The following column workerman php tutorial will introduce how to use the workerman timer. I hope it will be helpful to friends in need!
Workerman is a high-performance socket server communication framework, used to quickly develop various network applications, including tcp, udp, long connection, and short connection applications. Let's introduce the use of workerman timer.
add
int \Workerman\Lib\Timer::add(float $time_interval, callable $callback [,$args = array(), bool $persistent = true])
Execute a function or class method regularly
Parameters
time_interval: how often to execute, in seconds, supports decimals, yes Accurate to 0.001, that is, accurate to the millisecond level.
callback: callback function Note: If the callback function is a method of the class, the method must be a public attribute
args: the parameters of the callback function must be an array, and the array elements are parameter values
persistent: Whether it is persistent. If you only want to execute it once regularly, pass false (a task that is executed only once will be automatically destroyed after execution, and there is no need to call Timer::del()). The default is true, which means it is always executed regularly.
Return value
Returns an integer representing the timerid of the timer. This timer can be destroyed by calling Timer::del($timerid).
Example
1. The timing function is an anonymous function (closure)
use \Workerman\Worker; use \Workerman\Lib\Timer; require_once './Workerman/Autoloader.php'; $task = new Worker(); // 开启多少个进程运行定时任务,注意多进程并发问题 $task->count = 1; $task->onWorkerStart = function($task) { // 每2.5秒执行一次 $time_interval = 2.5; Timer::add($time_interval, function() { echo "task run\n"; }); }; // 运行worker Worker::runAll();
2. The timing function is an ordinary function
require_once './Workerman/Autoloader.php'; use \Workerman\Worker; use \Workerman\Lib\Timer; // 普通的函数 function send_mail($to, $content) { echo "send mail ...\n"; } $task = new Worker(); $task->onWorkerStart = function($task) { $to = 'workerman@workerman.net'; $content = 'hello workerman'; // 10秒后执行发送邮件任务,最后一个参数传递false,表示只运行一次 Timer::add(10, 'send_mail', array($to, $content), false); }; // 运行worker Worker::runAll();
3. The timing function is Class method
require_once './Workerman/Autoloader.php'; use \Workerman\Worker; use \Workerman\Lib\Timer; class Mail { // 注意,回调函数属性必须是public public function send($to, $content) { echo "send mail ...\n"; } } $task = new Worker(); $task->onWorkerStart = function($task) { // 10秒后发送一次邮件 $mail = new Mail(); $to = 'workerman@workerman.net'; $content = 'hello workerman'; Timer::add(10, array($mail, 'send'), array($to, $content), false); }; // 运行worker Worker::runAll();
4. The timing function is a class method (timer is used inside the class)
require_once './Workerman/Autoloader.php'; use \Workerman\Worker; use \Workerman\Lib\Timer; class Mail { // 注意,回调函数属性必须是public public function send($to, $content) { echo "send mail ...\n"; } public function sendLater($to, $content) { // 回调的方法属于当前的类,则回调数组第一个元素为$this Timer::add(10, array($this, 'send'), array($to, $content), false); } } $task = new Worker(); $task->onWorkerStart = function($task) { // 10秒后发送一次邮件 $mail = new Mail(); $to = 'workerman@workerman.net'; $content = 'hello workerman'; $mail->sendLater($to, $content); }; // 运行worker Worker::runAll();
5. The timing function is a static method of the class
require_once './Workerman/Autoloader.php'; use \Workerman\Worker; use \Workerman\Lib\Timer; class Mail { // 注意这个是静态方法,回调函数属性也必须是public public static function send($to, $content) { echo "send mail ...\n"; } } $task = new Worker(); $task->onWorkerStart = function($task) { // 10秒后发送一次邮件 $to = 'workerman@workerman.net'; $content = 'hello workerman'; // 定时调用类的静态方法 Timer::add(10, array('Mail', 'send'), array($to, $content), false); }; // 运行worker Worker::runAll();
6. Timing The function is a static method of the class (with namespace)
namespace Task; require_once './Workerman/Autoloader.php'; use \Workerman\Worker; use \Workerman\Lib\Timer; class Mail { // 注意这个是静态方法,回调函数属性也必须是public public static function send($to, $content) { echo "send mail ...\n"; } } $task = new Worker(); $task->onWorkerStart = function($task) { // 10秒后发送一次邮件 $to = 'workerman@workerman.net'; $content = 'hello workerman'; // 定时调用带命名空间的类的静态方法 Timer::add(10, array('\Task\Mail', 'send'), array($to, $content), false); }; // 运行worker Worker::runAll();
7. Destroy the current timer in the timer (use closure method to pass $timer_id)
use \Workerman\Worker; use \Workerman\Lib\Timer; require_once './Workerman/Autoloader.php'; $task = new Worker(); $task->onWorkerStart = function($task) { // 计数 $count = 1; // 要想$timer_id能正确传递到回调函数内部,$timer_id前面必须加地址符 & $timer_id = Timer::add(1, function()use(&$timer_id, &$count) { echo "Timer run $count\n"; // 运行10次后销毁当前定时器 if($count++ >= 10) { echo "Timer::del($timer_id)\n"; Timer::del($timer_id); } }); }; // 运行worker Worker::runAll();
8. Destroy the current timer in the timer Timer (pass $timer_id in parameter mode)
require_once './Workerman/Autoloader.php'; use \Workerman\Worker; use \Workerman\Lib\Timer; class Mail { public function send($to, $content, $timer_id) { // 临时给当前对象添加一个count属性,记录定时器运行次数 $this->count = empty($this->count) ? 1 : $this->count; // 运行10次后销毁当前定时器 echo "send mail {$this->count}...\n"; if($this->count++ >= 10) { echo "Timer::del($timer_id)\n"; Timer::del($timer_id); } } } $task = new Worker(); $task->onWorkerStart = function($task) { $mail = new Mail(); // 要想$timer_id能正确传递到回调函数内部,$timer_id前面必须加地址符 & $timer_id = Timer::add(1, array($mail, 'send'), array('to', 'content', &$timer_id)); }; // 运行worker Worker::runAll();
9. Set the timer only in the specified process
A worker instance has 4 processes, and only set it on the process with ID number 0 timer.
use Workerman\Worker; use Workerman\Lib\Timer; require_once './Workerman/Autoloader.php'; $worker = new Worker(); $worker->count = 4; $worker->onWorkerStart = function($worker) { // 只在id编号为0的进程上设置定时器,其它1、2、3号进程不设置定时器 if($worker->id === 0) { Timer::add(1, function(){ echo "4个worker进程,只在0号进程设置定时器\n"; }); } }; // 运行worker Worker::runAll();
For more workerman knowledge, please pay attention to the workerman tutorial column.
The above is the detailed content of Use of worker timer (with sample code). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
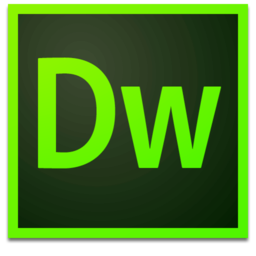
Dreamweaver Mac version
Visual web development tools
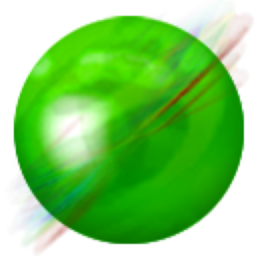
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
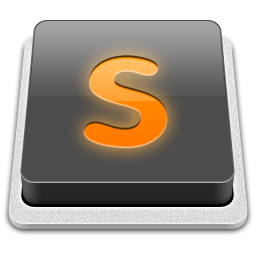
SublimeText3 Mac version
God-level code editing software (SublimeText3)