Three commonly used injection methods in Spring
1. Construction method, using reflection, completes injection through the construction method.
Let’s take a brief look at the structure of the test project. It is built with maven and has four packages:
entity: storage entity, with only one User class in it
dao: data access, one interface, two implementation classes
service: service layer, one interface, one implementation class, the implementation class depends on IUserDao
test: Test package
Register UserService in the spring configuration file, and inject UserDaoJdbc into a userService through the constructor-arg tag. Parameter constructor
<!-- 注册userService --> <bean id="userService" class="com.lyu.spring.service.impl.UserService"> <constructor-arg ref="userDaoJdbc"></constructor-arg> </bean> <!-- 注册jdbc实现的dao --> <bean id="userDaoJdbc" class="com.lyu.spring.dao.impl.UserDaoJdbc"></bean>
If there is only one constructor with parameters and the parameter type matches the type of the injected bean, it will be injected into the constructor.
public class UserService implements IUserService { private IUserDao userDao; public UserService(IUserDao userDao) { this.userDao = userDao; } public void loginUser() { userDao.loginUser(); } } @Test public void testDI() { ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml"); // 获取bean对象 UserService userService = ac.getBean(UserService.class, "userService"); // 模拟用户登录 userService.loginUser(); }
Test print result: jdbc-login successful
Note: The loginUser method that simulates user login actually just prints an output statement. The output of the class implemented by jdbc is: jdbc- The login is successful. The output of the class implemented by mybatis is: mybatis-login successful.
2. Setter also uses reflection, but the injection is completed through setter.
The configuration file is as follows:
<!-- 注册userService --> <bean id="userService" class="com.lyu.spring.service.impl.UserService"> <!-- 写法一 --> <!-- <property name="UserDao" ref="userDaoMyBatis"></property> --> <!-- 写法二 --> <property name="userDao" ref="userDaoMyBatis"></property> </bean> <!-- 注册mybatis实现的dao --> <bean id="userDaoMyBatis" class="com.lyu.spring.dao.impl.UserDaoMyBatis"></bean>
Note: Both of the above writing methods are acceptable. Spring will convert the first letter of each word in the name value to uppercase, and then splice it in front. Use "set" to form a method name, then search for the method in the corresponding class, and call it through reflection to achieve injection.
Remember: the name attribute value has nothing to do with the member variable name in the class or the parameter name of the set method. It is only related to the corresponding set method name. The following writing method can run successfully
public class UserService implements IUserService { private IUserDao userDao1; public void setUserDao(IUserDao userDao1) { this.userDao1 = userDao1; } public void loginUser() { userDao1.loginUser(); } }
One more thing to note: If you inject properties through the set method, spring will instantiate the object through the default empty parameter constructor, so if you write a constructor with parameters in the class, it must The constructor method with empty parameters must be written, otherwise spring will not be able to instantiate the object, resulting in an error.
3. Based on annotations, commonly used ones are "@Autowried" and "@Resource".
Sample code
// candidateBeans 为上一步通过类型匹配到的多个bean,该 Map 中至少有两个元素。 protected String determineAutowireCandidate(Map<String, Object> candidateBeans, DependencyDescriptor descriptor) { // requiredType 为匹配到的接口的类型 Class<?> requiredType = descriptor.getDependencyType(); // 1. 先找 Bean 上有@Primary 注解的,有则直接返回 String primaryCandidate = this.determinePrimaryCandidate(candidateBeans, requiredType); if (primaryCandidate != null) { return primaryCandidate; } else { // 2.再找 Bean 上有 @Order,@PriorityOrder 注解的,有则返回 String priorityCandidate = this.determineHighestPriorityCandidate(candidateBeans, requiredType); if (priorityCandidate != null) { return priorityCandidate; } else { Iterator var6 = candidateBeans.entrySet().iterator(); String candidateBeanName; Object beanInstance; do { if (!var6.hasNext()) { return null; } // 3. 再找 bean 的名称匹配的 Entry<String, Object> entry = (Entry)var6.next(); candidateBeanName = (String)entry.getKey(); beanInstance = entry.getValue(); } while(!this.resolvableDependencies.values().contains(beanInstance) && !this.matchesBeanName(candidateBeanName, descriptor.getDependencyName())); return candidateBeanName; } } }
Recommended tutorial: "Java Tutorial"
The above is the detailed content of Three commonly used injection methods in Spring. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
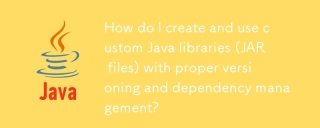
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
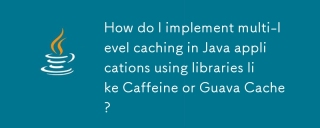
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
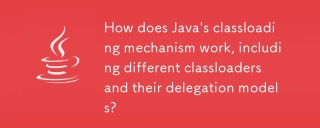
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
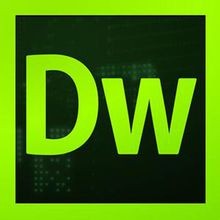
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
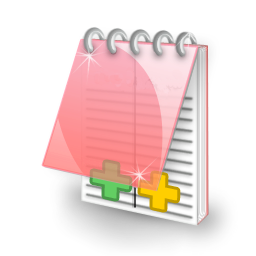
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.