In the normal development process, communication between father-son/sibling components is definitely encountered, so here is a summary of the communication props of 6 Vue components / $e$emit / Vuex$attrs / $ listeners
$parent / $children with ref
provide / inject
##Preface
1. props / $emit
This is one of the methods we usually use more often. Parent component A passes data to child component B through the props parameter. Component B sends an event (carrying parameter data) to component A through $emit. Component A listens to the event triggered by $emit and obtains the data sent by B to A. Let’s explain its implementation steps in detail:1: Parent component passes value to child component // App.vue 父组件
<template>
<a-compontent></a-compontent>
</template>
<script>
import aCompontent from './components/A.vue';
export default {
name: 'app',
compontent: { aCompontent },
data () {
return {
dataA: 'dataA数据'
}
}
}
// aCompontent 子组件
<template>
<p>{{dataA}} // 在子组件中把父组件传递过来的值显示出来
<script>export default {
name: 'aCompontent',
props: {
dataA: {
//这个就是父组件中子标签自定义名字
type: String,
required: true // 或者false
}
}
}
</script>
2: Child component passes value to parent component (Through events) // 子组件
<template>
<p>点击向父组件传递数据</p>
</template>
<script>export default {
name: 'child',
methods:{
changeTitle() {
// 自定义事件,会触发父组件的监听事件,并将数据以参数的形式传递
this.$emit('sendDataToParent','这是子组件向父组件传递的数据');
}
}
}
// 父组件
<template>
<child @sendDataToParent="getChildData">
<script>
import child from './components/child.vue';
export default {
name: 'child',
methods:{
getChildData(data) {
console.log(data); // 这里的得到了子组件的值
}
}
}
</script>
2. $emit / $on
This method uses an instance similar to App.vue as a module Event Center, use it to trigger and listen for events. If you put it in App.vue, you can implement communication in any component. However, this method is not easy to maintain when the project is relatively large. For example: Suppose there are 4 components now, Home.vue and A/B/C components. These three components AB are sibling components. Home.vue is equivalent to the parent component creating an empty Vue instance. Mount the communication event on the instance -D.js import Vue from 'vue'export default new Vue()
// 我们可以在router-view中监听change事件,也可以在mounted方法中监听 // home.vue<template> <p> <child-a></child-a> <child-b></child-b> <child-c></child-c> </p></template>
// A组件 <template> <p>将A组件的数据发送给C组件 - {{name}}</p> </template> <script> import Event from "./D";export default { data() { return { name: 'Echo' } }, components: { Event }, methods: { dataA() { Event.$emit('data-a', this.name); } } } </script>
// B组件 <template> <p>将B组件的数据发送给C组件 - {{age}}</p> </template> <script> import Event from "./D";export default { data() { return { age: '18' } }, components: { Event }, methods: { dataB() { Event.$emit('data-b', this.age); } } } </script>
// C组件 <template> <p>C组件得到的数据 {{name}} {{age}}</p> </template> <script> import Event from "./D";export default { data() { return { name: '', age: '' } }, components: { Event }, mounted() { // 在模板编译完成后执行 Event.$on('data-a', name => { this.name = name; }) Event.$on('data-b', age => { this.age = age; }) } } </script>We can know from the above that the $emit event of A/B is monitored in the mounted event of the C component and the parameters passed by it are obtained ( Since we are not sure when the event is triggered, we usually listen in mounted / created)
3. Vuex
Vuex is a state management mode. It uses centralized storage to manage the state of all components of the application, and uses corresponding rules to ensure that the state changes in a predictable way. The core of the Vuex application is the store (warehouse, a container). The store contains most of the state in your application; This part will not be introduced in detail, the official document is very detailed vuex.vuejs .org/zh/guide/st…Four. $attrs / $listeners
- Use Vuex for data management, but the problem with using vuex is that if the project is relatively small and there is less shared state between components, then use vuex is like killing a chicken with a knife.
- Use B component as a transfer station. When A component needs to pass information to C component, B accepts the information of A component and then uses props to pass it to C component. However, if there are too many nested components, it will The code is cumbersome and code maintenance is difficult; if the change of state in C needs to be passed to A, the event system must be used to pass it up level by level.
