Recommended (free): uni-app development tutorial
Article Directory
- Foreword
- 1. Use of properties and methods
- 2. Vue life cycle
- 3. Global variables
- 1. Public module
- 2. Mount Vue.prototype
- 3.globalData
- 4. Class and Style binding
- 1. Object syntax
- 2. Array syntax
- Summary
Preface
The main content of this article is the usage of Vue in uni-app, as follows:
Vue supports responsive data operations, It can realize the binding of data and events, and supports this transfer;
uni-app adds application life cycle and page life cycle based on the Vue instance life cycle;
3 ways to implement global variables, namely Public modules, mounting Vue.prototype
and globalData
;
Dynamic binding of Class and Style, including the use of object syntax and list syntax.
1. Use of properties and methods
Vue is a progressive framework based on JavaScript for building user interfaces, supportingResponsive data operation, after declaring a variable, the view will be re-rendered after the value of the variable changes, without the need to manually update the DOM node.
As you can see, all page file suffixes are .vue
, which is a single file. The variables are declared in the data attribute of the script
module. template
When using variables in a block, you need to include the variable with {<!-- -->{}}
;
You can also define methods to perform specific functions, which need to be included in In the methods attribute of the script
module, events can be bound in the component at the same time. The format is v-on:click="event name"
and @click="event name"
, corresponding events can be triggered based on conditions.
For the correspondence between web events and events in uni-app, please refer to https://uniapp.dcloud.io/use?id=Event Processor.
index.vue is as follows:
<template> <view class=""> <text>{{name}}</text> <button type="primary" @click="changeName">改变名字</button> </view></template><script> export default { data() { return { name: 'Corley' } }, onLoad() { }, onShow() { }, onHide() { }, methods: { changeName: function(){ this.name = 'Corlin' } }, }</script><style></style>
Among them, this
represents the Vue instance itself. In addition to calling properties, you can also call methods.
Display:
You can see that the name attribute has been changed.
If there are multiple functions nested in the function, there may be problems in passing this. In this case, you can use variables to replace , as follows:
<template> <view class=""> <text>{{name}}</text> <button type="primary" @click="changeName">改变名字</button> </view></template><script> var _self; export default { data() { return { name: 'Corley' } }, onLoad() { _self = this }, onShow() { }, onHide() { }, methods: { changeName: function(){ _self.name = 'Corlin'; setTimeout(function(){ _self.name = 'Corlin...' }, 2000); } }, }</script><style></style>
Display:
You can see that after clicking the button, the name is changed first, and then changed again after 2 seconds, which means _self
replaces this
successfully.
2. Vue life cycle
Vue supports instance life cycle, uni-app adds application life cycle on this basis and Page life cycle.
Vue instance life cycle hook automatically binds this context to the instance, so you can access data and perform operations on properties and methods.
details as follows:
Function | Meaning |
---|---|
After the instance is initialized, Data observer (data observer) and event/watcher event configuration are called before | |
is called immediately after the instance is created. At this step, the instance has completed the following configuration: data observer, property and method operations, and watch/event event callbacks. However, the mount phase has not started yet and the $el property is not yet available. | |
Called before the mount starts: the related render function is called for the first time. | |
Called after the instance is mounted, then el is replaced by the newly created vm.$el | |
Called when the data is updated, which occurs before the virtual DOM is patched. This is suitable for accessing the existing DOM before updating, such as manually removing an added event listener. | |
The virtual DOM is re-rendered and patched due to data changes, after which this hook will be called | |
Called when a component cached by keep-alive is activated | |
Called when a component cached by keep-alive is deactivated | |
Called before the instance is destroyed | |
Called after the instance is destroyed. After this hook is called, all instructions corresponding to the Vue instance are unbound, all event listeners are removed, and all child instances are destroyed. | |
Called when capturing an error from a descendant component. This hook receives three parameters: the error object, the component instance where the error occurred, and a string containing information about the source of the error. This hook can return false to prevent the error from propagating further upwards. |
The details are as follows:
Meaning | |
---|---|
Triggered when uni-app initialization is completed (only triggered once globally) | |
When uni-app starts, or enters the foreground display from the background | |
When uni-app enters the background from the front desk | |
When uni-app reports an error Triggered when | |
To monitor the data sent by the nvue page, please refer to nvue communicating with vue | |
Listening function for unhandled Promise rejection event (2.8.1) | |
There is no listening function on the page | |
Monitoring system theme changes |
Common page life cycles are as follows:
Meaning | |
---|---|
monitors page loading, its parameter is the data passed on the previous page, and the parameter type is Object (used for page parameters), refer to the example | |
Listen to the page display. Triggered every time the page appears on the screen, including returning from the lower-level page point to reveal the current page | |
Listens for the completion of the initial rendering of the page. Note that if the rendering speed is fast, | |
will be triggered before the page entry animation is completed | |
Listen for page unloading | |
Listen for window size changes | |
Monitor the user's pull-down action, generally used for pull-down refresh, refer to the example | |
The event when the page scrolls to the bottom (not scroll-view to the bottom), often used for pull-down Next page of data. See the notes below for details | |
Monitor page scrolling, the parameter is Object | |
Listen to the native title bar button click event, the parameter is Object |
The above is the detailed content of Learn to use Vue in uni-app. For more information, please follow other related articles on the PHP Chinese website!
