Memory leak means that when an object is ineffective and should be recycled, but it cannot be recycled because of another object's reference to it, and it remains in the heap memory, it is called a memory leak. Common ones include unexpected global variables, DOM leaks and circular references, etc.
[Recommended course: JavaScript Tutorial】
Memory leak
Memory leak generally refers to when an object has no effect and should be recycled. Another object in use cannot be recycled due to its reference. This object that cannot be recycled stays in the heap memory, which causes a memory leak.
When an object is no longer needed When the object that is supposed to be recycled is used, another object in use holds a reference to it, causing it to not be recycled. This causes the object that should be recycled to not be recycled and stays in the heap memory, which causes a memory leak.
Common memory leaks:
1. Unexpected global variables
The way Js handles undefined variables: Undefined The variable will create a new variable in the global object. In the browser, the global object is window.
function foo(arg) { bar = "this is a hidden global variable"; //等同于window.bar="this is a hidden global variable" this.bar2= "potential accidental global";//这里的this 指向了全局对象(window), 等同于window.bar2="potential accidental global"}
Solution: Add and enable strict mode 'use strict' in the JavaScript program to effectively avoid the above problems.
Note: Global variables used to temporarily store large amounts of data must be set to null or reassigned after ensuring that the data is processed.
2. DOM leakage
In the browser, the engines used by DOM and JS are different. DOM uses a rendering engine, while JS uses v8 engine, so using JS to operate DOM will consume more performance, so in order to reduce DOM operations, we will use variable references to cache them in the current environment. If you perform some deletion and update operations, you may forget to release the cached DOM, thus causing a memory leak
Example: Reference to uncleaned DOM elements
var refA = document.getElementById('refA'); document.body.removeChild(refA); // #refA不能回收,因为存在变量refA对它的引用。 将其对#refA引用释放,但还是无法回收#refA。
Solution: Set refA = null;
3. Forgotten timers and callback functions
var someResource = getData(); setInterval(function() { var node = document.getElementById('Node'); if(node) { node.innerHTML = JSON.stringify(someResource)); } }, 1000);
Such code is very common, if the element with id is Node If removed from the DOM, the timer will still exist. At the same time, because the callback function contains a reference to someResource, someResource outside the timer will not be released.
4. Circular Reference
In the memory management environment of js, if object A has permission to access the object B, it is called object A referencing object B. The reference counting strategy is to see if the object has other objects referencing it. If no object refers to the object, then the object will be recycled.
var obj1 = { a: 1 }; // 一个对象(称之为 A)被创建,赋值给 obj1,A 的引用个数为 1 var obj2 = obj1; // A 的引用个数变为 2 obj1 = 0; // A 的引用个数变为 1 obj2 = 0; // A 的引用个数变为 0,此时对象 A 就可以被垃圾回收了
But the biggest problem with reference counting is circular references.
function func() { var obj1 = {}; var obj2 = {}; obj1.a = obj2; // obj1 引用 obj2 obj2.a = obj1; // obj2 引用 obj1 }
When the function is executed, the return value is undefined, so the entire function and internal variables should be recycled. However, according to the reference counting method, the number of references of obj1 and obj2 is not 0, so they are not will be recycled. So to solve this problem, you can set their values to null
Summary: The above is the entire content of this article, I hope it will be helpful to everyone.
The above is the detailed content of What is a memory leak and how to fix it. For more information, please follow other related articles on the PHP Chinese website!
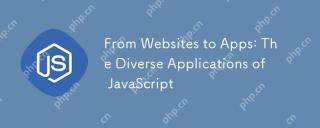
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
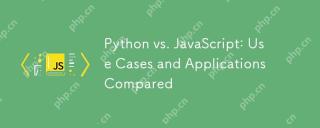
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
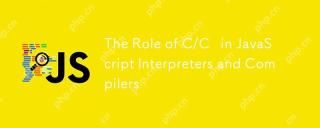
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
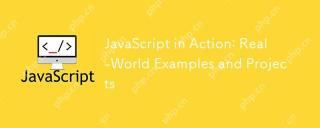
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
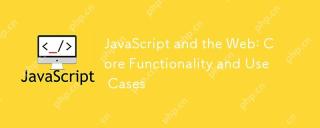
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
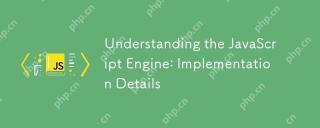
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
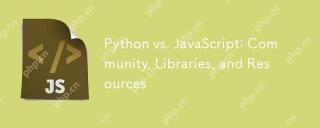
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
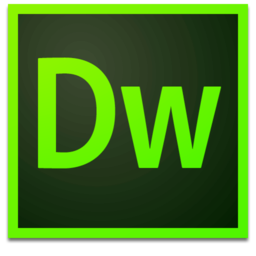
Dreamweaver Mac version
Visual web development tools
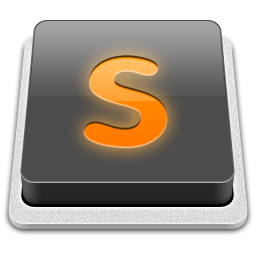
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
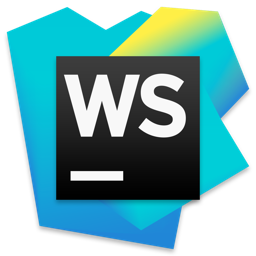
WebStorm Mac version
Useful JavaScript development tools