This article mainly introduces canvas learning and filter implementation code. Using canvas, front-end personnel can easily perform image processing. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you. help.
In this era of proliferation of digital products, taking pictures has become an indispensable part of life. Whether at home, outing, or traveling long distances, you will always take some beautiful photos. But the photos taken directly by the camera often have a certain gap between them and our psychological expectations. So how to reduce this gap? The answer is beauty P-pictures, so all kinds of beauty cameras are popping up, and P-pictures have become a portable skill.
In fact, the so-called beauty is just the use of many filters, and filters use certain algorithms to manipulate picture pixels to obtain some special image effects. Friends who have used Photoshop know that there are a lot of filters in PS. Below we will use js canvas technology to achieve several filter effects.
I recently learned the highlight of HTML5 - canvas
. Using canvas, front-end personnel can easily perform image processing. There are many APIs. This time I mainly study the commonly used APIs and complete the following two codes:
implementation of decolorization filter
implementation of negative Color (inverse color) filter
1 Do you know canvas?
1.1 What is canvas?
This HTML element is designed for client-side vector graphics. It has no behavior of its own, but exposes a drawing API to client JavaScript so that the script can draw whatever it wants to a canvas.
1.2 What is the difference between canvas, svg and vml?
<canvas></canvas>
An important difference between markup and SVG and VML is that <canvas></canvas>
has a JavaScript-based drawing API , while SVG and VML use an XML document to describe the drawing.
2 canvas drawing learning
Most Canvas drawing APIs are not defined on the <canvas></canvas>
element itself , but defined on a "drawing environment" object obtained through the getContext()
method of the canvas. The default width and height of the <canvas></canvas>
element itself are 300px and 150px respectively.
2.1 canvas draws a rectangle
// 处理canvas元素 var c = document.querySelector("#my-canvas"); c.width = 150; c.height = 70; // 获取 指定canvas标签 上的context对象 var ctx = c.getContext("2d"); ctx.fillStyle = "#FF0000"; // 颜色 ctx.fillRect(0, 0, 150, 75); // 形状
2.2 canvas draws a path
var c = document.querySelector("#my-canvas"); var ctx = c.getContext("2d"); ctx.moveTo(0, 0); // 开始坐标 ctx.lineTo(200, 100); // 结束坐标 ctx.stroke(); // 立即绘制
2.3 canvas draws a circle
For the ctx.arc()
interface, the five parameters are: (x,y,r,start,stop)
. Among them, x and y are the coordinates of the center of the circle, and r is the radius.
The units of start
and stop
are radians. Not length, not °.
var c = document.querySelector("#my-canvas"); var ctx = c.getContext("2d"); ctx.beginPath(); ctx.arc(95, 50, 40, 0, 2 * Math.PI); ctx.stroke();
2.4 canvas drawing text
var c = document.getElementById("myCanvas"); var ctx = c.getContext("2d"); ctx.font = "30px Arial"; ctx.fillText("Hello World", 10, 50);
3 canvas image processing learning
3.1 Commonly used API interfaces
There are four main APIs for image processing:
Drawing images: drawImage(img,x,y,width,height)
or drawImage(img,sx,sy,swidth,sheight,x,y,width,height)
Get image data: getImageData(x,y,width,height )
Rewrite image data: putImageData(imgData,x,y[,dirtyX,dirtyY,dirtyWidth,dirtyHeight])
Export image: toDataURL([type, encoderOptions])
For more detailed API and parameter descriptions, please see: canvas image processing API parameter explanation
3.2 Drawing images
Based on these APIs, we can draw our pictures in the canvas
element. Suppose our picture is ./img/photo.jpg
.
<script> window.onload = function () { var img = new Image() // 声明新的Image对象 img.src = "./img/photo.jpg" // 图片加载后 img.onload = function () { var canvas = document.querySelector("#my-canvas"); var ctx = canvas.getContext("2d"); // 根据image大小,指定canvas大小 canvas.width = img.width canvas.height = img.height // 绘制图像 ctx.drawImage(img, 0, 0, canvas.width, canvas.height) } } </script>
As shown below, the picture is drawn into the canvas:
4 Implement the filter
Here we mainly borrow the getImageData
function, which returns the RGBA value of each pixel. With the help of image processing formulas, you can manipulate pixels to perform corresponding mathematical operations.
