This article brings you a summary of the basic knowledge of Java multi-threading (with code). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Java main thread name
A program we start can be understood as a process. A process contains a main thread, and a thread can be understood as a subtask. Java You can get the default main thread name through the following code.
System.out.println(Thread.currentThread().getName());
The running result is main, which is the name of the thread and not the main method. The main method is executed through this thread.
Two ways to create threads
1. Inherit the Thread class
public class Thread1 extends Thread { @Override public void run() { System.out.println("qwe"); } }
2. Implement the Runnable interface
public class Thread2 implements Runnable { @Override public void run() { System.out.println("asd"); } }
Thread implements the Runnable interface. And runs in multiple threads , the execution order of the code has nothing to do with the calling order. In addition, if the start method is called multiple times, java.lang.IllegalThreadStateException will be thrown
currentThread method
Returns which thread the current code is being used by Call the .
public class Thread1 extends Thread { public Thread1() { System.out.println("构造方法的打印:" + Thread.currentThread().getName()); } @Override public void run() { System.out.println("run 方法的打印:" + Thread.currentThread().getName()); } }
Thread1 thread1 = new Thread1(); thread1.start();
isAlive method
to determine whether the current thread is active.
public class Thread1 extends Thread { @Override public void run() { System.out.println("run 方法的打印 Thread.currentThread().isAlive() == " + Thread.currentThread().isAlive()); System.out.println("run 方法的打印 this.isAlive() == " + this.isAlive()); System.out.println("run 方法的打印 Thread.currentThread().isAlive() == this.isAlive() == " + (Thread.currentThread() == this ? "true" : "false")); } }
Thread1 thread1 = new Thread1(); System.out.println("begin == " + thread1.isAlive()); thread1.start(); Thread.sleep(1000); System.out.println("end == " + thread1.isAlive());
The execution result is as follows
begin == false run 方法的打印 Thread.currentThread().isAlive() == true run 方法的打印 this.isAlive() == true run 方法的打印 Thread.currentThread() == this == true end == false
thread1 will be executed after 1 second. So the output result is false. And Thread.currentThread() and this are the same object. It can be understood that the thread object that executes the current run method is ourselves (this).
If the thread object is constructed as a parameter When the method is passed to the Thread object for start(), the execution result is different from the previous instance. The reason for this difference is still from the difference between Thread.currentThread() and this.
System.out.println("begin == " + thread1.isAlive()); //thread1.start(); // 如果将线程对象以构造参数的方式传递给 Thread 对象进行 start() 启动 Thread thread = new Thread(thread1); thread.start(); Thread.sleep(1000); System.out.println("end == " + thread1.isAlive());
Execution results
begin == false run 方法的打印 Thread.currentThread().isAlive() == true run 方法的打印 this.isAlive() == false run 方法的打印 Thread.currentThread() == this == false end == false
Thread.currentThread().isAlive() is true because Thread.currentThread() returns a thread object, and we also use this object to start the thread, so it is in the active state .
It is easier to understand if this.isAlive() is false, because we execute the run method through the thread started by the thread object. So it is false. It also means that the two are not the same object. .
sleep method
Let the current "executing thread" sleep within the specified number of milliseconds. The "executing thread" can only be Thread.currentThread()
The returned thread.
Thread.sleep(1000);
Stop the thread
Stopping a thread means stopping the operation being done before the thread completes the task, that is, giving up the current operation.
There are the following 3 methods to terminate a running thread in Java:
Use the exit flag to make the thread exit normally, that is, the thread terminates when the run method is completed.
Use the stop method to forcefully terminate the thread, but this method is not recommended.
Use the interrupt method to interrupt the thread.
The thread that cannot be stopped
Calling the interrupt method only marks the current thread as stopping, and does not actually stop the thread.
public class Thread1 extends Thread { @Override public void run() { for(int i = 0; i <pre class="brush:php;toolbar:false">Thread1 thread1 = new Thread1(); thread1.start(); Thread.sleep(2000); thread1.interrupt();
We call the interrupt method after two seconds, according to the printed results We can see that the thread does not stop, but after executing 500000 times of this loop, the run method ends and the thread stops.
Judge whether the thread is stopped
After we mark the thread as stopped, we need to judge inside the thread whether the thread is marked as stop, and if so, stop the thread.
Two judgment methods:
Thread.interrupted(); You can also use this.interrupted();
this.isInterrupted();
The following are two Source code of the method:
public static boolean interrupted() { return currentThread().isInterrupted(true); } public boolean isInterrupted() { return isInterrupted(false); }
interrupted()
Method data static method, that is, determine whether the current thread has been interrupted. isInterrupted()
Determine whether the thread has been interrupted.
interrupted()
Key points of the method from the official website.
The interrupt status of the thread is cleared by this method. In other words, if this method is called twice in a row, the second time will return false (except when the current thread is interrupted again after the first call has cleared its interrupt status and before the second call has verified the interrupt status).
Exception stop thread
public class Thread1 extends Thread { @Override public void run() { try { for (int i = 0; i <pre class="brush:php;toolbar:false">Thread1 thread1 = new Thread1(); thread1.start(); Thread.sleep(1000); thread1.interrupt();
Output results, these are the last few lines:
i = 195173 i = 195174 i = 195175 线程停止 线程通过 catch 停止 java.lang.InterruptedException at Thread1.run(Thread1.java:9)
Of course we can also replacethrow new InterruptedException();
withreturn
. It’s all the same End the thread.
Stop in sleep
If the thread calls the Thread.sleep()
method to make the thread sleep, then we call thread1.interrupt ()
will be thrown after. InterruptedException
Exception.
java.lang.InterruptedException: sleep interrupted at java.lang.Thread.sleep(Native Method) at Thread1.run(Thread1.java:8)
Violently stopping the thread
Violently stopping the thread can use the stop
method, but This method is obsolete and is not recommended for the following reasons.
即刻抛出 ThreadDeath 异常, 在线程的run()方法内, 任何一点都有可能抛出ThreadDeath Error, 包括在 catch 或 finally 语句中. 也就是说代码不确定执行到哪一步就会抛出异常.
释放该线程所持有的所有的锁. 这可能会导致数据不一致性.
public class Thread1 extends Thread { private String userName = "a"; private String pwd = "aa"; public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getPwd() { return pwd; } public void setPwd(String pwd) { this.pwd = pwd; } @Override public void run() { this.userName = "b"; try { Thread.sleep(100000); } catch (InterruptedException e) { e.printStackTrace(); } this.pwd = "bb"; } }
Thread1 thread1 = new Thread1(); thread1.start(); Thread.sleep(1000); thread1.stop(); System.out.println(thread1.getUserName() + " " + thread1.getPwd());
输出结果为:
b aa
我们在代码中然线程休眠 Thread.sleep(100000); 是为了模拟一些其它业务逻辑处理所用的时间, 在线程处理其它业务的时候, 我们调用 stop 方法来停止线程.
线程是被停止了也执行了 System.out.println(thread1.getUserName() + " " + thread1.getPwd()); 来帮我们输出结果, 但是 this.pwd = "bb"; 并没有被执行.
所以, 当调用了 stop 方法后, 线程无论执行到哪段代码, 线程就会立即退出, 并且会抛出 ThreadDeath 异常, 而且会释放所有锁, 从而导致数据不一致的情况.
interrupt 相比 stop 方法更可控, 而且可以保持数据一致, 当你的代码逻辑执行完一次, 下一次执行的时候, 才会去判断并退出线程.
如果大家不怎么理解推荐查看 为什么不能使用Thread.stop()方法? 这篇文章. 下面是另一个比较好的例子.
如果线程当前正持有锁(此线程可以执行代码), stop之后则会释放该锁. 由于此错误可能出现在很多地方, 那么这就让编程人员防不胜防, 极易造成对象状态的不一致. 例如, 对象 obj 中存放着一个范围值: 最小值low, 最大值high, 且low不得大于high, 这种关系由锁lock保护, 以避免并发时产生竞态条件而导致该关系失效.
假设当前low值是5, high值是10, 当线程t获取lock后, 将low值更新为了15, 此时被stop了, 真是糟糕, 如果没有捕获住stop导致的Error, low的值就为15, high还是10, 这导致它们之间的小于关系得不到保证, 也就是对象状态被破坏了!
如果在给low赋值的时候catch住stop导致的Error则可能使后面high变量的赋值继续, 但是谁也不知道Error会在哪条语句抛出, 如果对象状态之间的关系更复杂呢?这种方式几乎是无法维护的, 太复杂了!如果是中断操作, 它决计不会在执行low赋值的时候抛出错误, 这样程序对于对象状态一致性就是可控的.
suspend 与 resume 方法
用来暂停和恢复线程.
独占
public class PrintObject { synchronized public void printString(){ System.out.println("begin"); if(Thread.currentThread().getName().equals("a")){ System.out.println("线程 a 被中断"); Thread.currentThread().suspend(); } if(Thread.currentThread().getName().equals("b")){ System.out.println("线程 b 运行"); } System.out.println("end"); } }
try{ PrintObject pb = new PrintObject(); Thread thread1 = new Thread(pb::printString); thread1.setName("a"); thread1.start(); thread1.sleep(1000); Thread thread2 = new Thread(pb::printString); thread2.setName("b"); thread2.start(); }catch(InterruptedException e){ }
输出结果:
begin 线程 a 被中断
当调用 Thread.currentThread().suspend(); 方法来暂停线程时, 锁并不会被释放, 所以造成了同步对象的独占.
The above is the detailed content of Summary of basic knowledge of java multithreading (with code). For more information, please follow other related articles on the PHP Chinese website!
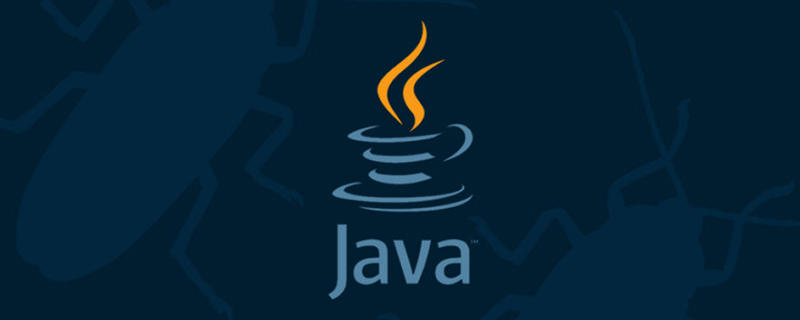
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
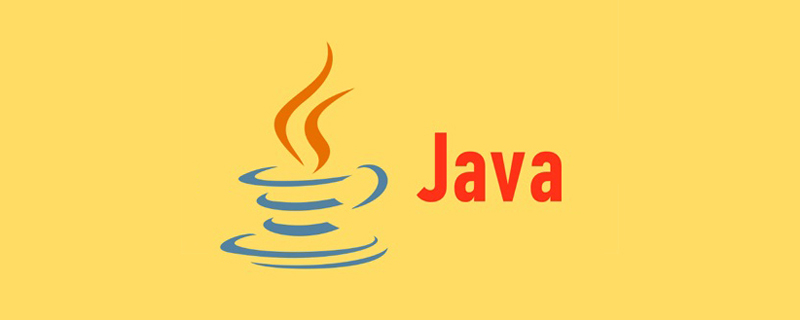
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
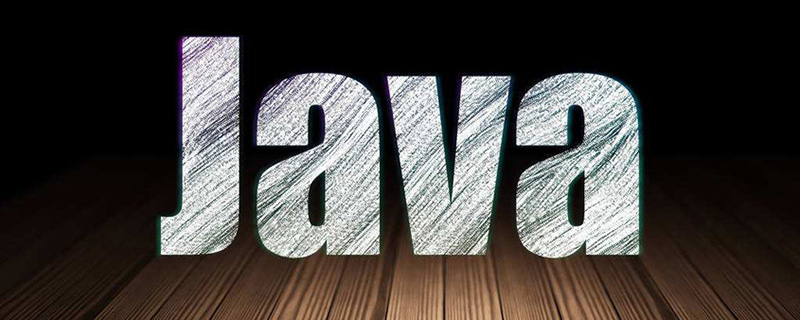
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
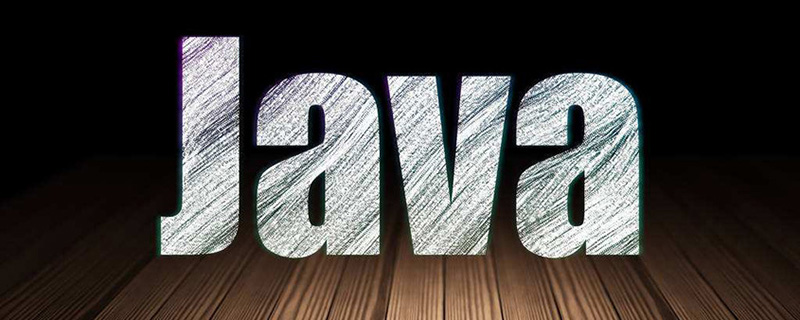
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
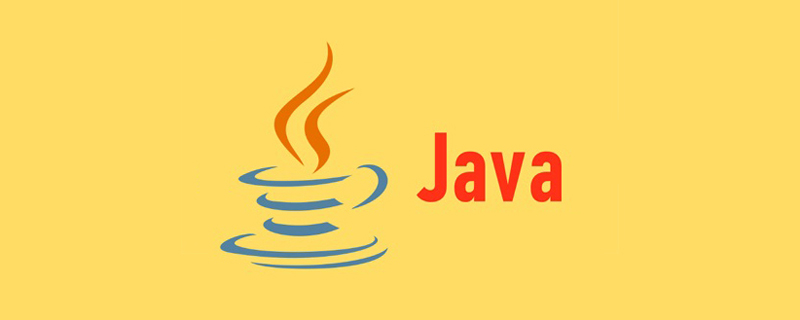
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
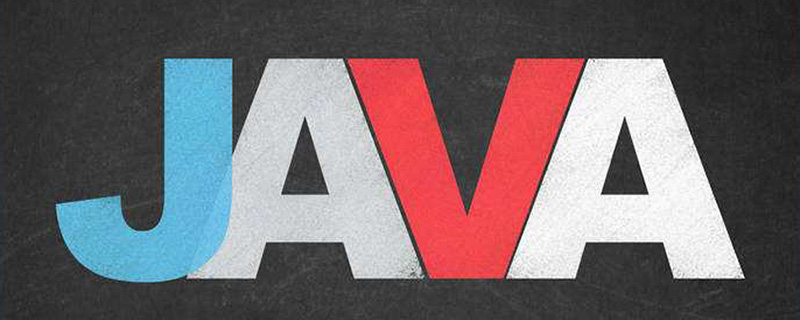
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
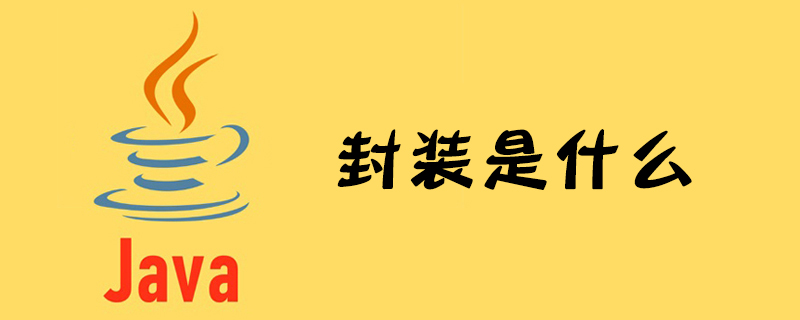
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
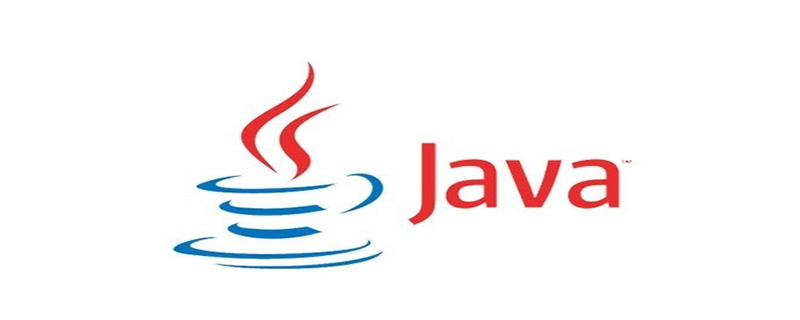
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
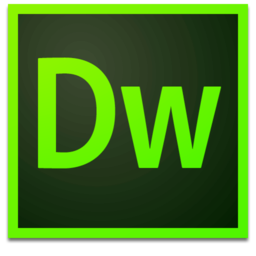
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
