The efficiency difference between using constructors and using setters in Java
When optimizing Java code, try every means to improve the overall efficiency. Use JProfiler to look at the time proportion of the code, and then see which parts can be optimized to reduce the running time. There are several directions below.
1. If you can use the constructor to get it right in one step, try to use the constructor instead of using setter functions one by one.
2. If you can use the array , just use an array. Instead of lists, arrays are really fast.
3. When using a for loop, use the for i loop to target the collection of list interfaces instead of the for each loop. When the for i loop is executed, the length value is mentioned outside the for loop.
4. Some variables can be extracted once and used multiple times. Don't get frequently. Even a simple int value.
5. If you can use inner classes, you can use inner classes, which can save the use of getter and setter methods.
6, for json serialization and deserialization, don’t say that fastjson is faster in theory, just use fastjson, because based on the simplicity and complexity of the data structure, you have to choose what to use for deserialization and deserialization. You need to actually test it before speaking. You can't just copy the theory directly, because sometimes Gson is really fast.
The following is a comparison of the efficiency of this construct and set
Then, the code used is as follows:
package com.lxk.fast; import com.google.common.collect.Lists; import com.lxk.model.Car; import com.lxk.model.Dog; /** * 测试谁快 直接构造或者一个个set,他们的效率差多少 * * @author LiXuekai on 2019/6/18 */ public class FastIsConstructOrSet { public static void main(String[] args) { testFast(); } /** * 使用JProfiler看时间占比 */ private static void testFast() { while (true) { //27.4% set(); //72.6% construct(); } } /** * 构造函数来给属性赋值 */ private static void construct() { Car car = new Car("oooo", 100, Lists.newArrayList(new Dog("aaa", true, true))); } /** * set来给属性赋值 */ private static void set() { Car car = new Car(); car.setSign("oooo"); car.setPrice(100); Dog dog = new Dog(); dog.setName("aaa"); dog.setAlive(true); dog.setLoyal(true); car.setMyDog(Lists.newArrayList(dog)); } }
It can be found that the construction is much faster than setting it one by one. Therefore, when you can set the value of the attribute in one step, considering the efficiency issue, you should do this
( 2019-07-16 Newly added)
Didn’t someone tell me about the builder mode?
I was curious and tested it, just in case this builder mode is faster.
The following is the builder code
/** * 使用JProfiler看时间占比 */ @Test public void testFast2() { while (true) { //33% set(); //12.4% construct(); //54.6% builder(); } } /** * 使用lombok的 builder 模式来赋值 */ private static void builder() { Car car = Car.builder() .sign("0000") .price(100) .myDog(Lists.newArrayList(Dog.builder().name("aaa").alive(true).isLoyal(true).build())) .build(); }
The annotations of Lombok used.
Then the JProfiler monitoring results
The result:
As you can see, it is still the constructor function Niu x , he is still faster. In addition, the above ratio: 72.6: 27.4 = 33: 12.4 = 2.64
The time ratio of construction and set has not changed.
The above is the detailed content of The efficiency difference between using constructors and using setters in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
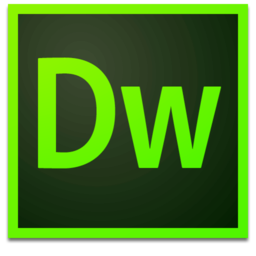
Dreamweaver Mac version
Visual web development tools
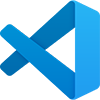
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft