


Relevant introduction to event bus (EventBus) knowledge points in vue (with code)
The content of this article is an introduction to the knowledge points of the Event Bus (EventBus) in Vue (with code). It has a certain reference value. Friends in need can refer to it. I hope it will be helpful to you. help.
A core concept of many modern JavaScript frameworks and libraries is the ability to encapsulate data and UI in modular, reusable components. This helps developers avoid writing large amounts of repetitive code when developing the entire application. While this is very useful, it also involves communicating data between components. The same concept exists in Vue. Through the previous study, Vue component data communication often involves data communication between parent-child components and sibling components. In other words, there are certain principles for component communication in Vue.
Communication principles of parent-child components
In order to improve the independence and reusability of components, the parent component will pass down data to the child component through props. When the child component has something to do When you want to tell the parent component, you will tell the parent component through the $emit event. This ensures that each component runs independently in a relatively isolated environment, which can greatly improve the maintainability of the components.
This part is introduced in detail in the article "Vue Component Communication". However, this set of communication principles has certain criticisms about data communication between sibling components. Of course, there are other ways to handle data communication between sibling components in Vue, such as libraries like Vuex. But in many cases, our applications do not need a library like Vuex to handle data communication between components, but we can consider the Event Bus in Vue, namely EventBus.
The next content is to learn the relevant knowledge points of EventBus in Vue.
Introduction to EventBus
EventBus is also called the event bus. In Vue, you can use EventBus as a communication bridge concept. It is like all components share the same event center. You can register to send events or receive events to the center, so components can notify other components in parallel up and down, but that is too It is convenient, so if used carelessly, it will cause disasters that are difficult to maintain. Therefore, a more complete Vuex is needed as a state management center to elevate the concept of notifications to the shared state level.
How to use EventBus
How to use EventBus in Vue projects to achieve data communication between components? Specifically, this can be accomplished through the following steps.
Initialization
The first thing you need to do is create the event bus and export it so that other modules can use or listen to it. We can handle this in two ways. Let’s look at the first one first, create a new .js file, such as event-bus.js:
// event-bus.js import Vue from 'vue' export const EventBus = new Vue()
All you need to do is introduce Vue and export an instance of it (in this case, I call It is EventBus ). In essence, it is a component that does not have a DOM. All it has is its instance method, so it is very lightweight.
Another way is to initialize EventBus directly in main.js in the project:
// main.js Vue.prototype.$EventBus = new Vue()
Note that the EventBus initialized in this way is a global event bus. We'll spend some time talking specifically about the global event bus later.
Now that we have created the EventBus, all you need to do is load it in your component and call the same method, just like you would pass messages to each other in parent-child components.
Send events
Suppose you have two sub-components: DecreaseCount and IncrementCount, respectively bound to the button decrease() and increment() methods. What these two methods do is very simple, that is, the value decreases (increases) by 1, and the angle value decreases (increases) by 180. In these two methods, listen to the decreased (and incremented) channel through EventBus.$emit(channel: string, callback(payload1,…)).
<!-- DecreaseCount.vue --> <template> <button>-</button> </template> <script> import { EventBus } from "../event-bus.js"; export default { name: "DecreaseCount", data() { return { num: 1, deg:180 }; }, methods: { decrease() { EventBus.$emit("decreased", { num:this.num, deg:this.deg }); } } }; </script> <!-- IncrementCount.vue --> <template> <button>+</button> </template> <script> import { EventBus } from "../event-bus.js"; export default { name: "IncrementCount", data() { return { num: 1, deg:180 }; }, methods: { increment() { EventBus.$emit("incremented", { num:this.num, deg:this.deg }); } } }; </script>
The above example sends the decreased and incremented channels in DecreaseCount and IncrementCount respectively. Next, we need to receive these two events in another component to maintain data communication between components.
