In this article, we will cover multiple authentication in Laravel 5.6. Multiple authentication here is just the process of authenticating against multiple user models.
#In the following sections, we will demonstrate Laravel’s built-in authentication capabilities. More specifically, I'll show you how to authenticate both an admin user and a normal user.
Step 1: Laravel Setup
Set up the Laravel project using composer.
composer create-project --prefer-dist laravel/laravel project-name
Step 2: Database Configuration
Open the .env file and set the database credentials in the file.
DB_DATABASE= database-name DB_USERNAME= root DB_PASSWORD= database-password
Step 3: Authentication
To register and log in using Laravel’s built-in authentication system, just run the following command:
php artisan make:auth
Step 4: Set up models and migrations
Create and set up models and migrations for Admin:
php artisan make:model Admin -m
To set up models for Admin, go to app/ Admin.php and update the code with the following code:
/** * Remove 'use Illuminate\Database\Eloquent\Model;' */ use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; class Admin extends Authenticatable { use Notifiable; // The authentication guard for admin protected $guard = 'admin'; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ];
To set up the migration table for Admin, go to database/migration/***_create_admins_table.php and update the code with the following code:
// { Schema::create('admins', function (Blueprint $table) { $table->increments('id'); $table->string('email')->unique(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); } //
Step 5: Set up the Admin Controller
To create a controller for Admin, run the following command:
php artisan make:controller AdminController
To set up the controller, go to app/Http/Controllers/AdminController.php and update the code with the following code:
class AdminController extends Controller{ /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('auth:admin'); } /** * Show the application dashboard. * * @return \Illuminate\Http\Response */ public function index() { return view('admin'); }}
Step 6: Set up the login controller for the admin user
To create the Login controller, run the following command:
php artisan make:controller Auth/AdminLoginController
To set up the login controller, go to app/Http/Controllers/Auth/AdminLoginController.php and update the code with the following code:
use Illuminate\Http\Request;use App\Http\Controllers\Controller;use Illuminate\Foundation\Auth\AuthenticatesUsers;use Illuminate\Support\Facades\Auth;class AdminLoginController extends Controller{ /** * Show the application’s login form. * * @return \Illuminate\Http\Response */ public function showLoginForm() { return view(’auth.admin-login’); } protected function guard(){ return Auth::guard('admin'); } use AuthenticatesUsers; /** * Where to redirect users after login. * * @var string */ protected $redirectTo = '/admin/dashboard'; /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('guest:admin')->except('logout'); }}
Step 7: Set up the login controller for normal users
To set up the login controller for normal users, go to app/Http/Controllers/Auth/LoginController.php and update with the following code Code:
///** * Show the application's login form. * * @return \Illuminate\Http\Response */public function showLoginForm(){ return view('auth.login');}//
Step 8: Set up login view for Admin
To create and set up login view for Admin, go to resources/views/auth/ and Create a new file admin-login.blade.php. Copy the code from resources/views/auth/login.blade.php and paste it into a new file.
Now update the new file with the following content:
// <div class="card-header">{{ __('Admin Login') }}</div> <div class="card-body"> <form method="POST" action="{{ route('admin.login.submit') }}"> //
Step 9: Set the Admin’s Home View
To create and set the home view for Admin views, go to resources/views/ and create a new file admin-home.blade.php. Copy the code in resources/views/home.blade.php and paste it into a new file.
Now update the new file with the following content:
// <div class="card"> <div class="card-header">Admin Dashboard</div> //
Step 10: Set up Web Application Routing
To set up Web for your application Routes, go to routes/web.php and update your code with the following code:
// Route::get('/', function () { return view('layouts.app'); }); Route::prefix('admin')->group(function() { Route::get('/login', 'Auth\AdminLoginController@showLoginForm')->name('admin.login'); Route::post('/login', 'Auth\AdminLoginController@login')->name('admin.login.submit'); Route::get('/home', 'AdminController@index')->name('admin.home'); }); //
Step 11: Set up the exception handler
To set up the exception handler, Please go to app/Exceptions/Hanler.php and update the code with the following code:
// use Illuminate\Auth\AuthenticationException; // // protected $dontReport = [ \Illuminate\Auth\AuthenticationException::class, \Illuminate\Auth\Access\AuthorizationException::class, \Symfony\Component\HttpKernel\Exception\HttpException::class, \Illuminate\Database\Eloquent\ModelNotFoundException::class, \Illuminate\Session\TokenMismatchException::class, \Illuminate\Validation\ValidationException::class, ]; // // public function render($request, Exception $exception) { return parent::render($request, $exception); } /** * Convert an authentication exception into an unauthenticated response. * * @param \Illuminate\Http\Request $request * @param \Illuminate\Auth\AuthenticationException $exception * @return \Illuminate\Http\Response */ protected function unauthenticated($request, AuthenticationException $exception) { if ($request->expectsJson()) { return response()->json(['error' => 'Unauthenticated.'],401); } $guard = array_get($exception->guards(), 0); switch ($guard) { case 'admin': $login = 'admin.login'; break; default: $login = 'login'; break; } return redirect()->guest(route($login)); }
Step 12: Set up redirect middleware
To be used after authentication To set up the redirect middleware, go to app/Http/Middleware/RedirectIfAuthenticated.php and update the code with the following code:
// public function handle($request, Closure $next, $guard = null) { switch ($guard) { case 'admin' : if (Auth::guard($guard)->check()) { return redirect()->route('admin.home'); } break; default: if (Auth::guard($guard)->check()) { return redirect()->route('home'); } break; } return $next($request); } //
Step 13: Set up the authentication configuration
To set up the authentication configuration, go to config/auth.php and update the code with the following code:
// 'defaults' => [ 'guard' => 'web', 'passwords' => 'users', ], 'admins' => [ 'driver' => 'eloquent', 'model' => App\Admin::class, ], // // 'guards' => [ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], 'api' => [ 'driver' => 'token', 'provider' => 'users', ], 'admin' => [ 'driver' => 'session', 'provider' => 'admins', ], 'admin-api' => [ 'driver' => 'token', 'provider' => 'admins', ], ], // // 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\User::class, ], 'admins' => [ 'driver' => 'eloquent', 'model' => App\Admin::class, ], ], // // 'passwords' => [ 'users' => [ 'provider' => 'users', 'table' => 'password_resets', 'expire' => 60, ], 'admins' => [ 'provider' => 'admins', 'table' => 'password_resets', 'expire' => 15, ], ],
Step 14: Set Database Migration Default String Lenth
To set the default string length for database migrations, go to app/Providers/AppServiceProvider.php and update the code with the following code:
use Illuminate\Support\ServiceProvider; use Illuminate\Support\Facades\Schema; // public function boot(){ Schema::defaultStringLength(191); }
Step 15: Run the migration
To run the migration, enter the following command:
php artisan migrate
Use the patch to enter the admin login credentials:
php artisan tinker $admin = new App\Admin $admin->email = 'admin@app.com' $admin->password = Hash::make(’admin-password’) $admin->save()
Hopefully you can now easily set up multiple identities in your Laravel project verify.
The above is the detailed content of How to set up multiple authentication in Laravel 5.6. For more information, please follow other related articles on the PHP Chinese website!
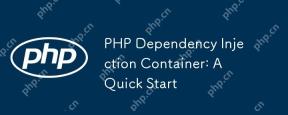
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
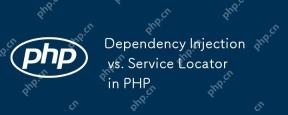
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
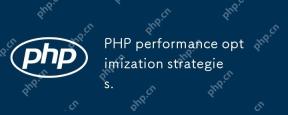
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
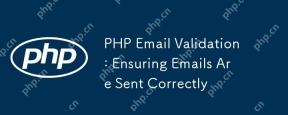
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
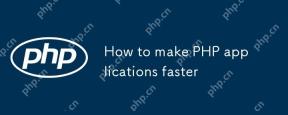
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
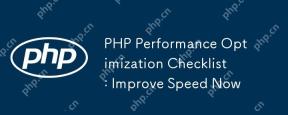
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
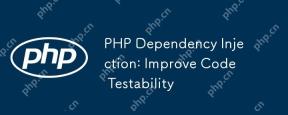
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
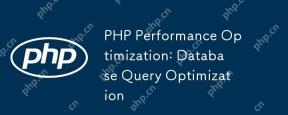
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
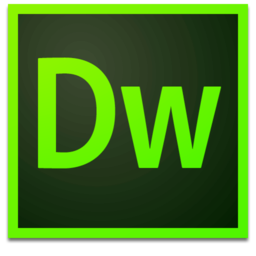
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
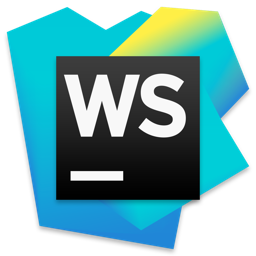
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
