Four ways of inheritance in javascript (code examples)
This article brings you the four methods (code examples) of JavaScript inheritance. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1. Prototype chain inheritance
Core: Use the instance of the parent class as the prototype of the subclass
Disadvantages: New prototype method for the parent class/ Prototype attributes can be accessed by subclasses. Once the parent class changes, everything else changes
function Person (name) { this.name = name; }; Person.prototype.getName = function () { //对原型进行扩展 return this.name; }; function Parent (age) { this.age = age; }; Parent.prototype = new Person('老明'); //这一句是关键 //通过构造器函数创建出一个新对象,把老对象的东西都拿过来。 Parent.prototype.getAge = function () { return this.age; }; // Parent.prototype.getName = function () { //可以重写从父类继承来的方法,会优先调用自己的。 // console.log(222); // }; var result = new Parent(22); console.log(result.getName()); //老明 //调用了从Person原型中继承来的方法(继承到了当前对象的原型中) console.log(result.getAge()); //22 //调用了从Parent原型中扩展来的方法
2. Constructive inheritance
Basic idea
Borrow constructor The basic idea is to use call
or apply
to copy (borrow) the properties and methods specified by this
in the parent class to the instance created by the subclass.
Because this
object is bound at runtime based on the execution environment of the function. That is, globally, this
is equal to window
, and when a function is called as a method of an object, this
is equal to that object. The call
, apply
methods can change the object context of a function from the initial context to the new object specified by thisObj.
So, this borrowed constructor is, when new
the object (when new is created, this
points to the created instance), a new instance is created Object,
and execute the code inside Parent
, and Parent
uses call
to call Person
, that is to say, this
points to a new instance,
so the this
related properties and methods in Person
will be assigned to the new instance, and Instead of assigning the value to Person
,
so all instances have these this
attributes and methods defined by the parent class.
Because the properties are bound to this
, they are assigned to the corresponding instances when called, and the values of each instance will not affect each other.
Core: Using the constructor of the parent class to enhance the instance of the subclass is equivalent to copying the instance attributes of the parent class to the subclass (no prototype is used)
Disadvantages: Methods are all in the constructor As defined in, only instance properties and methods of the parent class can be inherited, prototype properties/methods cannot be inherited, and function reuse cannot be achieved. Each subclass has a copy of the parent class instance function, which affects performance
function Person (name) { this.name = name; this.friends = ['小李','小红']; this.getName = function () { return this.name; } }; // Person.prototype.geSex = function () { //对原型进行扩展的方法就无法复用了 // console.log("男"); // }; function Parent = (age) { Person.call(this,'老明'); //这一句是核心关键 //这样就会在新parent对象上执行Person构造函数中定义的所有对象初始化代码, // 结果parent的每个实例都会具有自己的friends属性的副本 this.age = age; }; var result = new Parent(23); console.log(result.name); //老明 console.log(result.friends); //["小李", "小红"] console.log(result.getName()); //老明 console.log(result.age); //23 console.log(result.getSex()); //这个会报错,调用不到父原型上面扩展的方法
3. Combined inheritance
Combined inheritance (all instances can have their own properties and can use the same method. Combined inheritance avoids the defects of the prototype chain and borrowed constructors, and combines the two The advantage of each is the most commonly used inheritance method)
Core: By calling the parent class constructor, inherit the properties of the parent class and retain the advantages of passing parameters, and then use the parent class instance as the prototype of the subclass to achieve Function reuse
Disadvantages: The parent class constructor is called twice and two instances are generated (the subclass instance blocks the one on the subclass prototype)
function Person (name) { this.name = name; this.friends = ['小李','小红']; }; Person.prototype.getName = function () { return this.name; }; function Parent (age) { Person.call(this,'老明'); //这一步很关键 this.age = age; }; Parent.prototype = new Person('老明'); //这一步也很关键 var result = new Parent(24); console.log(result.name); //老明 result.friends.push("小智"); // console.log(result.friends); //['小李','小红','小智'] console.log(result.getName()); //老明 console.log(result.age); //24 var result1 = new Parent(25); //通过借用构造函数都有自己的属性,通过原型享用公共的方法 console.log(result1.name); //老明 console.log(result1.friends); //['小李','小红']
4. Parasitic combination inheritance
Core: Through parasitism, the instance attributes of the parent class are cut off, so that when the constructor of the parent class is called twice, the instance method will not be initialized twice/ Attributes avoid the shortcomings of combined inheritance
Disadvantages: It is perfect, but the implementation is more complicated
function Person(name) { this.name = name; this.friends = ['小李','小红']; } Person.prototype.getName = function () { return this.name; }; function Parent(age) { Person.call(this,"老明"); this.age = age; } (function () { var Super = function () {}; // 创建一个没有实例方法的类 Super.prototype = Person.prototype; Parent.prototype = new Super(); //将实例作为子类的原型 })(); var result = new Parent(23); console.log(result.name); console.log(result.friends); console.log(result.getName()); console.log(result.age);
[Related recommendations: JavaScript video tutorial]
The above is the detailed content of Four ways of inheritance in javascript (code examples). For more information, please follow other related articles on the PHP Chinese website!
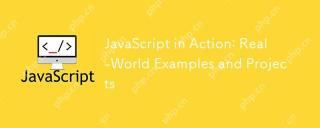
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
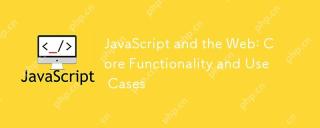
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
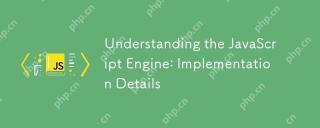
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
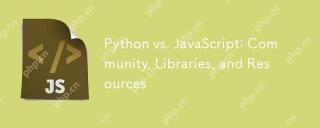
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
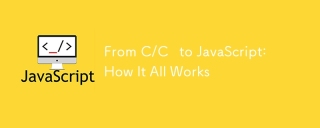
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
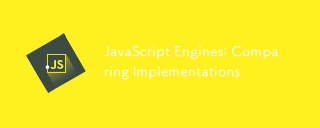
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
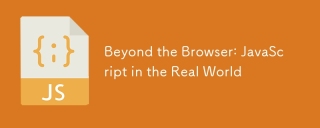
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
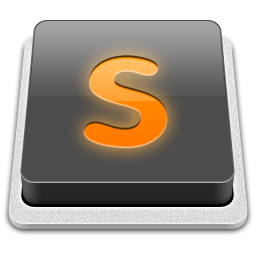
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.