Immutability of objects means that we do not want the objects to change in any way after they are created (setting them to a read-only type).
Suppose we need to define a car object and use its properties throughout the project to perform operations. We cannot allow any data to be modified incorrectly.
const myTesla = { maxSpeed: 155, batteryLife: 300, weight: 2300 };
Object.preventExtensions() Prevent extension
This method prevents adding new properties to existing objects, preventExtensions() is an irreversible operation, we can never Add additional properties to the object.
Object.isExtensible(myTesla); // true Object.preventExtensions(myTesla); Object.isExtensible(myTesla); // false myTesla.color = 'blue'; console.log(myTesla.color) // undefined
Object.seal() seal
It can prevent the addition or deletion of attributes. seal() can also prevent the modification of the attribute descriptor.
Object.isSealed(myTesla); // false Object.seal(myTesla); Object.isSealed(myTesla); // true myTesla.color = 'blue'; console.log(myTesla.color); // undefined delete myTesla.batteryLife; // false console.log(myTesla.batteryLife); // 300 Object.defineProperty(myTesla, 'batteryLife'); // TypeError: Cannot redefine property: batteryLife
Object.freeze() Freeze
It has the same effect as Object.seal(), and it makes the property unwritable.
Object.isFrozen(myTesla); // false Object.freeze(myTesla); Object.isFrozen(myTesla); // true myTesla.color = 'blue'; console.log(myTesla.color); // undefined delete myTesla.batteryLife; console.log(myTesla.batteryLife); // 300 Object.defineProperty(myTesla, 'batteryLife'); // TypeError: Cannot redefine property: batteryLife myTesla.batteryLife = 400; console.log(myTesla.batteryLife); // 300
Note: If you want an error to be thrown when trying to modify an immutable object, use strict mode.
Recommended tutorial: js introductory tutorial
The above is the detailed content of How to create immutable objects in js. For more information, please follow other related articles on the PHP Chinese website!
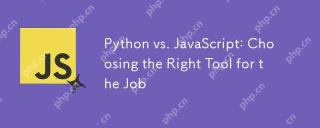
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
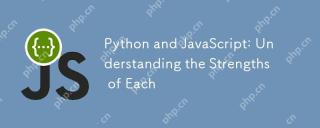
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
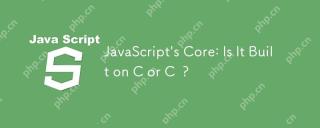
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
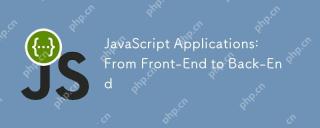
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
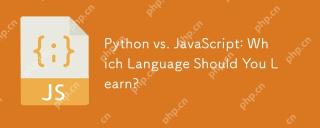
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
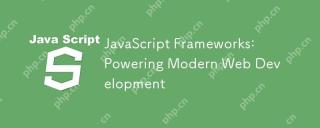
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
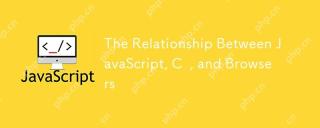
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
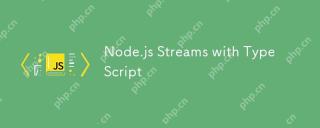
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
