This summary is mainly based on my previous series of articles on the basics of design patterns. The main thing is to explain the important knowledge points in my own words. There may be some mistakes, so I hope you can forgive me and give me some guidance.
Design Pattern
Creative Pattern
The role of Creational Pattern is Create an object. When it comes to creating an object, the most familiar thing is to create a new object and then set related properties. However, in many scenarios, we need to provide clients with a more friendly way of creating objects, especially when we define classes but need to provide them to other developers.
Single instance
单例模式保证全局的单例类只有一个实例,这样的话使用的时候直接获取即可,比如数据库的一个连接,Spring里的bean,都可以是单例的。 单例模式一般有5种写法。 第一种是饿汉模式,先把单例进行实例化,获取的时候通过静态方法直接获取即可。缺点是类加载后就完成了类的实例化,浪费部分空间。 第二种是饱汉模式,先把单例置为null,然后通过静态方法获取单例时再进行实例化,但是可能有多线程同时进行实例化,会出现并发问题。 第三种是逐步改进的方法,一开始可以用synchronized关键字进行同步,但是开销太大,而后改成使用volatile修饰单例,然后通过一次检查判断单例是否已初始化,如果未初始化就使用synchronized代码块,再次检查单例防止在这期间被初始化,而后才真正进行初始化。 第四种是使用静态内部类来实现,静态内部类只在被使用的时候才进行初始化,所以在内部类中进行单例的实例化,只有用到的时候才会运行实例化代码。然后外部类再通过静态方法返回静态内部类的单例即可。 第五种是枚举类,枚举类的底层实现其实也是内部类。枚举类确保每个类对象在全局是唯一的。所以保证它是单例,这个方法是最简单的。
Factory pattern
简单工厂一般是用一个工厂创建多个类的实例。 工厂模式一般是指一个工厂服务一个接口,为这个接口的实现类进行实例化 抽象工厂模式是指一个工厂服务于一个产品族,一个产品族可能包含多个接口,接口又会包含多个实现类,通过一个工厂就可以把这些绑定在一起,非常方便。
Prototype pattern
一般通过一个实例进行克隆从而获得更多同一原型的实例。使用实例的clone方法即可完成。
Builder Pattern
建造者模式中有一个概念叫做链式调用,链式调用为一个类的实例化提供便利,一般提供系列的方法进行实例化,实际上就是将set方法改造一下,将原本返回为空的set方法改为返回this实例,从而实现链式调用。 建造者模式在此基础上加入了builder方法,提供给外部进行调用,同样使用链式调用来完成参数注入。
Structural Pattern
The previous creational pattern introduced some design patterns for creating objects. The structure introduced in this section The pattern aims to achieve decoupling by changing the code structure, making our code easier to maintain and expand.
Adapter pattern
The adapter pattern is used to adapt two different classes.
Similarities and differences between adapter mode and proxy mode
Comparing these two modes is actually comparing object adapter mode and proxy mode. In terms of code structure,
They are very similar. All require an instance of a specific implementation class.
But their purposes are different. The proxy mode does the work of enhancing the original method;
The adapter does the work of adaptation, in order to provide "packaging chicken into duck, Then use it as a duck",
There is no inheritance relationship between chickens and ducks.
The adapter pattern can be divided into class adapter, object adapter, etc.
A class adapter can adapt itself to the parent class by inheriting the parent class.
The object adapter needs to pass the object into the constructor of another object for packaging.
Flyweight mode
/ The core of the Flyweight mode lies in the Flyweight factory class.
// The function of the Flyweight factory class is to provide a Flyweight pool used to store flyweight objects,
// When the user needs an object, first obtain it from the flyweight pool,
// If it does not exist in the flyweight pool, create it A new flyweight object is returned to the user,
// Save the new object in the flyweight pool.
//Flyweight Pattern
// English is Flyweight Pattern. I don’t know who translated this word first. I feel that the translation is really difficult to understand. We tried to force the connection. Bar. Flyweight means lightweight. Flyweight refers to shared components, that is, reusing already generated objects. This approach is of course lightweight.
// The simplest way to reuse objects is to use a HashMap to store each newly generated object. Every time you need an object, first go to the HashMap to see if it exists. If not, generate a new object, and then put this object into the HashMap.
// I won’t demonstrate this simple code.
Agent Mode
// We found that there is no such thing. To put it bluntly, the proxy mode is to do "method packaging" or "method enhancement".
