3 js substring methods to implement string_javascript skills
I recently encountered a question, "How to use JavaScript to implement the substring method of string?" I currently have the following three solutions in mind:
Method 1: Use charAt to extract the intercepted part:
String.prototype.mysubstring=function(beginIndex,endIndex){ var str=this, newArr=[]; if(!endIndex){ endIndex=str.length; } for(var i=beginIndex;i<endIndex;i++){ newArr.push(str.charAt(i)); } return newArr.join(""); } //test "Hello world!".mysubstring(3);//"lo world!" "Hello world!".mysubstring(3,7);//"lo w"
Method 2: Convert the string into an array and take out the required part:
String.prototype.mysubstring=function(beginIndex,endIndex){ var str=this, strArr=str.split(""); if(!endIndex){ endIndex=str.length; } return strArr.slice(beginIndex,endIndex).join(""); } //test console.log("Hello world!".mysubstring(3));//"lo world!" console.log("Hello world!".mysubstring(3,7));//"lo w"
Method 3: Take out the head and tail parts, and then use replace to remove the excess parts. It is suitable for situations where beginIndex is small and string length-endIndex is small:
String.prototype.mysubstring=function(beginIndex,endIndex){ var str=this, beginArr=[], endArr=[]; if(!endIndex){ endIndex=str.length; } for(var i=0;i<beginIndex;i++){ beginArr.push(str.charAt(i)); } for(var i=endIndex;i<str.length;i++){ endArr.push(str.charAt(i)); } return str.replace(beginArr.join(""),"").replace(endArr.join(""),""); } //test console.log("Hello world!".mysubstring(3));//"lo world!" console.log("Hello world!".mysubstring(3,7));//"lo w"
You can try the above three substring methods of string in js and compare which method is more convenient. I hope this article will be helpful to everyone's learning.
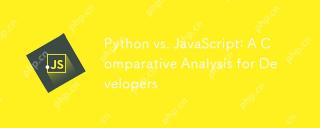
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
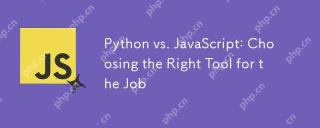
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
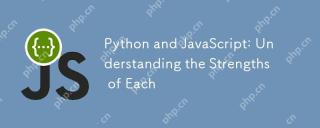
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
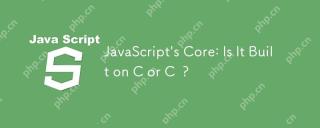
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
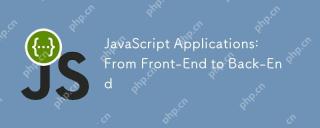
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
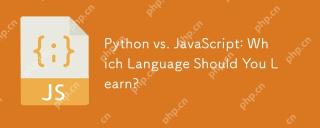
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
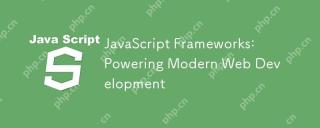
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
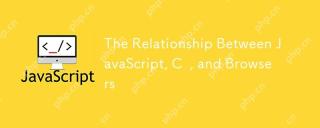
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
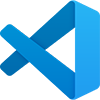
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
