


Master the conditional branch structure of shell programming through several cases
shell脚本是任何一个后端程序员都应该去掌握的技能,今天,我们来一起学习下它的条件分支结构,然后通过几个案例去掌握它。
首先,我们来看shell脚本的基本结构,基本结构如下:
#!/bin/bash 代码
下面,我们来编写一个最简单的shell脚本程序吧。
#!/bin/bash echo 'hello world'
当上面程序执行后,就会在屏幕上打印出hello world字符。
接下来,我们再来看看条件分支结构,shell脚本关于条件分支的语句有if、case。
if
和其他编程语言一样,shell程序的if语句,条件分支也分为单分支、双分支以及多分支。
# 单分支 if 条件 ;then …… fi # 双分支 if 条件 ;then …… else …… fi # 多分支 if 条件;then …… elif 条件;then …… else …… fi
首先,我们来用一个简单的例子来练练手,写一个shell脚本,该脚本功能是当用户输入一个得分时,程序通过得分来输出不同的评语,不及格、良好、优秀等。
首先,我们需要先提示用户,让用户输入一个三位数以内的数字。当用户输入的格式不正确的时候,需要告诉用户重新输入成绩,然后退出程序。代码如下:
read -p "请输入成绩,成绩范围0-100: " score if [ -z `echo $score | egrep '^[0-9]+$'` ];then echo "输入的成绩格式不正确" fi
上述代码,我们用到了if的单分支结构。接下来,我们需要用到多分支了,根据成绩打印出不同的评语。
if ((score >= 90));then echo '优秀' elif ((score >= 80));then echo '良好' elif ((score >= 70));then echo '一般' elif ((score >= 60 ));then echo '及格' else echo '不及格' fi
上述代码非常的简单,下面我们贴出完整的代码,完整代码如下:
#!/bin/bash read -p "请输入成绩,成绩范围0-100: " score if [ -z `echo $score | egrep '^[0-9]+$'` ];then echo "输入的成绩格式不正确" fi if ((score >= 90));then echo '优秀' elif ((score >= 80));then echo '良好' elif ((score >= 70));then echo '一般' elif ((score >= 60 ));then echo '及格' else echo '不及格' fi
case
下面,我们来看另一个条件分支语句case,它的基本结构如下:
case $变量 in "内容1") 代码块1 ;; "内容2") 代码块2 ;; …… *) 代码块n ;; esac
上述的内容意思是这样的,当“变量值”等于“内容1”时,执行代码块1,等于“内容2”时,执行代码块2,如果前面的都不满足,则执行代码块n。
接下里,我们通过一个简单的案例来看看case是如何运用的。
#!/bin/bash case $1 in "start") echo "this code is start" ;; "stop") echo "this code is stop" ;; "restart") echo "this code is restart" ;; *) echo "Usage ${0} {start|stop|restart}" ;; esac
上述代码的含义是,当用户输入参数为start时,程序打印this code is start,当输入的参数为stop时,输出this code is stop,当输入参数为restart时,输出this code is restart,否则的话输入“Usage 脚本文件名 {start|stop|restart}”。
The above is the detailed content of Master the conditional branch structure of shell programming through several cases. For more information, please follow other related articles on the PHP Chinese website!
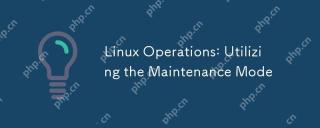
Linux maintenance mode can be entered through the GRUB menu. The specific steps are: 1) Select the kernel in the GRUB menu and press 'e' to edit, 2) Add 'single' or '1' at the end of the 'linux' line, 3) Press Ctrl X to start. Maintenance mode provides a secure environment for tasks such as system repair, password reset and system upgrade.
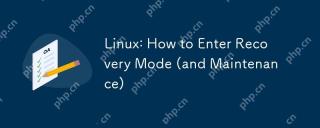
The steps to enter Linux recovery mode are: 1. Restart the system and press the specific key to enter the GRUB menu; 2. Select the option with (recoverymode); 3. Select the operation in the recovery mode menu, such as fsck or root. Recovery mode allows you to start the system in single-user mode, perform file system checks and repairs, edit configuration files, and other operations to help solve system problems.