// App.vue <template> <p> </p> <p>App.vue</p> <hr> // 这里我们可以看到,app.vue向下一集的child1组件传递了5个参数,分别是name / age / job / sayHi / title <child1></child1> </template> <script> const child1 = () => import("./components/child1.vue"); export default { name: 'app', components: { child1 }, data() { return { name: "Echo", age: "18", job: "FE", say: "this is Hi~" }; } }; </script>
// child1.vue <template> <p> </p> <p>child1.vue</p> <p>name: {{ name }}</p> <p>childCom1的$attrs: {{ $attrs }}</p> <p>可以看到,$attrs这个对象集合中的值 = 所有传值过来的参数 - props中显示定义的参数</p> <hr> <child2></child2> </template> <script> const child2 = () => import("./child2.vue"); export default { components: { child2 }, // 这个inheritAttrs默认值为true,不定义这个参数值就是true,可手动设置为false // inheritAttrs的意义在用,可以在从父组件获得参数的子组件根节点上,将所有的$attrs以dom属性的方式显示 inheritAttrs: true, // 可以关闭自动挂载到组件根元素上的没有在props声明的属性 props: { name: String // name作为props属性绑定 }, created() { // 这里的$attrs就是所有从父组件传递过来的所有参数 然后 除去props中显式定义的参数后剩下的所有参数!!! console.log(this.$attrs); // 输出{age: "18", job: "FE", say-Hi: "this is Hi~", title: "App.vue的title"} } }; </script>
// child2.vue <template> <p> </p> <p>child2.vue</p> <p>age: {{ age }}</p> <p>childCom2: {{ $attrs }}</p> <hr> <child3></child3> </template> <script> const child3 = () => import("./child3.vue"); export default { components: { child3 }, // 将inheritAttrs设置为false之后,将关闭自动挂载到组件根元素上的没有在props声明的属性 inheritAttrs: false, props: { age: String }, created() { // 同理和上面一样,$attrs这个对象集合中的值 = 所有传值过来的参数 - props中显示定义的参数 console.log(this.$attrs); } }; </script>
// child3.vue <template> <p> </p> <p>child3.vue</p> <p>job: {{job}}</p> <p>title: {{title}}</p> <p>childCom3: {{ $attrs }}</p> </template> <script>export default { inheritAttrs: true, props: { job: String, title: String } }; </script>Let’s take a look at the specific display effect:
<template> <p> <child2></child2> </p> </template> <script> const child2 = () => import("./child2.vue"); export default { components: { child2 }, methods: { reciveRocket() { console.log("reciveRocket success"); } } }; </script>复制代码
<template> <p> <child3></child3> </p> </template> <script> const child3 = () => import("./child3.vue"); export default { components: { child3 }, created() { this.$emit('child2', 'child2-data'); } }; </script>复制代码
// child3.vue <template> <p> </p> <p>child3</p> </template> <script> export default { methods: { startUpRocket() { this.$emit("upRocket"); console.log("startUpRocket"); } } }; </script>复制代码
这里的结果是,当我们点击 child3 组件的 child3 文字,触发 startUpRocket 事件,child1 组件就可以接收到,并触发 reciveRocket 打印结果如下:
> reciveRocket success > startUpRocket
五. $parent / $children 与 ref
- ref:如果在普通的 DOM 元素上使用,引用指向的就是 DOM 元素;如果用在子组件上,引用就指向组件实例
- $parent / $children:访问父 / 子实例
这两种方式都是直接得到组件实例,使用后可以直接调用组件的方法或访问数据。
我们先来看个用 ref 来访问组件的:
// child1子组件 export default { data() { return { title: 'Vue.js' }; }, methods: { sayHello() { console.log('child1!!'); } } };
// 父组件 <template> <child1></child1> </template> <script> export default { methods: { sayHi () { const child1 = this.$refs.child1; console.log(child1.title); // Vue.js child1.sayHello(); // 弹窗 } } } </script>
六. provide/inject
provide/inject 是 Vue2.2.0 新增 API,这对选项需要一起使用,以允许一个祖先组件向其所有子孙后代注入一个依赖,不论组件层次有多深,并在起上下游关系成立的时间里始终生效。如果你熟悉 React,这与 React 的上下文特性很相似。
provide 和 inject 主要为高阶插件/组件库提供用例。并不推荐直接用于应用程序代码中。
由于自己对这部分的内容理解不是很深刻,所以感兴趣的可以前往官方文档查看: cn.vuejs.org/v2/api/#pro…
总结
常见使用场景可以分为三类:
- 父子通信:props / $emit;$parent / $children;$attrs/$listeners;provide / inject API; ref
- 兄弟通信:Vuex
- 跨级通信:Vuex;$attrs/$listeners;provide / inject API
4.接下来我还会在我的裙里用视频讲解的方式给大家讲解【以下图中的内容】有兴趣的可以来我的扣扣裙 519293536 免费交流学习,我都会尽力帮大家哦
推荐教程:《JS教程》
The above is the detailed content of Six ways to communicate with Vue components. For more information, please follow other related articles on the PHP Chinese website!
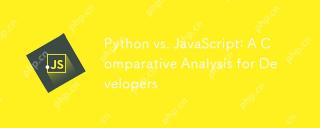
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
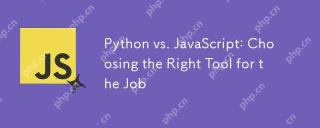
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
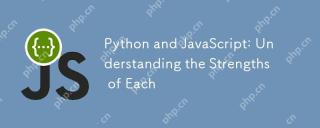
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
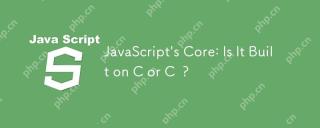
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
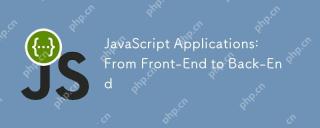
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
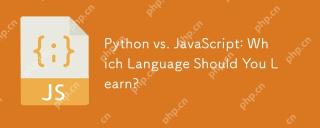
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
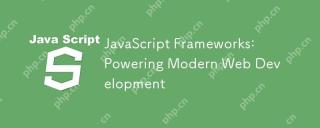
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
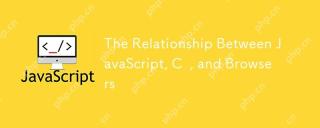
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
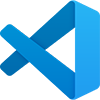
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
