JavaScript does not have any printing or output functions.
In HTML, JavaScript is commonly used to manipulate HTML elements.
Manipulate HTML elements
To access an HTML element from JavaScript, you can use the document.getElementById(id) method.
Please use the "id" attribute to identify HTML elements and innerHTML to get or insert element content:
Example
<!DOCTYPE html> <html> <body> <h1 id="我的第一个-Web-页面">我的第一个 Web 页面</h1> <p id="demo">我的第一个段落</p> <script> document.getElementById("demo").innerHTML = "段落已修改。"; </script> </body> </html>
The above JavaScript statement (in the <script> tag) can be executed in a web browser: </script>
- document.getElementById("demo") is JavaScript code that uses the id attribute to find an HTML element.
- innerHTML = "Paragraph changed." is the JavaScript code used to modify the HTML content (innerHTML) of the element.
Write to HTML document
For testing purposes, you can write JavaScript directly in the HTML document:
Example
<!DOCTYPE html> <html> <body> <h1 id="我的第一个-Web-页面">我的第一个 Web 页面</h1> <p>我的第一个段落。</p> <script> document.write(Date()); </script> </body> </html>
Please use document.write() to only write content to the document.
If document.write is executed after the document has finished loading, the entire HTML page will be overwritten.
Example
<!DOCTYPE html> <html> <body> <h1 id="我的第一个-Web-页面">我的第一个 Web 页面</h1> <p>我的第一个段落。</p> <button onclick="myFunction()">点我</button> <script> function myFunction() { document.write(Date()); } </script> </body> </html>
