Java's three factory patterns: 1. Simple factory pattern, which provides a function to create object instances without caring about its specific implementation; 2. Factory method pattern; 3. Abstract factory pattern, which provides a function to create an object instance. An interface for a family of related or interdependent objects without specifying their concrete classes.
(Recommended tutorial: java introductory tutorial)
1. Simple factory pattern
Definition of simple factory: Provides a function to create object instances without caring about its specific implementation. The type of the created instance can be an interface, an abstract class, or a specific class
Implementing the car interface
public interface Car { String getName(); }
Benz class
public class Benz implements Car { @Override public String getName() { return "Benz"; } }
BMW type
public class BMW implements Car { @Override public String getName() { return "BMW"; } }
Simple factory that can produce both BMW and Mercedes-Benz
public class SimpleFactory { public Car getCar(String name){ if (name.equals("BMW")){ return new BMW(); }else if (name.equals("benz")){ return new Benz(); }else { System.out.println("不好意思,这个品牌的汽车生产不了"); return null; } } }
Test class
public class SimpleFactoryTest { public static void main(String[] args){ SimpleFactory simpleFactory = new SimpleFactory(); Car car = simpleFactory.getCar("BMW"); System.out.println(car.getName()); } }
Test result
BMW
According to the definition of a simple factory, users only need the product and don’t care how the product is produced, and it looks perfect. But think about it, are there any factories in this world that produce everything?
Obviously does not exist. Every car brand has its own production factory and its own production technology. Mapping to the spring framework, we have many types of beans that need to be produced. If we only rely on a simple factory to implement it, how many if..else ifs do we have to nest in the factory class?
Moreover, when we produce a car in the code, it just comes out with a new one, but in actual operation we don’t know how many operations are required. Loading, registration and other operations will be reflected in the factory class, so this class It will become chaotic and inconvenient to manage, so each brand should have its own production category.
Because it is dedicated, it is professional. At this time, the factory method appeared.
2. Factory method pattern
Factory interface
//定义一个工厂接口,功能就是生产汽车 public interface Factory { Car getCar(); }
Benz factory
public class BenzFactory implements Factory { @Override public Car getCar() { return new Benz(); } }
BMW Factory
public class BMWFactory implements Factory{ @Override public Car getCar() { return new BMW(); } }
Test category
public class FactoryTest { public static void main(String[] args){ Factory bmwFactory = new BMWFactory(); System.out.println(bmwFactory.getCar().getName()); Factory benzFactory = new BenzFactory(); System.out.println(benzFactory.getCar().getName()); } }
Test result
BMW Benz
According to the above It can be seen from the code that cars of different brands are produced by different factories, and they seem to be perfect. But if you look at the test category, when a person wants to buy a BMW car (assuming there is no seller), then he has to go to the BMW factory to produce one for him, and a few days later he wants to buy a Mercedes-Benz. When buying a car, you have to go to the Mercedes-Benz factory to hire someone to produce it, which undoubtedly increases the complexity of the user's operation. So is there a user-friendly method? At this time, the abstract factory pattern appeared.
3. Abstract Factory Pattern
Abstract Factory
public abstract class AbstractFactory { protected abstract Car getCar(); //这段代码就是动态配置的功能 //固定模式的委派 public Car getCar(String name){ if("BMW".equalsIgnoreCase(name)){ return new BmwFactory().getCar(); }else if("Benz".equalsIgnoreCase(name)){ return new BenzFactory().getCar(); }else if("Audi".equalsIgnoreCase(name)){ return new AudiFactory().getCar(); }else{ System.out.println("这个产品产不出来"); return null; } } }
Default Factory
public class DefaultFactory extends AbstractFactory { private AudiFactory defaultFactory = new AudiFactory(); public Car getCar() { return defaultFactory.getCar(); } }
BMW Factory
public class BMWFactory extends AbstractFactory { @Override public Car getCar() { return new BMW(); } }
Benz Factory
public class BenzFactory extends AbstractFactory { @Override public Car getCar() { return new Benz(); } }
Test Class
public class AbstractFactoryTest { public static void main(String[] args) { DefaultFactory factory = new DefaultFactory(); System.out.println(factory.getCar("Benz").getName()); } }
Test results
Benz
As can be seen from the above code, if the user needs a car, he only needs to go to the default factory to put forward his needs (incoming parameters ), you can get the products you want without having to find different production factories based on the products, which is convenient for users to operate.
Note: Some people sneer at design patterns, and some people respect them as gods, but I agree with them.
According to my rough understanding, the classic thing about design patterns is that it solves the pain of both the person who writes the code and the person who calls the code. Different design patterns are only applicable to Different scenarios. As for whether to use it or not, and how to use it, you need to consider it carefully.
But you shouldn’t use it just for the sake of using it. The subtleties can only be left to everyone to taste slowly.
For more programming-related knowledge, please visit: Programming Courses! !
The above is the detailed content of What are the three factory patterns in java?. For more information, please follow other related articles on the PHP Chinese website!
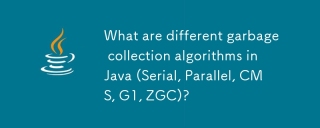
The article discusses various Java garbage collection algorithms (Serial, Parallel, CMS, G1, ZGC), their performance impacts, and suitability for applications with large heaps.

The article discusses the Java Virtual Machine (JVM), detailing its role in running Java programs across different platforms. It explains the JVM's internal processes, key components, memory management, garbage collection, and performance optimizatio
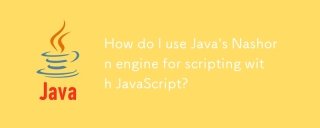
Java's Nashorn engine enables JavaScript scripting within Java apps. Key steps include setting up Nashorn, managing scripts, and optimizing performance. Main issues involve security, memory management, and future compatibility due to Nashorn's deprec
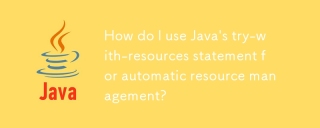
Java's try-with-resources simplifies resource management by automatically closing resources like file streams or database connections, improving code readability and maintainability.
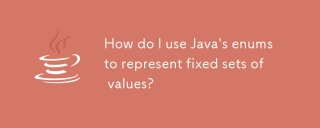
Java enums represent fixed sets of values, offering type safety, readability, and additional functionality through custom methods and constructors. They enhance code organization and can be used in switch statements for efficient value handling.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
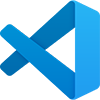
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
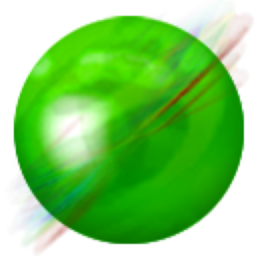
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
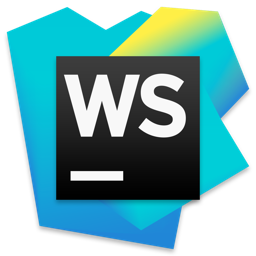
WebStorm Mac version
Useful JavaScript development tools