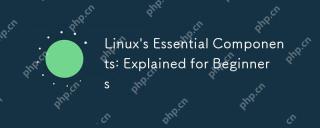
The core components of Linux include the kernel, file system, shell and common tools. 1. The kernel manages hardware resources and provides basic services. 2. The file system organizes and stores data. 3. Shell is the interface for users to interact with the system. 4. Common tools help complete daily tasks.
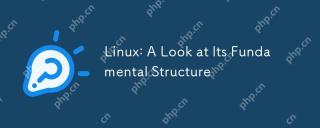
The basic structure of Linux includes the kernel, file system, and shell. 1) Kernel management hardware resources and use uname-r to view the version. 2) The EXT4 file system supports large files and logs and is created using mkfs.ext4. 3) Shell provides command line interaction such as Bash, and lists files using ls-l.
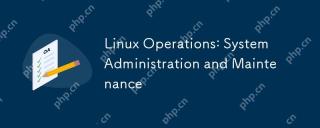
The key steps in Linux system management and maintenance include: 1) Master the basic knowledge, such as file system structure and user management; 2) Carry out system monitoring and resource management, use top, htop and other tools; 3) Use system logs to troubleshoot, use journalctl and other tools; 4) Write automated scripts and task scheduling, use cron tools; 5) implement security management and protection, configure firewalls through iptables; 6) Carry out performance optimization and best practices, adjust kernel parameters and develop good habits.
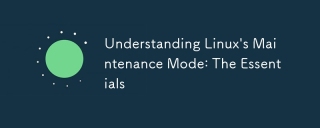
Linux maintenance mode is entered by adding init=/bin/bash or single parameters at startup. 1. Enter maintenance mode: Edit the GRUB menu and add startup parameters. 2. Remount the file system to read and write mode: mount-oremount,rw/. 3. Repair the file system: Use the fsck command, such as fsck/dev/sda1. 4. Back up the data and operate with caution to avoid data loss.
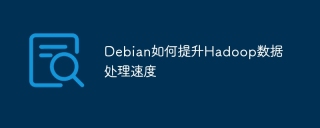
This article discusses how to improve Hadoop data processing efficiency on Debian systems. Optimization strategies cover hardware upgrades, operating system parameter adjustments, Hadoop configuration modifications, and the use of efficient algorithms and tools. 1. Hardware resource strengthening ensures that all nodes have consistent hardware configurations, especially paying attention to CPU, memory and network equipment performance. Choosing high-performance hardware components is essential to improve overall processing speed. 2. Operating system tunes file descriptors and network connections: Modify the /etc/security/limits.conf file to increase the upper limit of file descriptors and network connections allowed to be opened at the same time by the system. JVM parameter adjustment: Adjust in hadoop-env.sh file
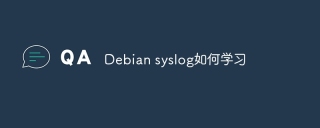
This guide will guide you to learn how to use Syslog in Debian systems. Syslog is a key service in Linux systems for logging system and application log messages. It helps administrators monitor and analyze system activity to quickly identify and resolve problems. 1. Basic knowledge of Syslog The core functions of Syslog include: centrally collecting and managing log messages; supporting multiple log output formats and target locations (such as files or networks); providing real-time log viewing and filtering functions. 2. Install and configure Syslog (using Rsyslog) The Debian system uses Rsyslog by default. You can install it with the following command: sudoaptupdatesud


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
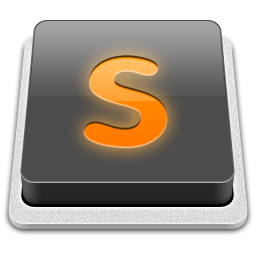
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.