Receive events
Now we can use EventBus.$on(channel: string, callback(payload1,…)) in the component App.vue to monitor DecreaseCount and IncrementCount The decreased and incremented channels were sent out respectively.
<!-- App.vue --> <template> <div> <div> <div> <div> <incrementcount></incrementcount> </div> <div> {{fontCount}} </div> <div> <decreasecount></decreasecount> </div> </div> <div> <div> <incrementcount></incrementcount> </div> <div> {{backCount}} </div> <div> <decreasecount></decreasecount> </div> </div> </div> </div> </template> <script> import IncrementCount from "./components/IncrementCount"; import DecreaseCount from "./components/DecreaseCount"; import { EventBus } from "./event-bus.js"; export default { name: "App", components: { IncrementCount, DecreaseCount }, data() { return { degValue:0, fontCount:0, backCount:0 }; }, mounted() { EventBus.$on("incremented", ({num,deg}) => { this.fontCount += num this.$nextTick(()=>{ this.backCount += num this.degValue += deg; }) }); EventBus.$on("decreased", ({num,deg}) => { this.fontCount -= num this.$nextTick(()=>{ this.backCount -= num this.degValue -= deg; }) }); } }; </script>
The final effect is as follows:
Finally, a picture is used to describe the relationship between EventBus
used in the example:
如果你只想监听一次事件的发生,可以使用 EventBus.$once(channel: string, callback(payload1,…)) 。
移除事件监听者
如果想移除事件的监听,可以像下面这样操作:
import { eventBus } from './event-bus.js' EventBus.$off('decreased', {})
你也可以使用 EventBus.$off(‘decreased’) 来移除应用内所有对此事件的监听。或者直接调用EventBus.$off() 来移除所有事件频道, 注意不需要添加任何参数 。
上面就是 EventBus 的使用方式,是不是很简单。上面的示例中我们也看到了,每次使用 EventBus 时都需要在各组件中引入 event-bus.js 。事实上,我们还可以通过别的方式,让事情变得简单一些。那就是创建一个全局的 EventBus 。接下来的示例向大家演示如何在Vue项目中创建一个全局的 EventBus 。
全局EventBus
全局EventBus,虽然在某些示例中不提倡使用,但它是一种非常漂亮且简单的方法,可以跨组件之间共享数据。
它的工作原理是发布/订阅方法,通常称为 Pub/Sub
。
这整个方法可以看作是一种设计模式,因为如果你查看它周围的东西,你会发现它更像是一种体系结构解决方案。我们将使用普通的JavaScript,并创建两个组件,并演示EventBus的工作方式。
让我们看看下图,并试着了解在这种情况下究竟发生了什么。
我们从上图中可以得出以下几点:
有一个全局EventBus
所有事件都订阅它
所有组件也发布到它,订阅组件获得更新
总结一下。所有组件都能够将事件发布到总线,然后总线由另一个组件订阅,然后订阅它的组件将得到更新
在代码中,我们将保持它非常小巧和简洁。我们将它分为两部分,将展示两个组件以及生成事件总线的代码。
创建全局EventBus
全局事件总线只不过是一个简单的 vue
组件。代码如下:
var EventBus = new Vue(); Object.defineProperties(Vue.prototype, { $bus: { get: function () { return EventBus } } })
现在,这个特定的总线使用两个方法 $on
和 $emit
。一个用于创建发出的事件,它就是$emit
;另一个用于订阅 $on
:
var EventBus = new Vue(); this.$bus.$emit('nameOfEvent',{ ... pass some event data ...}); this.$bus.$on('nameOfEvent',($event) => { // ... })
现在,我们创建两个简单的组件,以便最终得出结论。
接下来的这个示例中,我们创建了一个 ShowMessage 的组件用来显示信息,另外创建一个 UpdateMessage 的组件,用来更新信息。