Write to console
If your browser supports debugging, you can use the console.log() method to display JavaScript values in the browser.
Use F12 in the browser to enable debug mode, and click the "Console" menu in the debug window.
Example
<!DOCTYPE html> <html> <body> <h1 id="我的第一个-Web-页面">我的第一个 Web 页面</h1> <script> a = 5; b = 6; c = a + b; console.log(c); </script> </body> </html>
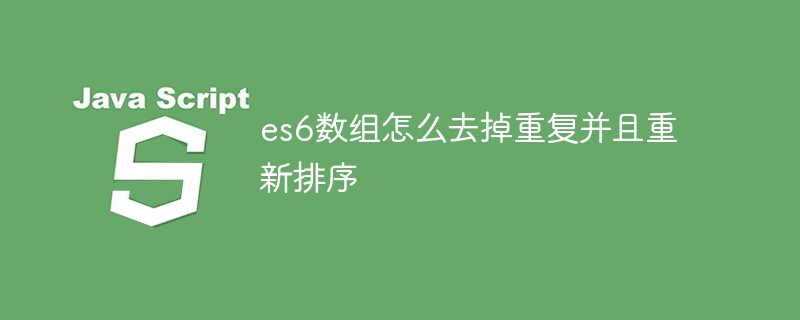
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
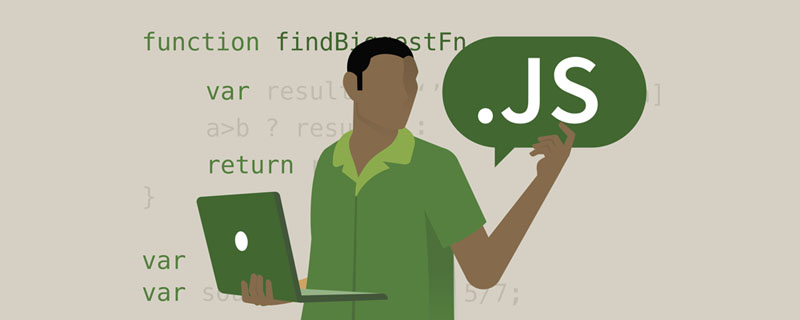
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
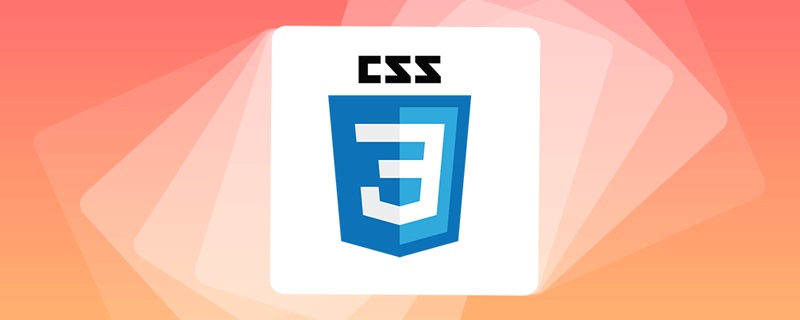
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
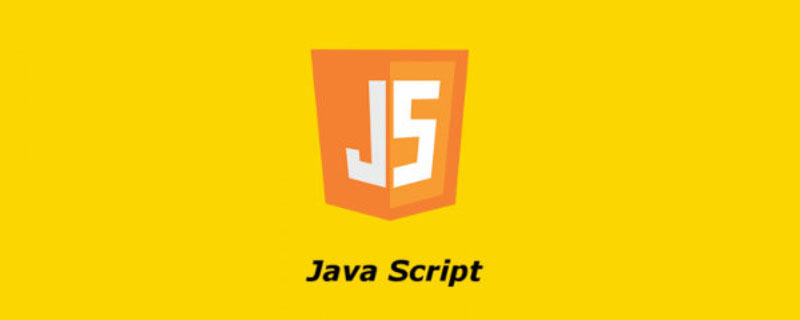
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
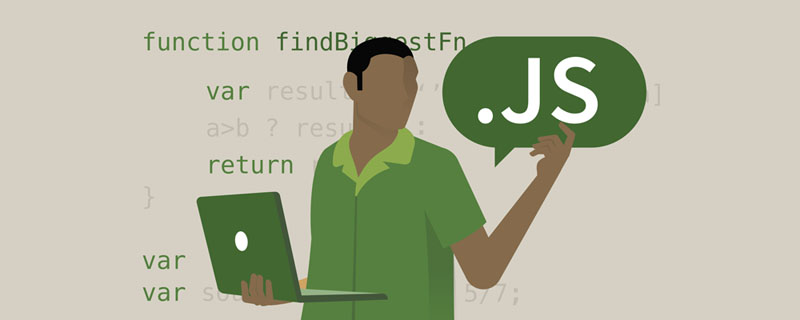
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
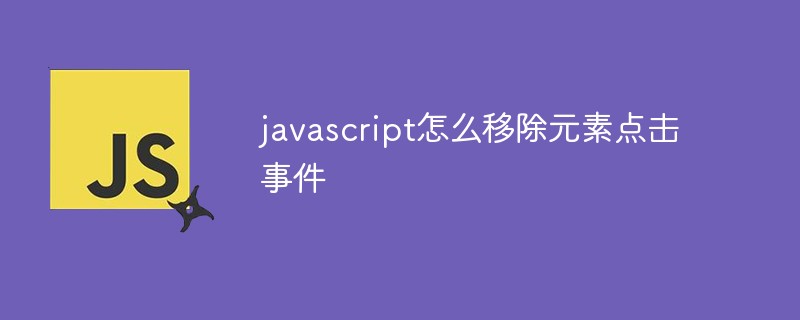
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
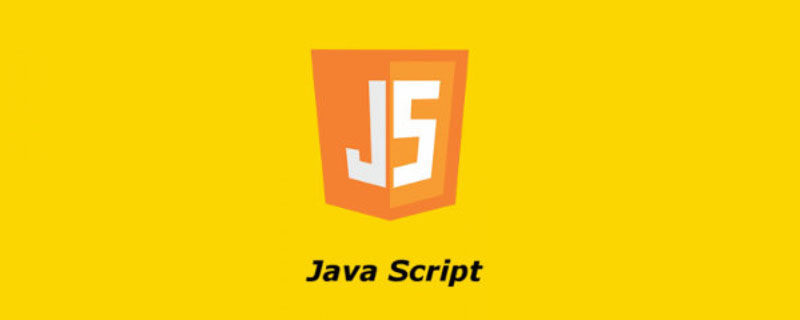
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
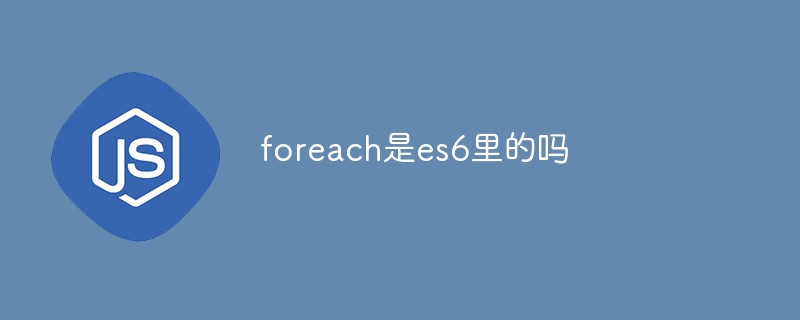
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
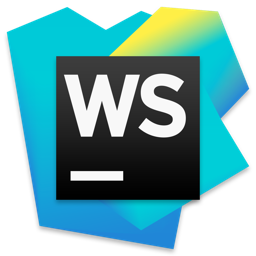
WebStorm Mac version
Useful JavaScript development tools
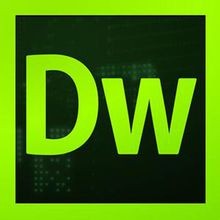
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
