Methods to obtain data in react: 1. Use life cycle methods to request data; 2. Use Hooks to obtain data; 3. Use suspend to obtain data.
The operating environment of this tutorial: windows10 system, react16, this article is applicable to all brands of computers.
Methods to get data in react:
1. Use life cycle methods to request data
Application Employees.org Do two things:
1. Get 20 employees as soon as you enter the program.
2. You can filter employees by filtering conditions.
Before implementing these two requirements, let’s review the two life cycle methods of react class components:
componentDidMount()
: Executed after the component is mountedcomponentDidUpdate(prevProps)
: Executed when props or state changes
ComponentUse the above two life cycle methods to implement the acquisition logic:
import EmployeesList from "./EmployeesList"; import { fetchEmployees } from "./fake-fetch"; class EmployeesPage extends Component { constructor(props) { super(props); this.state = { employees: [], isFetching: true }; } componentDidMount() { this.fetch(); } componentDidUpdate(prevProps) { if (prevProps.query !== this.props.query) { this.fetch(); } } async fetch() { this.setState({ isFetching: true }); const employees = await fetchEmployees(this.props.query); this.setState({ employees, isFetching: false }); } render() { const { isFetching, employees } = this.state; if (isFetching) { return <div>获取员工数据中...</div>; } return <EmployeesList employees={employees} />; } }
Open codesandbox to view the <employeespage></employeespage>
acquisition process.
<employeespage></employeespage>
There is an asynchronous method fetch() to obtain data. After the get request is completed, use the setState method to update employees.
this.fetch()
Executed in the componentDidMount()
life cycle method: it gets the employee data when the component is initially rendered.
When our keywords are filtered, props.query will be updated. Whenever props.query is updated, componentDidUpdate() will re-execute this.fetch().
While lifecycle methods are relatively easy to master, class-based methods have boilerplate code that makes reusability difficult.
Advantages
This method is easy to understand: componentDidMount()
Gets data on the first render, while componentDidUpdate( )
Re-obtain data when props are updated.
Disadvantages
Boiler code
Class-based components need to inherit React.Component and execute super(props)# in the constructor ## etc.
componentDidMount()The code in
componentDidUpdate() is mostly repeated.
2. Use Hooks to obtain data
Hooks are a better choice for obtaining data based on classes. As simple functions, Hooks do not need to be inherited like class components and are easier to reuse. Briefly recalluseEffect(callback[, deps]) Hook. This hook executes the callback after mounting and re-renders when dependency deps change.
import EmployeesList from "./EmployeesList"; import { fetchEmployees } from "./fake-fetch"; function EmployeesPage({ query }) { const [isFetching, setFetching] = useState(false); const [employees, setEmployees] = useState([]); useEffect(function fetch() { (async function() { setFetching(true); setEmployees(await fetchEmployees(query)); setFetching(false); })(); }, [query]); if (isFetching) { return <div>Fetching employees....</div>; } return <EmployeesList employees={employees} />; }Open
codesandbox to see how useEffect() obtains data.
useEffect(fetch, [query]) in the <employeespage> function component, the fetch callback is executed after the initial rendering. In addition, the fetch method will also be re-executed when the dependency query is updated. </employeespage>
import React, { useState } from 'react'; import EmployeesList from "./EmployeesList"; import { fetchEmployees } from "./fake-fetch"; function useEmployeesFetch(query) { // 这行有变化 const [isFetching, setFetching] = useState(false); const [employees, setEmployees] = useState([]); useEffect(function fetch { (async function() { setFetching(true); setEmployees(await fetchEmployees(query)); setFetching(false); })(); }, [query]); return [isFetching, employees]; } function EmployeesPage({ query }) { const [employees, isFetching] = useEmployeesFetch(query); // 这行有变化 if (isFetching) { return <div>Fetching employees....</div>; } return <EmployeesList employees={employees} />; }Mention the required value from
useEmployeesFetch(). The component <employeespage> has no corresponding acquisition logic and is only responsible for rendering the interface. </employeespage>
Advantages
- Clear and simple, Hooks have no boilerplate code because they are ordinary functions.
- Reusability, the data acquisition logic implemented in Hooks is easy to reuse.