4.1 Color removal effect
The color removal effect is equivalent to the black and white photos taken by old cameras. Based on the sensitivity of the human eye, people have given the following formula:
gray = red * 0.3 green * 0.59 blue * 0.11
The code is as follows:
<script> window.onload = function () { var img = new Image() img.src = "./img/photo.jpg" img.onload = function () { var canvas = document.querySelector("#my-canvas"); var ctx = canvas.getContext("2d"); canvas.width = img.width canvas.height = img.height ctx.drawImage(img, 0, 0, canvas.width, canvas.height) // 开始滤镜处理 var imgData = ctx.getImageData(0, 0, canvas.width, canvas.height); for (var i = 0; i < imgData.data.length / 4; ++i) { var red = imgData.data[i * 4], green = imgData.data[i * 4 + 1], blue = imgData.data[i * 4 + 2]; var gray = 0.3 * red + 0.59 * green + 0.11 * blue; // 计算gray // 刷新RGB,注意: // imgData.data[i * 4 + 3]存放的是alpha,不需要改动 imgData.data[i * 4] = gray; imgData.data[i * 4 + 1] = gray; imgData.data[i * 4 + 2] = gray; } ctx.putImageData(imgData, 0, 0); // 重写图像数据 } } </script>
The effect is as shown below:
4.2 Negative color effect
The negative color effect is to subtract the current value from the maximum value value. The theoretical maximum numerical value in RGB obtained by getImageData is: 255. Therefore, the formula is as follows:
new_val = 255 - val
The code is as follows:
<script> window.onload = function () { var img = new Image() img.src = "./img/photo.jpg" img.onload = function () { var canvas = document.querySelector("#my-canvas"); var ctx = canvas.getContext("2d"); canvas.width = img.width canvas.height = img.height ctx.drawImage(img, 0, 0, canvas.width, canvas.height) // 开始滤镜处理 var imgData = ctx.getImageData(0, 0, canvas.width, canvas.height); for (var i = 0; i < imgData.data.length / 4; ++i) { var red = imgData.data[i * 4], green = imgData.data[i * 4 + 1], blue = imgData.data[i * 4 + 2]; // 刷新RGB,注意: // imgData.data[i * 4 + 3]存放的是alpha,不需要改动 imgData.data[i * 4] = 255 - imgData.data[i * 4]; imgData.data[i * 4 + 1] = 255 - imgData.data[i * 4 + 1]; imgData.data[i * 4 + 2] = 255 - imgData.data[i * 4 + 2]; } ctx.putImageData(imgData, 0, 0); // 重写图像数据 } } </script>
The rendering is as follows:
Summary: The above is the entire content of this article, I hope it will be helpful to everyone's study. For more related tutorials, please visit Html5 Video Tutorial!
Related recommendations:
php public welfare training video tutorial
The above is the detailed content of Canvas learning and filter implementation code. For more information, please follow other related articles on the PHP Chinese website!