在 UpdateMessage 组件中触发需要的事件。在这个示例中,将触发一个 updateMessage 事件,这个事件发送了 updateMessage 的频道:
<!-- UpdateMessage.vue --> <template> <div class="form"> <div class="form-control"> <input v-model="message" > <button @click="updateMessage()">更新消息</button> </div> </div> </template> <script> export default { name: "UpdateMessage", data() { return { message: "这是一条消息" }; }, methods: { updateMessage() { this.$bus.$emit("updateMessage", this.message); } }, beforeDestroy () { $this.$bus.$off('updateMessage') } }; </script>
同时在 ShowMessage 组件中监听该事件:
<!-- ShowMessage.vue --> <template> <div> <h1 id="message">{{ message }}</h1> </div> </template> <script> export default { name: "ShowMessage", data() { return { message: "我是一条消息" }; }, created() { var self = this this.$bus.$on('updateMessage', function(value) { self.updateMessage(value); }) }, methods: { updateMessage(value) { this.message = value } } }; </script><p>最终的效果如下:</p><p>从上面的代码中,我们可以看到 <code>ShowMessage</code> 组件侦听一个名为 <code>updateMessage</code> 的特定事件,这个事件在组件实例化时被触发,或者你可以在创建组件时触发。另一方面,我们有另一个组件<code>UpdateMessage</code> ,它有一个按钮,当有人点击它时会发出一个事件。这导致订阅组件侦听发出的事件。这产生了 <code>Pub/Sub</code> 模型,该模型在兄弟姐妹之间持续存在并且非常容易实现。</p><p><strong>总结</strong></p><p>本文主要通过两个实例学习了Vue中有关于 EventBus 相关的知识点。主要涉及了 EventBus 如何实例化,又是怎么通过 $emit 发送频道信号,又是如何通过 $on 来接收频道信号。最后简单介绍了怎么创建全局的 EventBus 。从实例中我们可以了解到, EventBus 可以较好的实现兄弟组件之间的数据通讯。</p>
The above is the detailed content of Relevant introduction to event bus (EventBus) knowledge points in vue (with code). For more information, please follow other related articles on the PHP Chinese website!
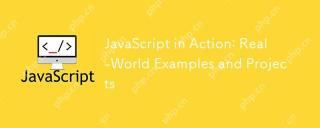
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
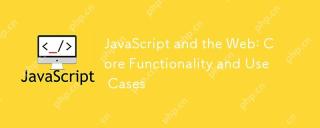
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
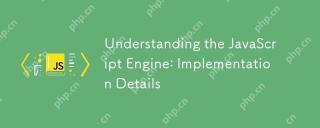
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
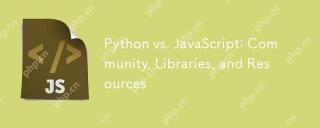
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
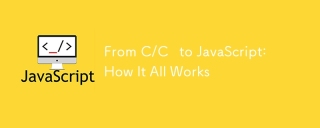
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
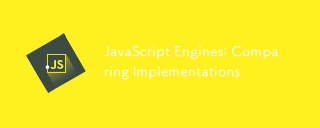
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
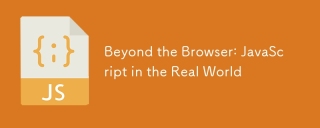
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
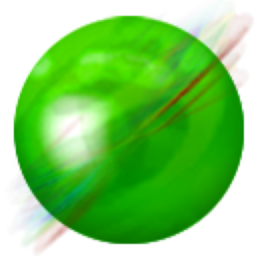
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
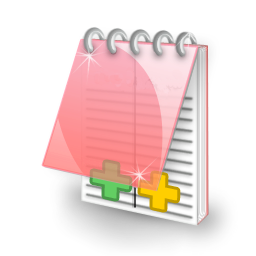
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
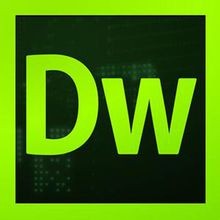
Dreamweaver CS6
Visual web development tools