// In aspect-oriented programming, forget about this term. In AOP,
// is actually the process of dynamic proxy. For example, in Spring,
// we do not define the proxy class ourselves, but Spring will help us dynamically define the proxy,
// and then define us in @Before, @After, @Around The code logic in is dynamically added to the agent.
Appearance mode
Appearance mode generally encapsulates specific implementation details and provides users with a simpler interface.
You can get the required content through a method call.
Combined mode
//Combined mode is used to represent data with a hierarchical structure, making our access to single objects and combined objects consistent.
//Let’s look at an example directly. Each employee has attributes such as name, department, and salary.
// There is also a collection of subordinate employees (although the collection may be empty),
// The subordinate employees have the same structure as their own.
// They also have attributes such as name and department.
// They also have a collection of their subordinate employees.
class Employee { private String name; private String dept; private int salary; private List<employee> subordinates; // 下属 }</employee>
Decorator pattern
Decorator
The Decorator pattern inherits each enhanced class from the highest-level parent class . Then when functionality enhancement is needed, just pass the class instance into the enhanced class, and then the enhanced class can enhance the functionality of the original class when used.
Different from the proxy mode, in the decorator mode, each decoration class inherits the parent class and can be encapsulated at multiple levels.
Behavioral pattern
Behavioral pattern focuses on the interaction between various classes, clearly dividing responsibilities, making our code clearer.
Strategy mode
The strategy mode generally regards a strategy as a class, and passes in the instance when the strategy needs to be specified, so we can use the algorithm where Pass in the specified algorithm.
Command Mode
The command mode is generally divided into three roles: command initiator, command and command receiver.
The command initiator needs to inject the command instance when using it. Then execute the command call.
The command call actually calls the command receiver's method to make the actual call.
For example, the remote control button is equivalent to a command. When the button is clicked, the command runs and the method provided by the TV is automatically called.
Template method pattern
Template method generally refers to providing a method template, which contains some implementation classes and some abstract classes, and specifies the execution sequence.
Implementation classes are good methods provided by templates. Abstract classes require users to implement them themselves.
The template method specifies the execution order of methods in a template, which is very suitable for some development frameworks, so the template method is also widely used in open source frameworks.
Observer pattern and event listening mechanism
The observer pattern is generally used for data subscription between subscribers and message publishers.
Generally divided into observers and topics. Observers subscribe to topics and register instances to the observer list maintained by the topic.
When the topic updates data, it automatically pushes the data to the observer or notifies the observer that the data has been updated.
But due to this method, the coupling relationship of message push is relatively tight. And it's hard to know what the data type is without opening the data.
I know that in order to make the data format more flexible, the event and event listener patterns were used. The event type and event data wrapped by the event were decoupled from the subject and observer.
Topic When an event occurs, all the listeners of the event are triggered, and the event is sent to each listener through the listener list. After listening to the event, first find the corresponding event type according to the event type it supports. event handler, and then use the handler to process the corresponding event.
Responsibility chain model
The responsibility chain usually needs to establish a one-way linked list first, and then the caller only needs to call the head node, and it will flow automatically later. . For example, process approval is a good example. As long as the end user submits an application, a chain of responsibility will be automatically established based on the content information of the application, and then the flow can begin
The above is the detailed content of Introduction to Java Design Patterns. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
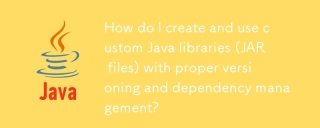
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
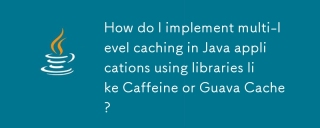
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
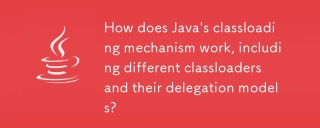
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
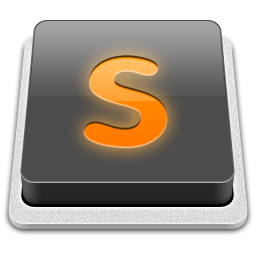
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
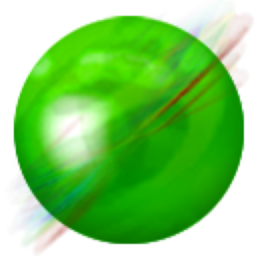
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment