[Related tutorial recommendations: React video tutorial]
Cross-site scripting (XSS) attack is a type of injecting malicious code into a web page and then executing it s attack. This is one of the most common forms of cyberattacks that front-end web developers have to deal with, so it's important to understand how the attack works and how to protect against it.
In this article, we’ll look at a few code examples written in React so you, too, can protect your site and users.
Example 1: Successful XSS attack in React
For all of our examples, we will implement the same basic functionality. We will have a search box on the page where the user can enter text. Clicking the "Go" button will simulate running a search, and then some confirmation text will appear on the screen and repeat to the user the terms they searched for. This is standard behavior for any website that allows you to search.
Pretty simple, right? What could go wrong?
Okay, what if we typed some HTML into the search box? Let’s try the following code snippet:
<img src="/static/imghwm/default1.png" data-src="1" class="lazy" alt="How to prevent XSS attacks in React? (code example)" >
What happens now?
Wow, onerror
event handler has been executed! That's not what we want! We just unknowingly executed scripts from untrusted user input.
#Then, a broken image will be rendered on the page, and that’s not what we want either.
So how did we get here? Well, in this example of the JSX that renders the search results, we use the following code:
<p> You searched for: <b><span></span></b> </p>
The reason the user input is parsed and rendered is that we use the dangerouslySetInnerHTML
attribute, which is A feature in React that works just like the native innerHTML
browser API. For this reason, it is generally considered unsafe to use this attribute.
Example 2: Failed XSS attack in React
Now, let’s look at an example of a successful defense against an XSS attack. The fix here is very simple: in order to render user input safely, we should not use the dangerouslySetInnerHTML
attribute. Instead, let's code the output like this:
<p> You searched for: <b>{this.state.submittedSearch}</b> </p>
We'll take the same input, but this time, here's the output:
Great! User input is rendered as text on the screen and the threat has been neutralized.
This is good news! React escapes rendered content by default and treats all data as text strings, which is equivalent to using the native textContent
browser API.
Example 3: Cleaning HTML content in React
So, the advice here seems simple. Just don't use dangerouslySetInnerHTML
in your React code and you'll be fine. But what if you find yourself needing to use this feature?
For example, maybe you're pulling content from a content management system (CMS) like Drupal, and some of that content contains markup. (BTW, I probably wouldn't recommend including tags in text content and translations from the CMS in the first place, but for this example, we'll assume your opinion is overruled and the tagged content will remain.)
In this case, you really want to parse the HTML and render it on the page. So, how do you do this safely?
The answer is to clean the HTML before rendering it. Instead of fully escaping the HTML, you'll run the content through a function to remove any potentially malicious code before rendering.
There are many good HTML sanitizing libraries you can use. As with anything to do with cybersecurity, it's best not to write this stuff yourself. Some people are much smarter than you, whether they are good or bad, they think more than you do, be sure to use tried and tested solutions.
One of my favorite sanitizer libraries is called sanitize-html (https://www.npmjs.com/package/sanitize-html), and it does exactly what its name suggests. You start with some dirty HTML, run it through a function, and get some nice, clean, safe HTML as output. You can even customize the allowed HTML tags and attributes if you want more control than their default settings offer.
End
That’s it. How to perform XSS attacks, how to prevent them, and how to safely parse HTML content if necessary.
I wish you happy programming and safety!
The complete code example can be found on GitHub: https://github.com/thawkin3/xss-demo
Original address: https://blog. zhangbing.site/2019/11/24/protecting-against-xss-attacks-in-react/
For more programming-related knowledge, please visit: Programming Learning! !
The above is the detailed content of How to prevent XSS attacks in React? (code example). For more information, please follow other related articles on the PHP Chinese website!
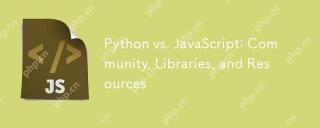
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
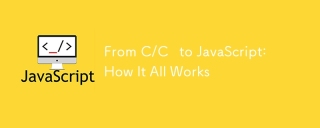
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
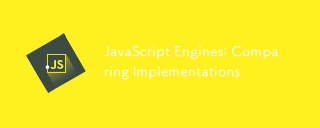
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
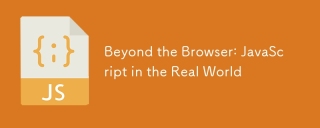
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
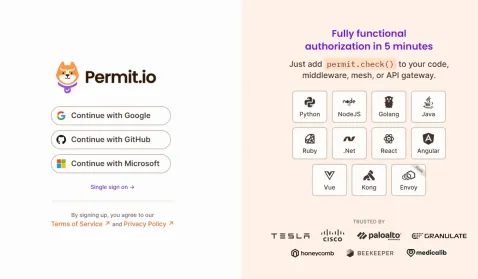
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
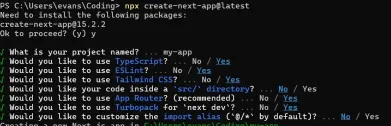
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
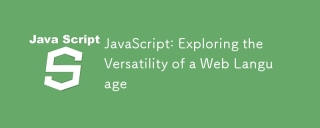
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
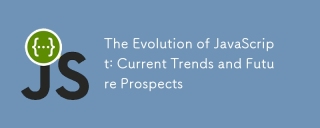
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
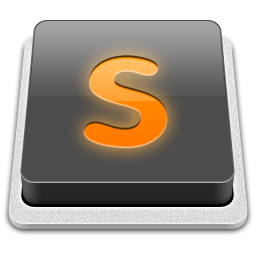
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
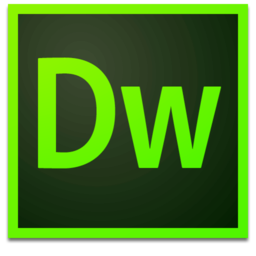
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool