


This article is based on multi-process testing done by pcntl extension.
Process Scheduling Strategy
The operating system is responsible for the scheduling of parent and child processes. The specific scheduling of the child process or the parent process first is determined by the system's scheduling algorithm. Of course, the parent process can be scheduled first. Adding a delay to the process or calling the process recycling function pcntl_wait can let the child process run first. The purpose of process recycling is to release the memory space occupied when the process is created to prevent it from becoming a zombie process.
Signal:
The signal is called the soft interrupt system or soft interrupt. Its function is to send asynchronous event notifications to the process.
Signal number: [The source code is based on SIGINT, SIGTERM, SIGUSR1 signals, please check the kill command manual for the meaning, no description is given]
linux supports 64, half of them are Half of the real-time signals are non-real-time signals. These signals have their own numbers and corresponding integer values. Readers can refer to the relevant Linux manuals for the meaning of each signal number [you can find out by looking at the man manual]
Signal processing function:
Signals are generally bound to the corresponding Functions, some have default actions such as SIGKILL, SIGTERM, SIGINT. The default operation is to kill the process. Of course, we can override it by overriding it through pcntl_signal.
The concept of signal: It is the same as hardware interrupt. Please refer to my previous articles or check the chip hardware interrupt principle.
Signal sending:
kill signal number process or the interrupt signal of the key product or you can use posix_kill and other functions in the source code.
Processes are isolated from each other and have their own stack space. In addition to some common text [code area], they also have their own executable code. When the process is running, it will occupy CPU resources and other processes will not have access to it. Right to run, at this time other processes will be in a blocked state [such as the tcp service mentioned earlier]. When the process ends [run to the last sentence of the code or encounter return or exit, exit the process function or encounter It will exit when a signal event occurs] Give up permissions and release memory, and other processes will have the opportunity to run.
The process has its own process descriptor, of which the process number PID is more commonly used. When the process is running, the corresponding process file will be generated under the system /proc/PID, and the user can view it by himself.
Each process has its own process group [collection of processes]. A collection of multiple process groups is a session. Creating a session is created through a process, and this process cannot be the group leader process. , this process will become the session first process during the session, and will also become the process leader of the process group. At the same time, it will leave the control terminal. Even if the previous process is bound to the control terminal, it will leave [Creation of Daemon Process].
File description permission mask [Permission mask word]:
umask () You can run this command in linux, then create the file and view its permissions [ If you don’t find anything after running, it means you still haven’t trained enough^_^】
<?php /** * Created by PhpStorm. * User: 1655664358@qq.com * Date: 2018/3/26 * Time: 14:19 */ namespace Chen\Worker; class Server { public $workerPids = []; public $workerJob = []; public $master_pid_file = "master_pid"; public $state_file = "state_file.txt"; function run() { $this->daemon(); $this->worker(); $this->setMasterPid(); $this->installSignal(); $this->showState(); $this->wait(); } function wait() { while (1){ pcntl_signal_dispatch(); $pid = pcntl_wait($status); if ($pid>0){ unset($this->workerPids[$pid]); }else{ if (count($this->workerPids)==0){ exit(); } } usleep(100000); } } function showState() { $state = "\nMaster 信息\n"; $state.=str_pad("master pid",25); $state.=str_pad("worker num",25); $state.=str_pad("job pid list",10)."\n"; $state.=str_pad($this->getMasterPid(),25); $state.=str_pad(count($this->workerPids),25); $state.=str_pad(implode(",",array_keys($this->workerPids)),10); echo $state.PHP_EOL; } function getMasterPid() { if (file_exists($this->master_pid_file)){ return file_get_contents($this->master_pid_file); }else{ exit("服务未运行\n"); } } function setMasterPid() { $fp = fopen($this->master_pid_file,"w"); @fwrite($fp,posix_getpid()); @fclose($fp); } function daemon() { $pid = pcntl_fork(); if ($pid<0){ exit("fork进程失败\n"); }else if ($pid >0){ exit(0); }else{ umask(0); $sid = posix_setsid(); if ($sid<0){ exit("创建会话失败\n"); } $pid = pcntl_fork(); if ($pid<0){ exit("进程创建失败\n"); }else if ($pid >0){ exit(0); } //可以关闭标准输入输出错误文件描述符【守护进程不需要】 } } function worker() { if (count($this->workerJob)==0)exit("没有工作任务\n"); foreach($this->workerJob as $job){ $pid = pcntl_fork(); if ($pid<0){ exit("工作进程创建失败\n"); }else if ($pid==0){ /***************子进程工作范围**********************/ //给子进程安装信号处理程序 $this->workerInstallSignal(); $start_time = time(); while (1){ pcntl_signal_dispatch(); if ((time()-$start_time)>=$job->job_run_time){ break; } $job->run(posix_getpid()); } exit(0);//子进程运行完成后退出 /***************子进程工作范围**********************/ }else{ $this->workerPids[$pid] = $job; } } } function workerInstallSignal() { pcntl_signal(SIGUSR1,[__CLASS__,'workerHandleSignal'],false); } function workerHandleSignal($signal) { switch ($signal){ case SIGUSR1: $state = "worker pid=".posix_getpid()."接受了父进程发来的自定义信号\n"; file_put_contents($this->state_file,$state,FILE_APPEND); break; } } function installSignal() { pcntl_signal(SIGINT,[__CLASS__,'handleMasterSignal'],false); pcntl_signal(SIGTERM,[__CLASS__,'handleMasterSignal'],false); pcntl_signal(SIGUSR1,[__CLASS__,'handleMasterSignal'],false); } function handleMasterSignal($signal) { switch ($signal){ case SIGINT: //主进程接受到中断信号ctrl+c foreach ($this->workerPids as $pid=>$worker){ posix_kill($pid,SIGINT);//向所有的子进程发出 } exit("服务平滑停止\n"); break; case SIGTERM://ctrl+z foreach ($this->workerPids as $pid=>$worker){ posix_kill($pid,SIGKILL);//向所有的子进程发出 } exit("服务停止\n"); break; case SIGUSR1://用户自定义信号 if (file_exists($this->state_file)){ unlink($this->state_file); } foreach ($this->workerPids as $pid=>$worker){ posix_kill($pid,SIGUSR1); } $state = "master pid\n".$this->getMasterPid()."\n"; while(!file_exists($this->state_file)){ sleep(1); } $state.= file_get_contents($this->state_file); echo $state.PHP_EOL; break; } } } <?php /**\ * Created by PhpStorm.\ * User: 1655664358@qq.com * Date: 2018/3/26\ * Time: 14:37\ */\namespace Chen\Worker; class Job { public $job_run_time = 3600; function run($pid) {\sleep(3); echo "worker pid = $pid job 没事干,就在这里job\n"; } } <?php /** * Created by PhpStorm.\ * User: 1655664358@qq.com * Date: 2018/3/26\ * Time: 14:37\ */\namespace Chen\Worker; class Talk { public $job_run_time = 3600; function run($pid) {\sleep(3); echo "worker pid = $pid job 没事干,就在这里talk\n"; } } <?php /** * Created by PhpStorm.\ * User: 1655664358@qq.com * Date: 2018/3/26\ * Time: 15:45\ */ require_once 'vendor/autoload.php'; $process = new \Chen\Worker\Server(); $process->workerJob = [new \Chen\Worker\Talk(),new \Chen\Worker\Job()]; $process->run();
For more Laravel related technical articles, please visit Laravel Framework Getting Started Tutorial column for learning!
The above is the detailed content of PHP multi-process and signal interrupt realize multi-task resident memory management [Master/Worker model]. For more information, please follow other related articles on the PHP Chinese website!
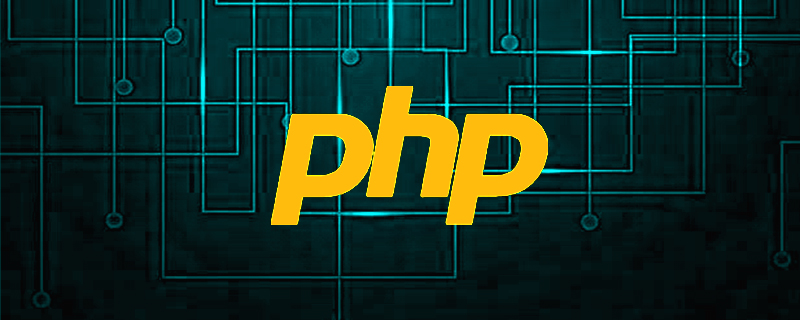
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
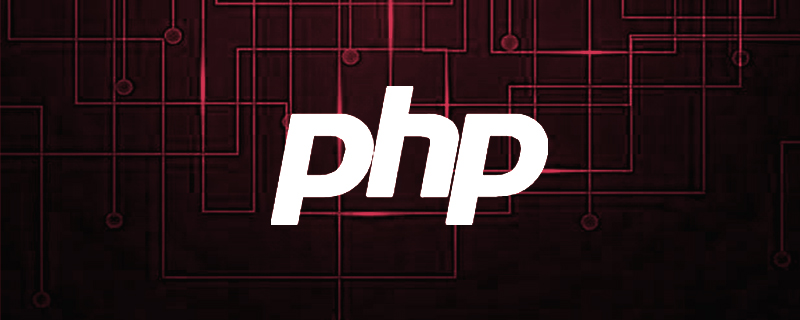
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
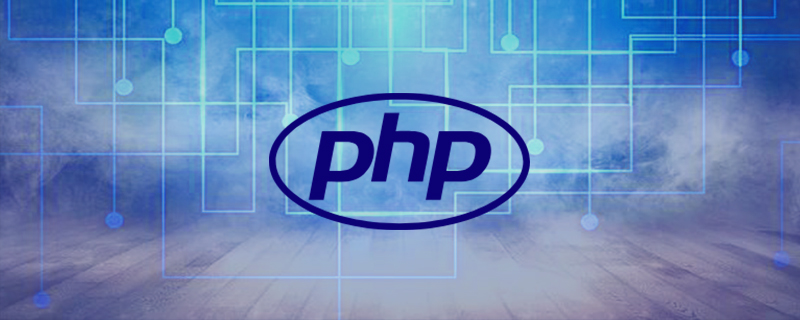
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
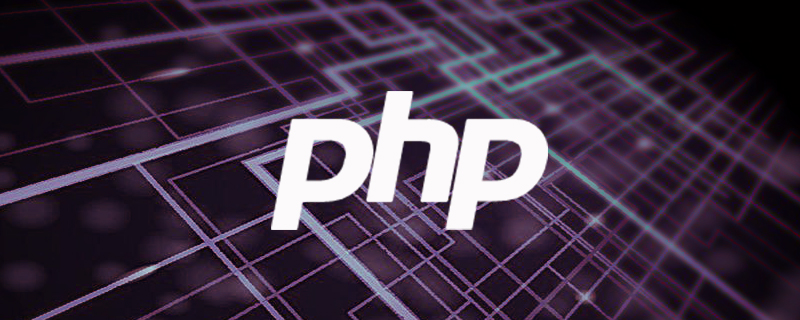
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
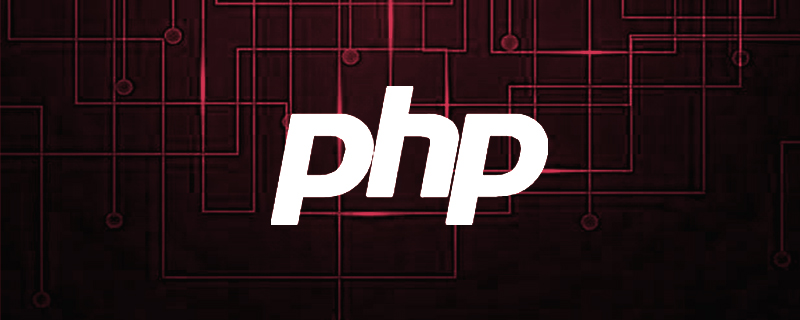
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
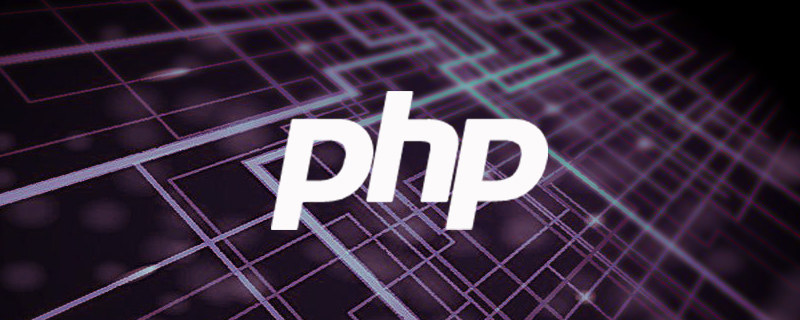
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
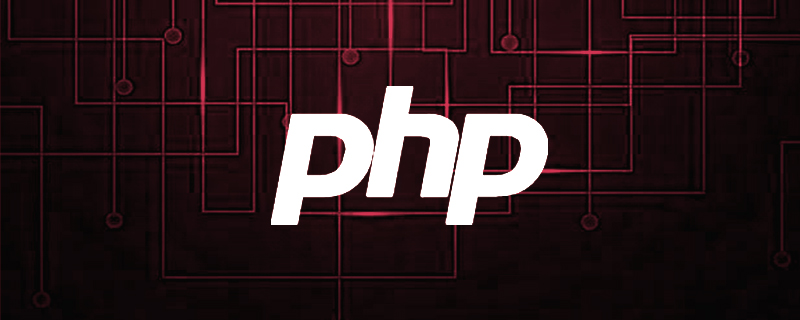
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
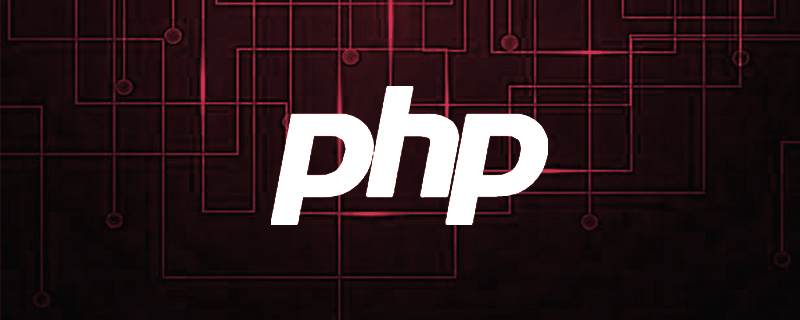
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
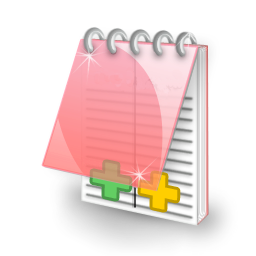
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
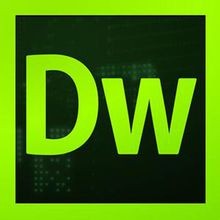
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
