Usage of this keyword in java: 1. When member variables and local variables have the same name, when using this in a method, it means the member variable in the class where the method is located; 2. In the constructor , you can call other constructors in the same class through this; 3. Use this to pass multiple parameters at the same time.
Related recommendations: "Java Video Tutorial"
1. When member variables and local variables overlap When using this in a method, it refers to the member variable in the class where the method is located. (this is the current object itself)
public class Hello { String s = "Hello"; public Hello(String s) { System.out.println("s = " + s); System.out.println("1 -> this.s = " + this.s); this.s = s;//把参数值赋给成员变量,成员变量的值改变 System.out.println("2 -> this.s = " + this.s); } public static void main(String[] args) { Hello x = new Hello("HelloWorld!"); System.out.println("s=" + x.s);//验证成员变量值的改变 } }
The result is:
s = HelloWorld! 1 -> this.s = Hello 2 -> this.s = HelloWorld! s=HelloWorld!
In this example, in the constructor Hello, the parameter s has the same name as the member variable s of the class Hello. At this time If you directly operate on s, you will operate on the parameter s. If you want to operate on the member variable s of class Hello, you should use this for reference. The first line of the running result is to directly print the parameter s passed in the constructor; the second line is to print the member variable s; the third line is to first assign the passed parameter s value to the member variable s and then Print, so the result is HelloWorld! And the fourth line directly prints the value of the member variable in the class in the main function, and you can also verify the change of the member variable value.
2. When passing yourself as a parameter, you can also use this. (this is passed as the current parameter)
class A { public A() { new B(this).print();// 调用B的方法 } public void print() { System.out.println("HelloAA from A!"); } } class B { A a; public B(A a) { this.a = a; } public void print() { a.print();//调用A的方法 System.out.println("HelloAB from B!"); } } public class HelloA { public static void main(String[] args) { A aaa = new A(); aaa.print(); B bbb = new B(aaa); bbb.print(); } }
The result is:
HelloAA from A! HelloAB from B! HelloAA from A! HelloAA from A! HelloAB from B!
In this example , in the constructor of object A, use new B(this) to pass object A itself as a parameter to the constructor of object B.
3. Sometimes, we will use some internal classes and anonymous classes, such as event processing. When this is used in an anonymous class, this refers to the anonymous class or inner class itself. At this time, if we want to use the methods and variables of the external class, we should add the class name of the external class. Such as:
public class HelloB { int i = 1; public HelloB() { Thread thread = new Thread() { public void run() { for (int j=0;j<p>In the above example, thread is an anonymous class object. In its definition, its run function uses the run function of the external class. At this time, since the function has the same name, calling it directly will not work. There are two ways at this time. One is to change the name of the external run function, but this method is not advisable for an application that is halfway developed. Then you can use the method in this example to use the class name of the external class plus this reference to indicate that the method run of the external class is to be called. </p><p>4. In the constructor, other constructors in the same class can be called through this. For example: </p><pre class="brush:php;toolbar:false">public class ThisTest { ThisTest(String str) { System.out.println(str); } ThisTest() { this("this测试成功!"); } public static void main(String[] args) { ThisTest thistest = new ThisTest(); } }
In order to explain the usage of this more accurately, another example is:
public class ThisTest { private int age; private String str; ThisTest(String str) { this.str=str; System.out.println(str); } ThisTest(String str,int age) { this(str); this.age=age; System.out.println(age); } public static void main(String[] args) { ThisTest thistest = new ThisTest("this测试成功",25); } }
The result is:
this测试成功 25
It is worth noting:
1: When calling another constructor during construction, the calling action must be placed at the very beginning.
2: The constructor cannot be called in any function other than the constructor.
3: Only one constructor can be called within a constructor.
5. This passes multiple parameters at the same time.
public class TestClass { int x; int y; static void showtest(TestClass tc) {//实例化对象 System.out.println(tc.x + " " + tc.y); } void seeit() { showtest(this); } public static void main(String[] args) { TestClass p = new TestClass(); p.x = 9; p.y = 10; p.seeit(); } }
The result is:
9 10
showtest(this) in the code, this here is to pass the currently instantiated p to the showtest() method, and then it runs.
For more programming-related knowledge, please visit: Introduction to Programming! !
The above is the detailed content of How to use this keyword in java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
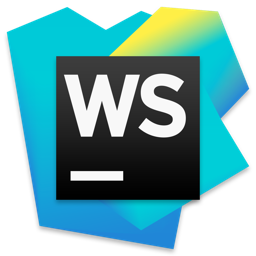
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
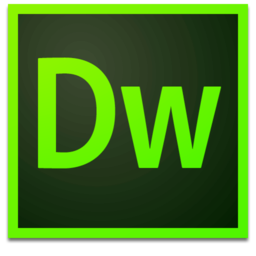
Dreamweaver Mac version
Visual web development tools
