a goroutine from sendq. Store the data in the goroutine into the buf alignment, and end the reading to release the lock.
- If there is no waiting goroutine and there is data in the buffer, read the buffer data directly and explain the read release lock.
- If there is no waiting goroutine, and there is no buffer or the buffer area is empty, add the current goroutine to the denq queue, enter sleep, and wait to be awakened by writing goroutine. Finish releasing the lock.
-
The above is the detailed content of About the implementation of Golang channel. For more information, please follow other related articles on the PHP Chinese website!