Some ways to improve code quality in php
1. Do not use relative paths
We often see:
require_once('../../lib/some_class.php');
This method has many shortcomings:
It first looks for the specified php include path, then looks for the current directory, so too many paths are checked. If the script is included by a script in another directory, its base directory becomes the directory where the other script is located. Another problem is that when the scheduled task runs the script, its parent directory may not be the working directory.
So the best option is to use an absolute path:
define('ROOT' , '/var/www/project/'); require_once(ROOT . '../../lib/some_class.php'); //rest of the code
We define an absolute path and the value is written Dead. We can still improve it. The path /var/www/project may also change, so do we have to change it every time? No, We can use the __FILE__ constant, such as:
//suppose your script is /var/www/project/index.php //Then __FILE__ will always have that full path. define('ROOT' , pathinfo(__FILE__, PATHINFO_DIRNAME)); require_once(ROOT . '../../lib/some_class.php'); //rest of the code
Now, no matter which directory you move to, such as moving to an external server, the code will run correctly without any changes.
#2. Do not use require, include, include_once, required_once directly
can be used in the script head Introduce multiple files, such as class libraries, tool files and helper functions, such as:
require_once('lib/Database.php'); require_once('lib/Mail.php'); require_once('helpers/utitlity_functions.php');
This usage is quite primitive. It should be more flexible. A helper function should be written Include the file. For example:
function load_class($class_name) { //path to the class file $path = ROOT . '/lib/' . $class_name . '.php'); require_once( $path ); } load_class('Database'); load_class('Mail');
What's the difference? The code is more readable. In the future you can extend the function as needed, like:
function load_class($class_name) { //path to the class file $path = ROOT . '/lib/' . $class_name . '.php'); if(file_exists($path)) { require_once( $path ); } }
