It is important to navigate between the code you want to inspect. It would be tedious and unnecessary to go through every line of code. The debugger provides a convenient way to see what's important and jump out of unimportant blocks of code. Let's see how to enter, skip, and exit functions while debugging!
In Previous article, we studied the VS Code debugger, added breakpoints in the code, and also viewed the local status.
This time, we'll learn how to step through code line by line and how to jump in and out of function calls.
Get the code
First, let’s make the last server modification more complex. Add two extra functions: one to get the name from the request and another to generate the greeting.
You can paste the following code into index.js
.
const http = require('http'); const url = require('url'); const hostname = '127.0.0.1'; const port = 3456; const serverUrl = `http://${hostname}:${port}` const getNameFromReq = (req) => { const {name} = url.parse(req.url, true).query; return name } const getGreeting = (name) => { const greeting = `Hello, ${name}!` return greeting } const server = http.createServer((req, res) => { const name = getNameFromReq(req) const greeting = getGreeting(name) res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end(`${greeting}\n`); }); server.listen(port, hostname, () => { console.log(`Server running at ${serverUrl}`); });
The code of this series can be obtained at https://github.com/thekarel/debug-anything
Start the debugger
Let's start the debugger: Use the debug toolbar or press F5
and select Node.js
:
You should be able to access http://127.0.0.1:3456/?name=Coco
and See the greeting.
If you like the command line, you can also use curl http://127.0.0.1:3456\?name\=Coco
to access.
Okay, now that the server is up and running, let's add a breakpoint. The debugger will not start without a breakpoint:
Add a breakpoint on line 21:
const name = getNameFromReq(req)
Step by Step debugging
Trigger the pair againhttp://127.0.0.1: 3456/?name=Coco
, the debugger will be activated and stop at line 21 of the code:
##pretty! Now let's focus on the
Debug Toolbar:
still execute all code as usual. The debugger won't make you bored, and you'll be able to complete your main work faster.
Continue
Continue will run the code until the next breakpoint or the end of the program. One way to debug is to add multiple breakpoints on the relevant lines beforehand and jump between them with continue:Step Over
You can think of Step Over as stepping through the function line by line, but without entering the function call. Use it if you are not interested in the internal logic of the function call in the current line, but just want to see how local variables change over time, for example:declarative code.
Step Into
When a line calls a function that interests you and you want to dig deeper, you can use Step Into. Once inside the code block, you can debug as usual (using continue, step, etc.).
Observe how we skip getNameFromReq
and then enter getGreeting
:
Step Out
Step Out is the opposite of Step In: if you are no longer interested in a function, you can leave it. Using "Step out" will run the rest of the function's code in one go.
Check the difference between these two functions through debugging. We execute the first function line by line, but exit the second function early:
Now, you should have a better understanding of the debugger toolbar, how to focus on the important things and skip the irrelevant parts. Not only will these commands save you time, they will make the entire debugging job more enjoyable! Why not try it in your project?
VSCode debugging tutorial series:
English original address: https://charlesagile.com/debug-javascript-typescript-debugger-navigating-with-steps
Author: Charles Szilagyi
Recommended related tutorials: vscode introductory tutorial
The above is the detailed content of VSCode debugging tutorial (2): step-by-step debugging. For more information, please follow other related articles on the PHP Chinese website!

The difference between VisualStudioProfessional and Enterprise is in the functionality and target user groups. The Professional version is suitable for professional developers and provides functions such as code analysis; the Enterprise version is for large teams and has added advanced tools such as test management.

VisualStudio is suitable for large projects, VSCode is suitable for projects of all sizes. 1. VisualStudio provides comprehensive IDE functions, supports multiple languages, integrated debugging and testing tools. 2.VSCode is a lightweight editor that supports multiple languages through extension, has a simple interface and fast startup.

VisualStudio is a powerful IDE developed by Microsoft, supporting multiple programming languages and platforms. Its core advantages include: 1. Intelligent code prompts and debugging functions, 2. Integrated development, debugging, testing and version control, 3. Extended functions through plug-ins, 4. Provide performance optimization and best practice tools to help developers improve efficiency and code quality.

The differences in pricing, licensing and availability of VisualStudio and VSCode are as follows: 1. Pricing: VSCode is completely free, while VisualStudio offers free community and paid enterprise versions. 2. License: VSCode uses a flexible MIT license, and the license of VisualStudio varies according to the version. 3. Usability: VSCode is supported across platforms, while VisualStudio performs best on Windows.

VisualStudio supports the entire process from code writing to production deployment. 1) Code writing: Provides intelligent code completion and reconstruction functions. 2) Debugging and testing: Integrate powerful debugging tools and unit testing framework. 3) Version control: seamlessly integrate with Git to simplify code management. 4) Deployment and Release: Supports multiple deployment options to simplify the application release process.

VisualStudio offers three license types: Community, Professional and Enterprise. The Community Edition is free, suitable for individual developers and small teams; the Professional Edition is annually subscribed, suitable for professional developers who need more functions; the Enterprise Edition is the highest price, suitable for large teams and enterprises. When selecting a license, project size, budget and teamwork needs should be considered.

VisualStudio is suitable for large-scale project development, while VSCode is suitable for projects of all sizes. 1. VisualStudio provides comprehensive development tools, such as integrated debugger, version control and testing tools. 2.VSCode is known for its scalability, cross-platform and fast launch, and is suitable for fast editing and small project development.

VisualStudio is suitable for large projects and Windows development, while VSCode is suitable for cross-platform and small projects. 1. VisualStudio provides a full-featured IDE, supports .NET framework and powerful debugging tools. 2.VSCode is a lightweight editor that emphasizes flexibility and extensibility, and is suitable for various development scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
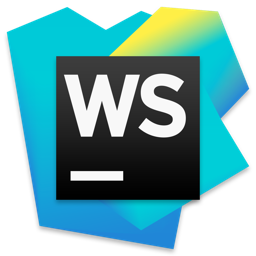
WebStorm Mac version
Useful JavaScript development tools
