One of the biggest advantages of Python is its concise syntax. Good code is like pseudocode, clean, tidy, and clear at a glance. To write Pythonic (elegant, authentic, and clean) code, you need to read and learn more code written by experts. There are many excellent source codes on github worth reading, such as: requests, flask, tornado, listed below Some common Pythonic writing methods.
Related learning recommendations: python video tutorial
0. The program must be read by humans before it can be executed by the computer.
“Programs must be written for people to read, and only incidentally for machines to execute.”
1. Exchange assignment
##不推荐 temp = a a = b b = a ##推荐 a, b = b, a # 先生成一个元组(tuple)对象,然后unpack
2. Unpacking
##不推荐 l = ['David', 'Pythonista', '+1-514-555-1234'] first_name = l[0] last_name = l[1] phone_number = l[2] ##推荐 l = ['David', 'Pythonista', '+1-514-555-1234'] first_name, last_name, phone_number = l # Python 3 Only first, *middle, last = another_list
3. Using operators in
##不推荐 if fruit == "apple" or fruit == "orange" or fruit == "berry": # 多次判断 ##推荐 if fruit in ["apple", "orange", "berry"]: # 使用 in 更加简洁
4. String operations
##不推荐 colors = ['red', 'blue', 'green', 'yellow'] result = '' for s in colors: result += s # 每次赋值都丢弃以前的字符串对象, 生成一个新对象 ##推荐 colors = ['red', 'blue', 'green', 'yellow'] result = ''.join(colors) # 没有额外的内存分配
5. Dictionary key value list
##不推荐 for key in my_dict.keys(): # my_dict[key] ... ##推荐 for key in my_dict: # my_dict[key] ... # 只有当循环中需要更改key值的情况下,我们需要使用 my_dict.keys() # 生成静态的键值列表。
6. Dictionary key value judgment
##不推荐 if my_dict.has_key(key): # ...do something with d[key] ##推荐 if key in my_dict: # ...do something with d[key]
7. Dictionary get and setdefault method
##不推荐 navs = {} for (portfolio, equity, position) in data: if portfolio not in navs: navs[portfolio] = 0 navs[portfolio] += position * prices[equity] ##推荐 navs = {} for (portfolio, equity, position) in data: # 使用 get 方法 navs[portfolio] = navs.get(portfolio, 0) + position * prices[equity] # 或者使用 setdefault 方法 navs.setdefault(portfolio, 0) navs[portfolio] += position * prices[equity]
8. Determine authenticity
##不推荐 if x == True: # .... if len(items) != 0: # ... if items != []: # ... ##推荐 if x: # .... if items: # ...
9. Traverse the list and index
##不推荐 items = 'zero one two three'.split() # method 1 i = 0 for item in items: print i, item i += 1 # method 2 for i in range(len(items)): print i, items[i] ##推荐 items = 'zero one two three'.split() for i, item in enumerate(items): print i, item
10. List comprehension
##不推荐 new_list = [] for item in a_list: if condition(item): new_list.append(fn(item)) ##推荐 new_list = [fn(item) for item in a_list if condition(item)]
11. List comprehension-nested
##不推荐 for sub_list in nested_list: if list_condition(sub_list): for item in sub_list: if item_condition(item): # do something... ##推荐 gen = (item for sl in nested_list if list_condition(sl) \ for item in sl if item_condition(item)) for item in gen: # do something...
12. Loop nesting
##不推荐 for x in x_list: for y in y_list: for z in z_list: # do something for x & y ##推荐 from itertools import product for x, y, z in product(x_list, y_list, z_list): # do something for x, y, z
13. Try to use generators instead of lists
##不推荐 def my_range(n): i = 0 result = [] while i < n: result.append(fn(i)) i += 1 return result # 返回列表 ##推荐 def my_range(n): i = 0 result = [] while i < n: yield fn(i) # 使用生成器代替列表 i += 1 *尽量用生成器代替列表,除非必须用到列表特有的函数。
14. Try to use imap/ifilter instead of map/filter for intermediate results
##不推荐 reduce(rf, filter(ff, map(mf, a_list))) ##推荐 from itertools import ifilter, imap reduce(rf, ifilter(ff, imap(mf, a_list))) *lazy evaluation 会带来更高的内存使用效率,特别是当处理大数据操作的时候。
15. Use any/all function
##不推荐 found = False for item in a_list: if condition(item): found = True break if found: # do something if found... ##推荐 if any(condition(item) for item in a_list): # do something if found...
16. Properties
##不推荐 class Clock(object): def __init__(self): self.__hour = 1 def setHour(self, hour): if 25 > hour > 0: self.__hour = hour else: raise BadHourException def getHour(self): return self.__hour ##推荐 class Clock(object): def __init__(self): self.__hour = 1 def __setHour(self, hour): if 25 > hour > 0: self.__hour = hour else: raise BadHourException def __getHour(self): return self.__hour hour = property(__getHour, __setHour)
17. Use with to process file opening
##不推荐 f = open("some_file.txt") try: data = f.read() # 其他文件操作.. finally: f.close() ##推荐 with open("some_file.txt") as f: data = f.read() # 其他文件操作...
18. Use with to ignore exceptions (Python 3 only)
##不推荐 try: os.remove("somefile.txt") except OSError: pass ##推荐 from contextlib import ignored # Python 3 only with ignored(OSError): os.remove("somefile.txt")
19. Use with to handle locking
##不推荐 import threading lock = threading.Lock() lock.acquire() try: # 互斥操作... finally: lock.release() ##推荐 import threading lock = threading.Lock() with lock: # 互斥操作...
Related recommendations:Programming video course
The above is the detailed content of Master python 19 programming skills worth learning. For more information, please follow other related articles on the PHP Chinese website!
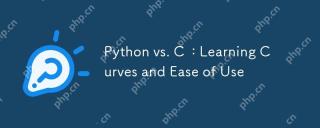
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
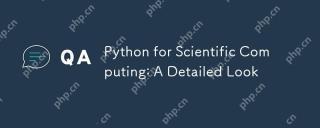
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
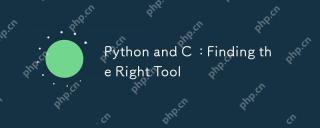
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
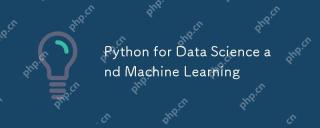
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
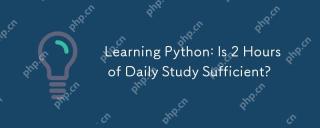
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
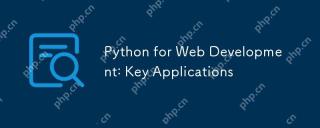
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
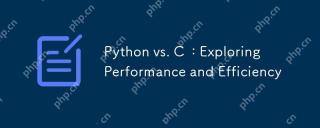
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
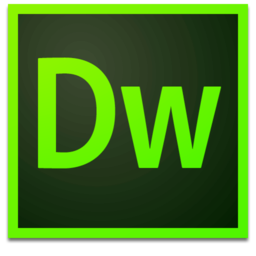
Dreamweaver Mac version
Visual web development tools
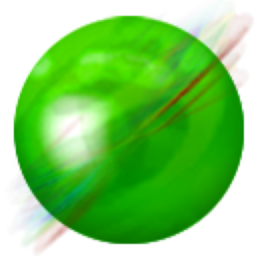
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
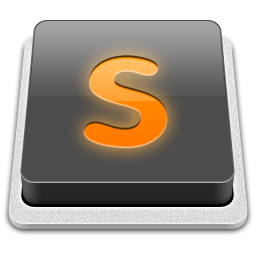
SublimeText3 Mac version
God-level code editing software (SublimeText3)