


9 powerful mainstream writing methods in JS (various Hack writing methods)
1. Global replacement
We know that the string function replace () only replaces the first occurrence Condition.
You can replace all occurrences by adding /g at the end of the regular expression.
var example = "potato potato"; console.log(example.replace(/pot/, "tom")); // "tomato potato" console.log(example.replace(/pot/g, "tom")); // "tomato tomato"
2. Extract unique values
By using the Set object and the spread operator, we can create a new array with only unique values.
var entries = [1, 2, 2, 3, 4, 5, 6, 6, 7, 7, 8, 4, 2, 1] var unique_entries = [...new Set(entries)]; console.log(unique_entries); // [1, 2, 3, 4, 5, 6, 7, 8]
3. Convert number to string
We just need to connect a set of empty quotes.
var converted_number = 5 + ""; console.log(converted_number); // 5 console.log(typeof converted_number); // string
4. Convert string to number
All we need is operator.
One thing to note is that it only applies to "string numbers".
the_string = "123"; console.log(+the_string); // 123 the_string = "hello"; console.log(+the_string); // NaN
5. Randomly arrange the elements in the array
I shuffle the cards every day
var my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]; console.log(my_list.sort(function() { return Math.random() - 0.5 })); // [4, 8, 2, 9, 1, 3, 6, 5, 7]
6. Multidimensional array flattening
Just use the spread operator.
var entries = [1, [2, 5], [6, 7], 9]; var flat_entries = [].concat(...entries); // [1, 2, 5, 6, 7, 9]
7. Short Circuit Condition
Let’s look at this example:
if (available) { addToCart(); }
Just use a variable with a function to shorten it :
available && addToCart()
8. Dynamic property names
I always thought that I must first declare an object before assigning dynamic properties.
const dynamic = 'flavour'; var item = { name: 'Coke', [dynamic]: 'Cherry' } console.log(item); // { name: "Coke", flavour: "Cherry" }
9. Use length to adjust or clear an array
We mainly rewrite the length of the array.
If we want to adjust the size of the array:
var entries = [1, 2, 3, 4, 5, 6, 7]; console.log(entries.length); // 7 entries.length = 4; console.log(entries.length); // 4 console.log(entries); // [1, 2, 3, 4]
If we want an empty array:
var entries = [1, 2, 3, 4, 5, 6, 7]; console.log(entries.length); // 7 entries.length = 0; console.log(entries.length); // 0 console.log(entries); // []
Recommended tutorial: "JS Tutorial"
The above is the detailed content of 9 powerful mainstream writing methods in JS (various Hack writing methods). For more information, please follow other related articles on the PHP Chinese website!
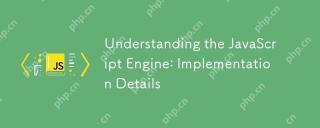
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
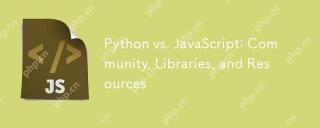
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
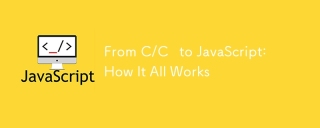
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
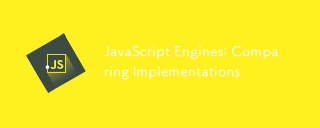
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
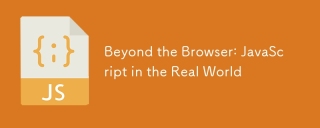
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
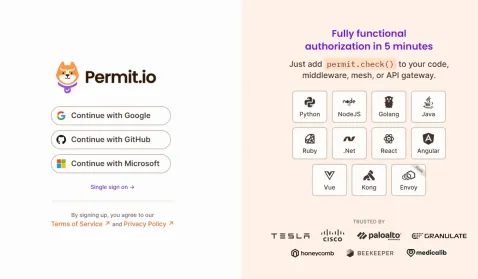
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
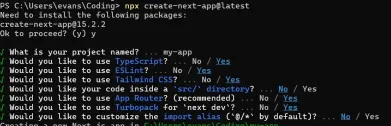
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool