#The import statement in Python is used to call modules.
1. Module
Usually the module is a file, just use import to import it directly. The file types that can be used as modules include ".py", ".pyo", ".pyc", ".pyd", ".so", and ".dll".
2. Package
Usually a package is always a directory. You can use import to import the package, or from import to import some modules in the package. The first file in the package directory is __init__.py. Then there are some module files and subdirectories. If there is also __init__.py in the subdirectory, then it is a subpackage of this package.
Module
You can use the import statement to import a source code file as a module. For example:
# file : spam.py a = 37 # 一个变量 def foo: # 一个函数 print "I'm foo" class bar: # 一个类 def grok(self): print "I'm bar.grok" b = bar() # 创建一个实例
Use the import spam statement to import this file as a module. When the system imports a module, it must do the following three things:
1. Create a namespace for the objects defined in the source code file. Through this namespace, you can access the functions and variables defined in the module.
2. Execute the source code file in the newly created namespace.
3. Create an object named the source code file, which references the module’s namespace, so that it can be executed through This object accesses functions and variables in the module, such as:
import spam # 导入并运行模块 spam print spam.a # 访问模块 spam 的属性 spam.foo() c = spam.bar() ...
Use commas to separate the module names to import multiple modules at the same time:
import socket, os, regex modules can be imported using the as keyword. Change the name of the reference object of the module:
import os as system import socket as net, thread as threads system.chdir("..") net.gethostname()
Use the from statement to directly import the objects in the module into the current name space. The from statement does not create a reference object to the module name space, but imports the imported module One or more objects are directly placed into the current namespace:
from socket import gethostname # 将gethostname放如当前名字空间 print gethostname() # 直接调用 socket.gethostname() # 引发异常NameError: socket
The from statement supports comma-separated objects, and an asterisk (*) can also be used to represent all objects in the module except those starting with an underscore:
from socket import gethostname, socket from socket import * # 载入所有对象到当前名字空间
The above is the detailed content of What does import in python mean?. For more information, please follow other related articles on the PHP Chinese website!
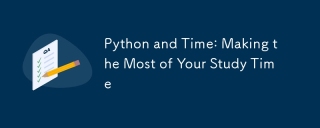
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
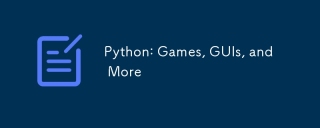
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
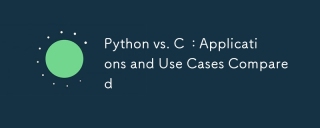
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
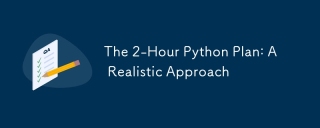
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
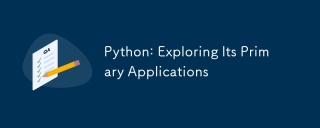
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
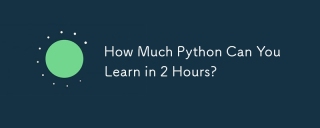
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
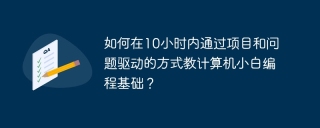
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
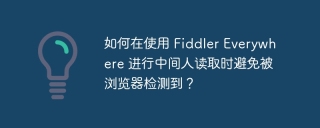
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
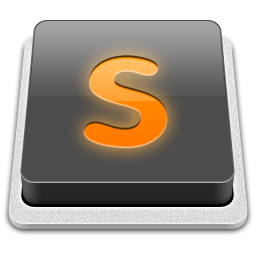
SublimeText3 Mac version
God-level code editing software (SublimeText3)
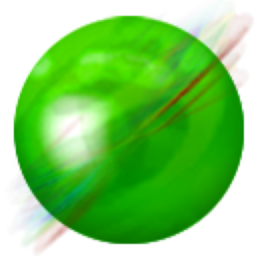
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
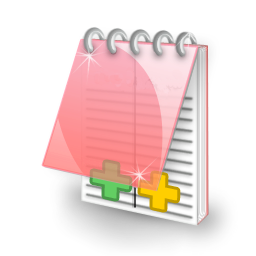
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function