The content this article brings to you is about the analysis of Map objects in JavaScript (with examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1. Create a Map object
Map objects save key-value pairs. Any value (object or primitive) can be used as a key or a value
1. Constructor
Syntax: new Map([iterable])
Parameters:
iterable can be an array or other iterable object, whose elements are either key-value pairs or an array of two elements; each key-value pair will be added to a new Map, and null will be treated as undefined
let arr = [1,2,3]; let myMap = new Map(arr.entries()); console.log(myMap.get(0)); // 1
2. Map instance properties
myMap.size Accessible properties return the number of elements of the Map object
size The value of the property is an integer , indicating how many key-value pairs the Map object has. size is a read-only attribute, and its corresponding set method is undefined, that is, its value cannot be changed
let myMap = new Map(); myMap.set("a", "alpha"); myMap.set("b", "beta"); myMap.set("g", "gamma"); console.log(myMap.size); // 3
3. Map instance method
1.set ()
Syntax: myMap.set(key, value)
Parameters:
key is required, the key value of the element added to the Map object
value is required Fill in the value of the element added to the Map object
let myMap = new Map(); myMap.set("bar", "foo"); myMap.set(1, "foobar"); // 在Map对象中更新一个新元素 myMap.set("bar", "baz");
2.get()
Syntax: myMap.get(key)
Parameters :
key The key of the element you want to get
Return value: Return the value associated with the specified key in a Map object. If the key cannot be found, undefined
let myMap = new Map(); myMap.set("bar", "foo"); console.log(myMap.get("bar")); // "foo" console.log(myMap.get("baz")); // undefined
is returned 3.has()
Syntax: myMap.has(key)
Parameters:
key is required, used to detect whether there is a key value for the specified element
Return value: Returns true if the specified element exists in the Map. Return false in other cases
let myMap = new Map(); myMap.set("bar", "foo"); console.log(myMap.has("bar")); // returns true console.log(myMap.has("baz")); // returns false
4.delete() method is used to remove the specified element in the Map object
Syntax: myMap.delete(key)
Parameters:
key must be the key of the element removed from the Map object
Return value: If the element exists in the Map object, remove it and return true; otherwise If the element does not exist, return false
let myMap = new Map(); myMap.set("bar", "foo"); myMap.delete("bar"); // 返回 true。成功地移除元素 console.log(myMap.size); // 0
5. The clear() method will remove all elements in the Map object
Syntax: myMap.clear()
let myMap = new Map(); myMap.set("bar","baz"); myMap.set(1,"foo"); console.log(myMap.size); // 2 myMap.clear();
6.entries()
Syntax: myMap.entries()
Return value: Return a new pair containing [key, value] Iterator object, the iteration order of the returned iterator is the same as the insertion order of the Map object
let myMap = new Map(); myMap.set("0", "foo"); myMap.set(1, "bar"); myMap.set({}, "baz"); let mapIter = myMap.entries(); console.log(mapIter.next().value); // ["0", "foo"] console.log(mapIter.next().value); // [1, "bar"] console.log(mapIter.next().value); // [Object, "baz"]
7.keys() returns a new Iterator object. It contains the key value of each element inserted in the Map object in order
Syntax: myMap.keys()
let myMap = new Map(); myMap.set("0", "foo"); myMap.set(1, "bar"); myMap.set({}, "baz"); let mapIter = myMap.keys(); console.log(mapIter.next().value); // "0" console.log(mapIter.next().value); // 1 console.log(mapIter.next().value); // Object
8.values() method returns a new Iterator object. It contains the value of each element inserted in the Map object in order
Syntax: myMap.values()
let myMap = new Map(); myMap.set("0", "foo"); myMap.set(1, "bar"); myMap.set({}, "baz"); let mapIter = myMap.values(); console.log(mapIter.next().value); // "foo" console.log(mapIter.next().value); // "bar" console.log(mapIter.next().value); // "baz"
9.forEach()
Syntax: myMap.forEach(callback[, thisArg])
Parameters:
callback is required, the function to be executed for each element
thisArg is optional, when callback is executed The value of this
let myMap = new Map([["foo", 3], ["bar", {}], ["baz", undefined]]); myMap.forEach((value,key,map) => { console.log("key =",key,",value =",value); //key = foo ,value = 3 });
The above is the detailed content of Parsing Map objects in JavaScript (with examples). For more information, please follow other related articles on the PHP Chinese website!
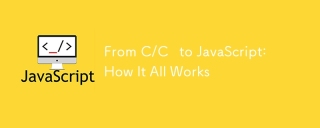
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
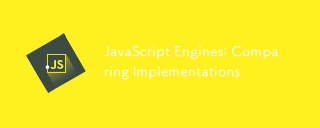
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
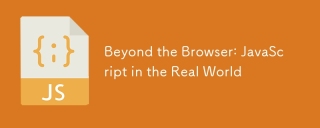
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
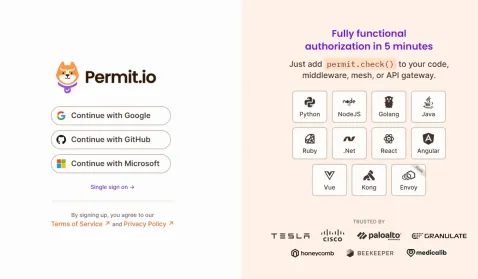
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
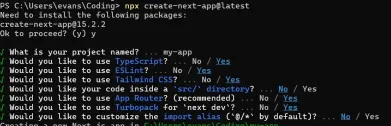
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
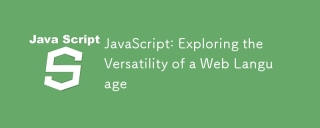
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
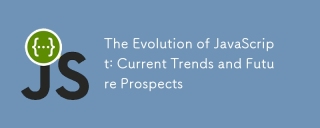
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
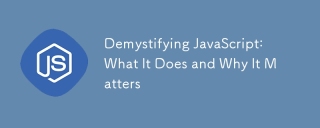
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
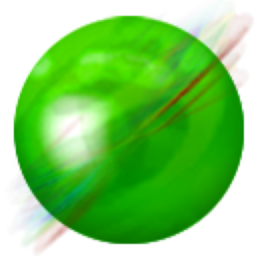
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use
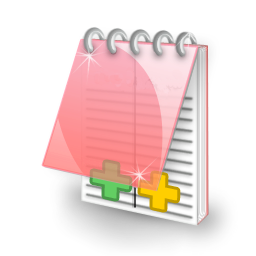
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function