Disadvantages
Requires prior knowledgeHooks are a bit counterintuitive, so you must understand them before using them, Hooks dependencies closures, so be sure to understand them well.Necessity
Using Hooks, you still have to use an imperative approach to perform data retrieval. Foshan vi design https://www.houdianzi.com/fsvi/ Pea resource search directory https://55wd.com3. Use suspense to obtain data
Suspense provides a declarative way to asynchronously obtain data in React. Note: As of November 2019, Suspense is in an experimental phase.Package components that perform asynchronous operations:
<Suspense fallback={<span>Fetch in progress...</span>}>
<FetchSomething />
</Suspense>
.
import React, { Suspense } from "react"; import EmployeesList from "./EmployeesList"; function EmployeesPage({ resource }) { return ( <Suspense fallback={<h1 id="Fetching-nbsp-employees">Fetching employees....</h1>}> <EmployeesFetch resource={resource} /> </Suspense> ); } function EmployeesFetch({ resource }) { const employees = resource.employees.read(); return <EmployeesList employees={employees} />; }Open codesandbox to see how Suspense obtains data.
resource.employees The biggest advantage is: Suspense handles asynchronous operations in a declarative and synchronous manner. Components do not have complex data retrieval logic, but use resources in a declarative manner to render content. There is no life cycle inside the component, no Hooks, async/await, no callbacks: only the display interface. Advantages Declarative Suspense performs asynchronous operations in React in a declarative way. Simple Declarative code is simple to use and these components have no complex data retrieval logic. Loose Coupling and Fetching Implementation Components using Suspense don’t see how to get data: use REST or GraphQL. Suspense sets a boundary to protect fetch details from leaking into the component. Standard status If multiple get operations are requested, Suspense will use the latest get request. 4. Summary For a long time, lifecycle methods have been the only solution for how to get data. However, using them to obtain data comes with a lot of boilerplate code, duplication, and reusability issues. Related free learning recommendations: JavaScript (video) Use the Suspense processing component to pass the obtained data to the
component.
in
The above is the detailed content of How to get data in react. For more information, please follow other related articles on the PHP Chinese website!
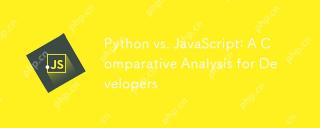
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
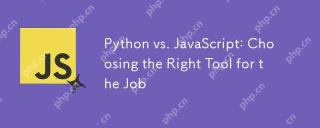
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
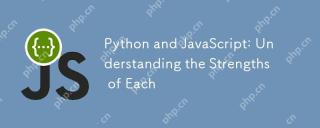
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
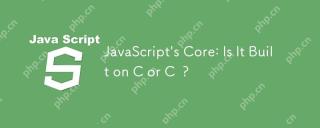
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
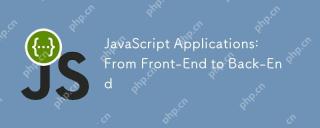
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
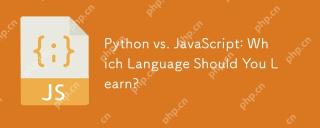
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
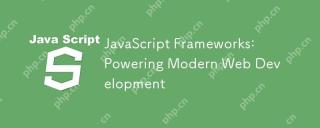
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
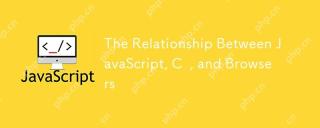
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
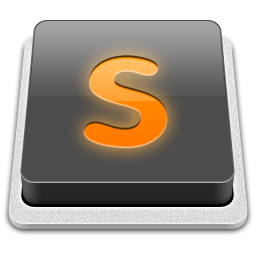
SublimeText3 Mac version
God-level code editing software (SublimeText3)
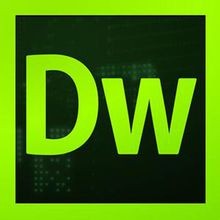
Dreamweaver CS6
Visual web development tools
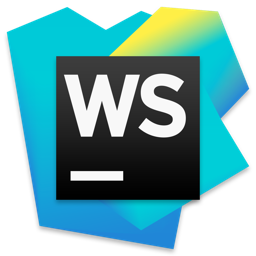
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
