Detailed introduction to the variable promotion mechanism in js
Variable promotion
There are two types of variable promotion in JavaScript, variables declared with var and variables declared with function.
Variables declared with var
Let’s first look at the following code, what is the value of a
Code 1
console.log(a); var a;
According to From the perspective of the past programming language thinking, the code runs from top to bottom. According to this thinking, an error will be reported. Because when the execution reaches the second line, the variable a has not been defined, so the error a is not defined
will be reported. However, in fact the answer is undefined
Okay, with doubts, let’s look at the following code
var a; console.log(a);
We found that the two pieces of code are the same, so there is a new question, which is It doesn't matter whether there is var a or not. Its answer is always undefined, which creates the illusion that the variable will be improved, so I wrote code 3
code 3
console.log(a);
Okay, it finally An error was reported, so this proves that the javaScript code is not executed from top to bottom, at least on the surface it seems like this.
So let’s look at code 4
Code 4
console.log(a); var a = 2;
Because the variable is promoted, the answer is 2, but in fact, it is still undefined, why?
At this time we have to ask the compiler, who is responsible for the dirty work such as syntax analysis and code generation.
When the compiler sees var a = 2;, it will treat it as two declarations, var a; and a = 2. The first declaration will be made during the compilation phase, and the second declaration will be Waiting in place for the execution phase.
That is to say, the above code will become the following code
var a; console.log(a); a = 2;
So it will end up being undefined
Okay, let me be verbose, look at this code 5
Code 5
a = 2; var a; console.log(a);
I think everyone should already know the actual order of execution of this code and its answer. Yes, the answer is 2, but what I want to say is to change the 2nd line is commented out, the answer is still 2, but this has nothing to do with variable promotion. It is a matter of strict mode and non-strict mode. In non-strict mode, developers are allowed not to use keywords to declare variables, but in strict mode This is not possible in mode, it will report an error.
Variables declared with function
Like var, variables declared by function will still be promoted.
log(5); function log(mes){ console.log(mes) }
According to the previous understanding of variable promotion, the real sequence of this code is this,
function log(mes){ console.log(mes) } log(5);
Very good, very correct, then look at the next code
log(5); var log = function(mes){ console.log(mes) }
It reported an error, log is not a function. From here we can see that this kind of function expression will not be promoted. Only function declarations will be promoted. Try adding a line of code console.log at the front. (log), undefined will be output first.
So the real order here is
var log; log(); //这时候只是声明了log这个变量,并不是函数,却用函数的方法调用它,所以会报错,说这不是一个函数。 log = function(mes){ console.log(mes) }
Use var to declare variables in function
Although we know that variables declared with var will be promoted, we don’t know To what extent will it be improved?
Let’s look at a piece of code before this
var a = 4; function foo(){ var a = 5; console.log(a); } foo(); console.log(a)
The answer is 5,4, output 5 first, and then output 4.
Variables declared with var have function scope, so a in foo has no relationship with a outside foo. This situation is exactly what I want.
Change the code again
function foo(){ a = 5 console.log(a); var a; } foo(); console.log(a)
The answer is 5, a is not defined
The 4th line of code outputs 5, and the 9th line reports an error.
In this case, variable promotion will only be promoted to the top of the scope where the variable is located, and will not be promoted to the parent scope.
So we can draw a conclusion: variable promotion will only promote the variable to the top of its own scope
Function priority
Since using var and function The variables have the function of promotion, so what will happen if the same variable is declared with both of them? Well, just look at the title and you will know that the function takes precedence.
Look at the code in detail
foo(); var foo; function foo(){ console.log(1) } foo = function(){ console.log(2) }
The answer is 1
This code actually looks like this
function foo(){ console.log(1) } foo();// 1 foo = function(){ console.log(2) }
Look carefully, var foo; is gone, Yep, it was ignored by the engine, which considered it a duplicate declaration and threw it away.
Okay, since variables declared by var are not as good as function declarations, then use function declarations to declare the same variable multiple times.
foo() function foo(){ console.log(1); } foo() function foo(){ console.log(2); } foo() function foo(){ console.log(3); } foo()
foo is declared three times and called four times. The result of each call is 3, so the final function declaration will overwrite the previous function declaration
But var still wants to struggle, I still feel it is necessary to prove my sense of existence.
foo() function foo(){ console.log(1); } var foo; foo() foo = function(){ console.log(2); } foo() function foo(){ console.log(3); } foo()
Look carefully, the middle part of the code has been changed, outputting 3,3,2,2 in sequence
Although var foo is ignored, the following function is still useful, this code It can be seen as like this
function foo(){ console.log(3); } foo();//3 foo();//3 foo = function(){ console.log(2); } foo();//2 foo();//2
Declare the function inside the ordinary block
Before, the function was declared in the scope, now declare the function inside the block
function foo(){ console.log(b); // undefined b(); //TypeError: b is not a function var a = true; if(a){ function b(){ console.log(2) } //下面这段代码和上面的结果一样 // var b = function(){ // console.log(2) // } } //b() --> 这里会被执行 } foo()
From From the above, b is undefined, which proves that this variable still exists, but it is not a function. This situation is similar to using a function expression.
Summary
1. Promotion is divided into function declaration promotion and variable declaration promotion
2. Use var to declare variables and function to declare functions
3. Variable promotion will promote the variable to the top of its own scope.
4. There is no promotion mechanism for function expressions.
5. Function declaration and variable declaration declare the same identifier at the same time. symbol, the function declaration takes precedence
6. When multiple functions declare the same identifier, the last declaration overwrites the previous declaration
Recommended tutorial: js tutorial
The above is the detailed content of Detailed introduction to the variable promotion mechanism in js. For more information, please follow other related articles on the PHP Chinese website!
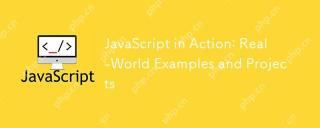
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
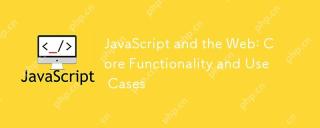
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
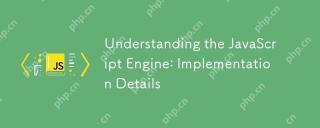
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
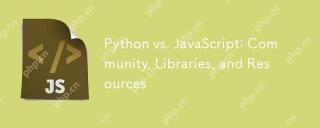
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
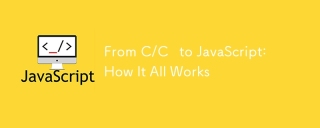
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
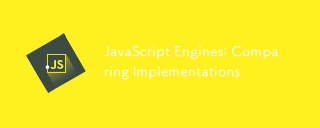
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
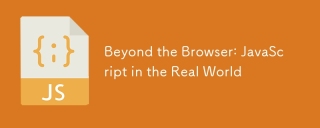
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
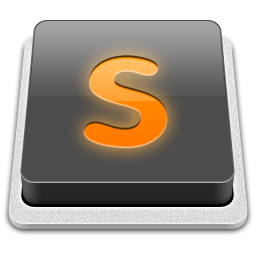
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.