You can do more:
Find multiple directories for the same file and easily change the directory where class files are placed. , there is no need to modify the code one by one, you can use similar functions to load files, such as html content.
3. Keep debugging code for the application
In the development environment, we print the database query statement and dump the problematic variable values. And once the problem is solved, we comment or delete them. However a better approach is to keep the debug code. In the development environment, you can:define('ENVIRONMENT' , 'development'); if(! $db->query( $query ) { if(ENVIRONMENT == 'development') { echo "$query failed"; } else { echo "Database error. Please contact administrator"; } }
In the server, you can:
define('ENVIRONMENT' , 'production'); if(! $db->query( $query ) { if(ENVIRONMENT == 'development') { echo "$query failed"; } else { echo "Database error. Please contact administrator"; } }Recommended tutorial:
The above is the detailed content of How to ensure code quality in PHP. For more information, please follow other related articles on the PHP Chinese website!
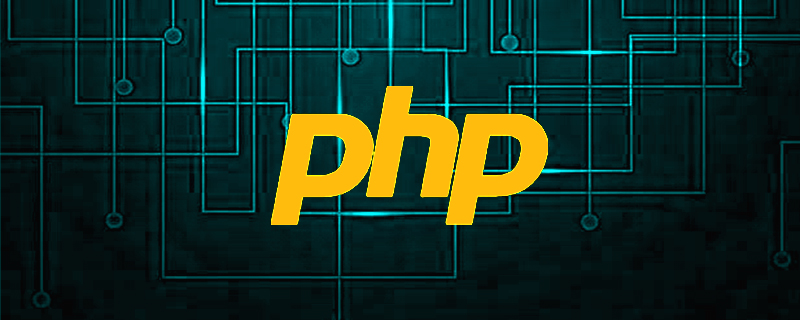
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
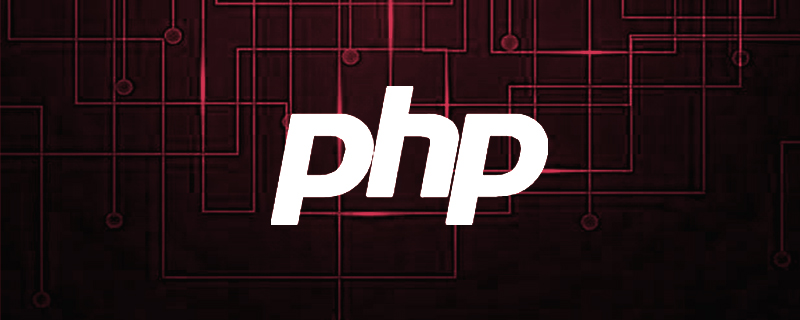
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
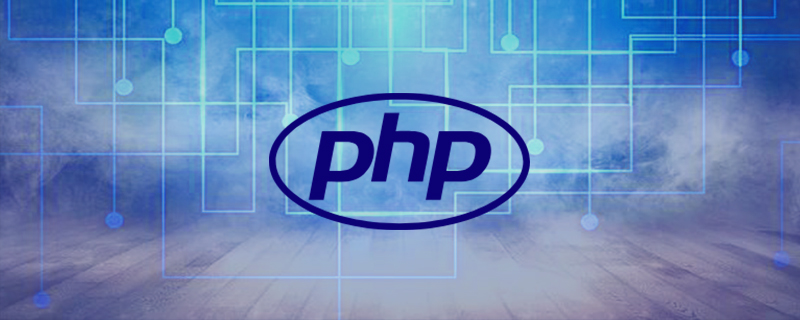
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
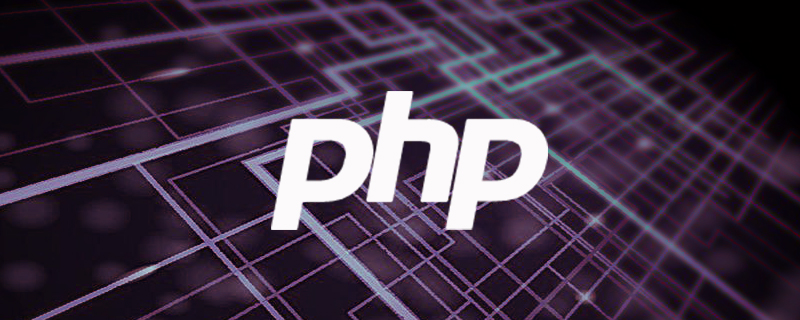
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
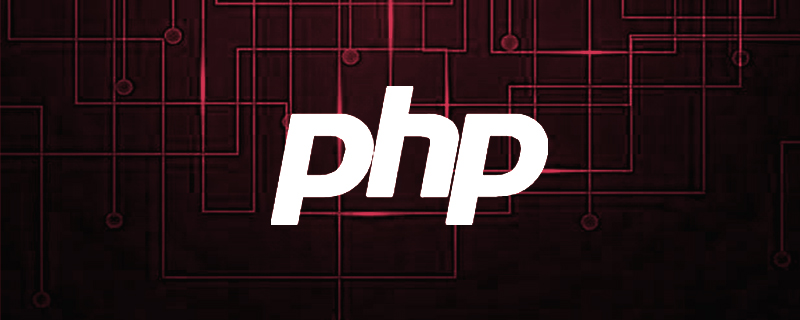
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
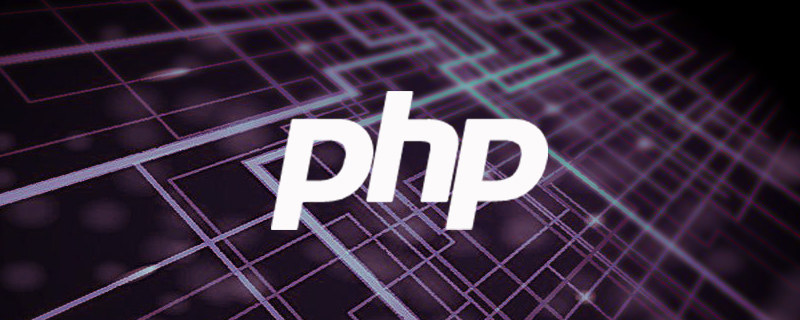
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
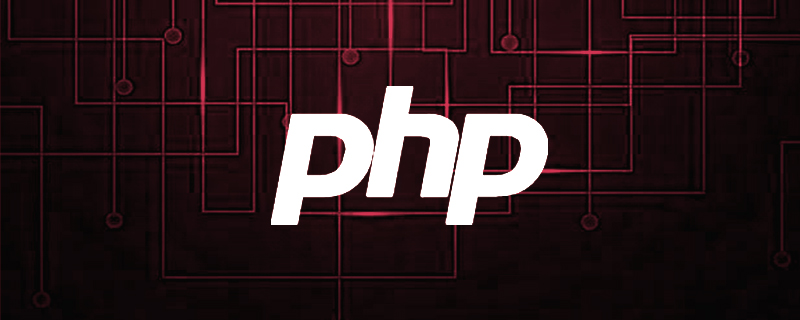
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
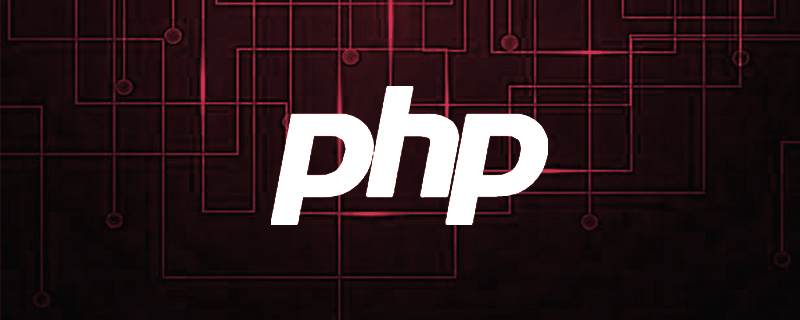
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
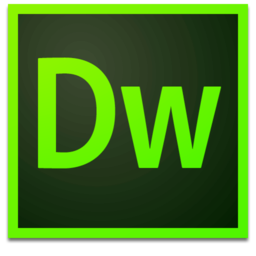
Dreamweaver Mac version
Visual web development tools
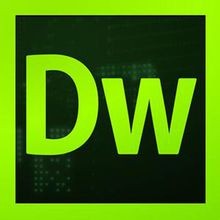
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
