In PHP5, the type of variables is undefined. A variable can point to any type of numerical value, string, object, resource, etc. We cannot say that polymorphism in PHP5 is a variable.
We can only say that in PHP5, polymorphism is applied in the type hint position of method parameters. Any subclass object of a class can satisfy the type requirement with the current type as a type hint.
All classes that implement this interface can meet the method parameter requirements with the interface type as a type hint. Simply put, a class has the identity of its parent class and implemented interfaces.
Achieve polymorphism by implementing interfaces
<?php interface User{ // User接口 public function getName(); public function setName($_name); } class NormalUser implements User { // 实现接口的类. private $name; public function getName(){ return $this->name; } public function setName($_name){ $this->name = $_name; } } class UserAdmin{ //操作. public static function ChangeUserName(User $_user,$_userName){ $_user->setName($_userName); } } $normalUser = new NormalUser(); UserAdmin::ChangeUserName($normalUser,"Tom");//这里传入的是 NormalUser的实例. echo $normalUser->getName(); ?>
Use interfaces and composition to simulate multiple inheritance
Simulate multiple inheritance through composition. Multiple inheritance is not supported in PHP. If we want to use the methods of multiple classes to achieve code reuse, is there any way?
That is combination. In one class, set another class as a property.
The following example simulates multiple inheritance.
Interface Example
Write a conceptual example. We design an online sales system, and the user part is designed as follows: Users are divided into three types: NormalUser, VipUser, and InnerUser. It is required to calculate the price of the product purchased by the user based on the user's different discounts. And require space to be reserved for future expansion and maintenance.
<?php interface User { public function getName(); public function setName($_name); public function getDiscount(); } abstract class AbstractUser implements User { private $name = ""; protected $discount = 0; protected $grade = ""; function __construct($_name) { $this->setName($_name); } function getName() { return $this->name; } function setName($_name) { $this->name = $_name; } function getDiscount() { return $this->discount; } function getGrade() { return $this->grade; } } class NormalUser extends AbstractUser { protected $discount = 1.0; protected $grade = "Normal"; } class VipUser extends AbstractUser { protected $discount = 0.8; protected $grade = "VipUser"; } class InnerUser extends AbstractUser { protected $discount = 0.7; protected $grade = "InnerUser"; } interface Product { function getProductName(); function getProductPrice(); } interface Book extends Product { function getAuthor(); } class BookOnline implements Book { private $productName; protected $productPrice; protected $Author; function __construct($_bookName) { $this->productName = $_bookName; } function getProductName() { return $this->productName; } function getProductPrice() { $this->productPrice = 100; return $this->productPrice; } public function getAuthor() { $this->Author = "chenfei"; return $this->Author; } } class Productsettle { public static function finalPrice(User $_user, Product $_product, $number) { $price = $_user->getDiscount() * $_product->getProductPrice() * $number; return $price; } } $number = 10; $book = new BookOnline("设计模式"); $user = new NormalUser("tom"); $price = Productsettle::finalPrice($user, $book, $number); $str = "您好,尊敬的" . $user->getName() . "<br />"; $str .= "您的级别是" . $user->getGrade() . "<br />"; $str .= "您的折扣是" . $user->getDiscount() . "<br />"; $str .= "您的价格是" . $price; echo $str; ?>
Recommended tutorial: PHP video tutorial
The above is the detailed content of What forms does php use to implement polymorphism?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
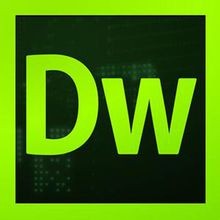
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