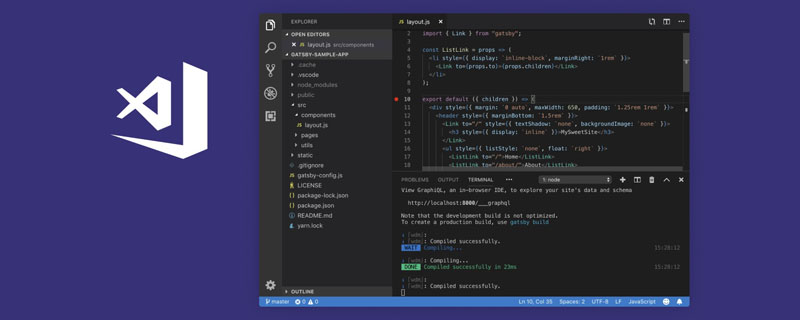
VSCode中如何开发uni-app?下面本篇文章给大家分享一下VSCode中开发uni-app的教程,这可能是最好、最详细的教程了。快来看看!
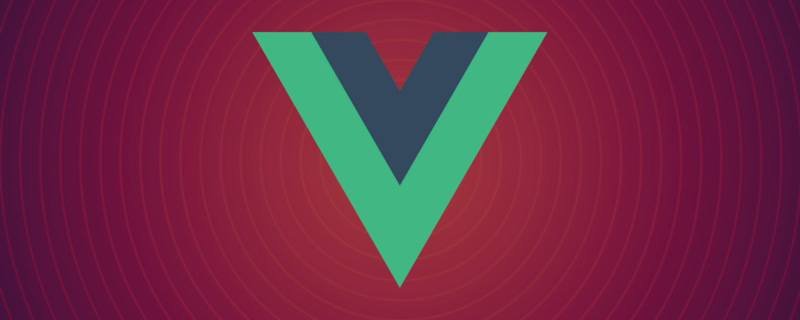
本篇文章带大家聊聊vue指令中的修饰符,对比一下vue中的指令修饰符和dom事件中的event对象,介绍一下常用的事件修饰符,希望对大家有所帮助!
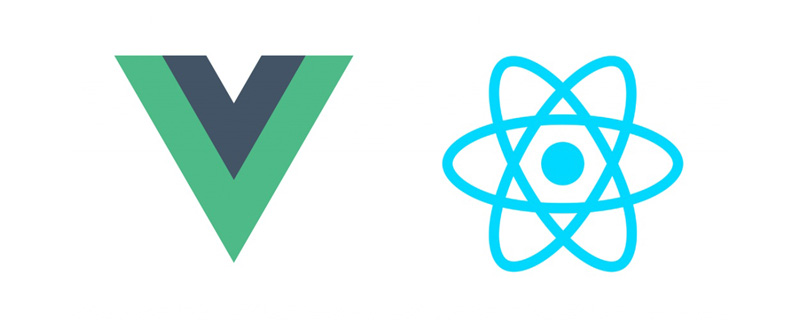
如何覆盖组件库样式?下面本篇文章给大家介绍一下React和Vue项目中优雅地覆盖组件库样式的方法,希望对大家有所帮助!
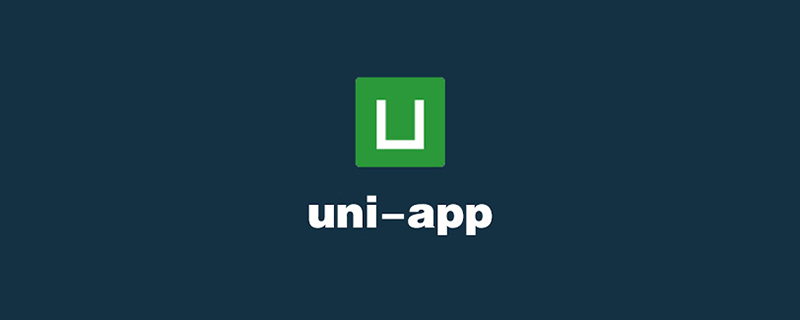
如何利用uniapp开发一个贪吃蛇小游戏?下面本篇文章就手把手带大家在uniapp中实现贪吃蛇小游戏,希望对大家有所帮助!


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
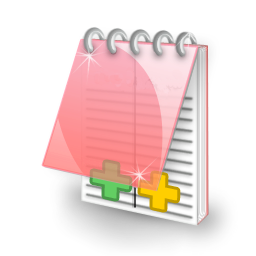
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version