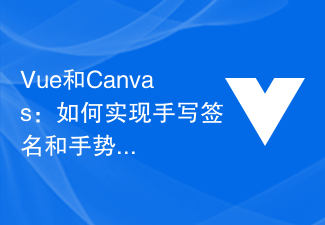
Vue和Canvas:如何实现手写签名和手势识别功能引言:手写签名和手势识别功能在现代应用程序中越来越常见,它们可以为用户提供更加直观和自然的交互方式。Vue.js作为一款流行的前端框架,搭配Canvas元素可以实现这两个功能。本文将介绍如何使用Vue.js和Canvas元素来实现手写签名和手势识别功能,并给出相应的代码示例。一、手写签名功能实现要实现手写签
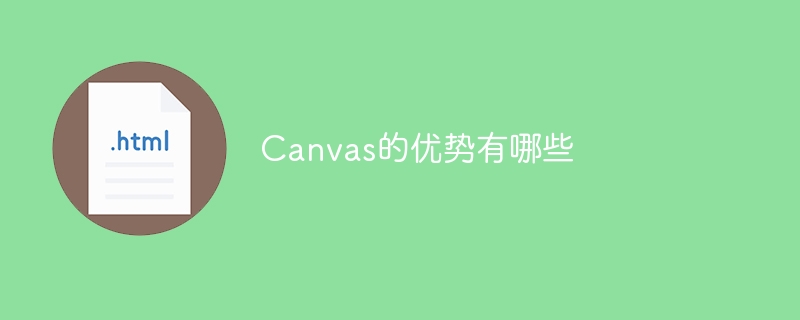
canvas的优势有强大的绘图功能、高性能、跨平台兼容性、支持多种图形格式、可以与其他Web技术集成、可以实现动态效果和可以实现复杂的图像处理。详细介绍:1、Canvas提供了丰富的绘图功能,可以绘制各种形状、线条、文本、图像等;2、Canvas在浏览器中直接操作像素,因此具有很高的性能;3、Canvas是基于HTML5标准的一部分,可以在各种现代浏览器上运行等等。
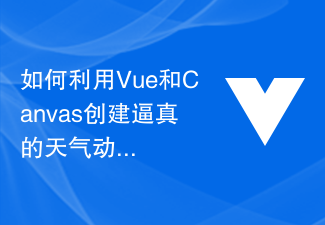
如何利用Vue和Canvas创建逼真的天气动态背景引言:在现代网页设计中,动态背景效果是吸引用户眼球的重要元素之一。本文将介绍如何利用Vue和Canvas技术来创建一个逼真的天气动态背景效果。通过代码示例,你将学习如何编写Vue组件和利用Canvas绘制不同天气场景,从而实现一个独特而吸引人的背景效果。步骤一:创建Vue项目首先,我们需要创建一个Vue项目。
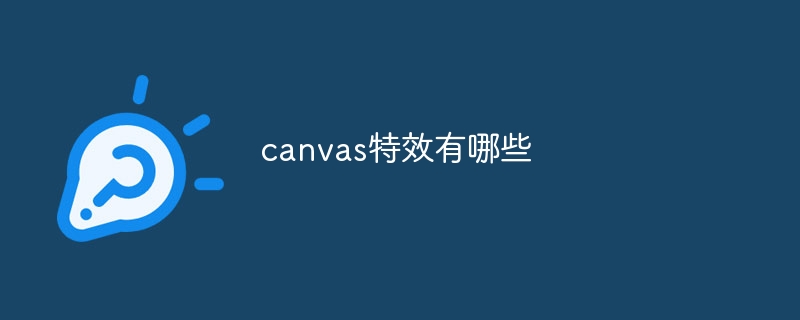
canvas特效有粒子效果、线条动画、图片处理、文字动画、音频可视化、3D效果、游戏开发等。详细介绍:1、粒子效果,通过控制粒子的位置、速度和颜色等属性来实现各种效果,如烟花、雨滴、星空等;2、线条动画,通过在画布上绘制连续的线条,创建出各种动态的线条效果;3、图片处理,通过对图片进行处理,可以实现各种炫酷的效果,如图片切换、图片特效等;4、文字动画等等特性。
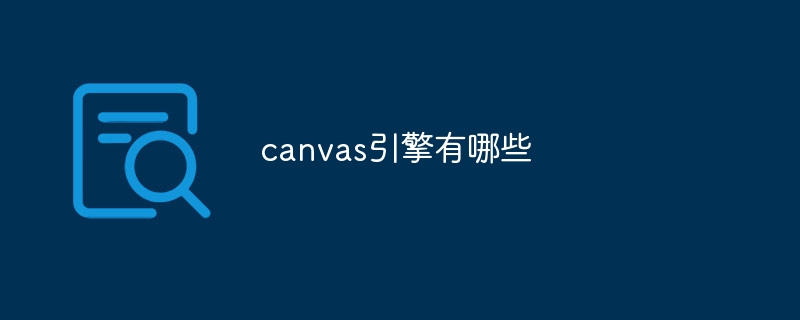
canvas引擎有Three.js、Pixi.js、EaselJS、Konva.js、Paper.js等。详细介绍:1、Pixi.js,提供了简单易用的API,支持精灵、纹理、滤镜等功能,同时还提供了丰富的工具和插件,方便开发者进行交互、动画和优化等操作;2、Pixi.js,提供了简单易用的API,支持精灵、纹理、滤镜等功能,还提供了丰富的工具和插件;3、EaselJS等等。
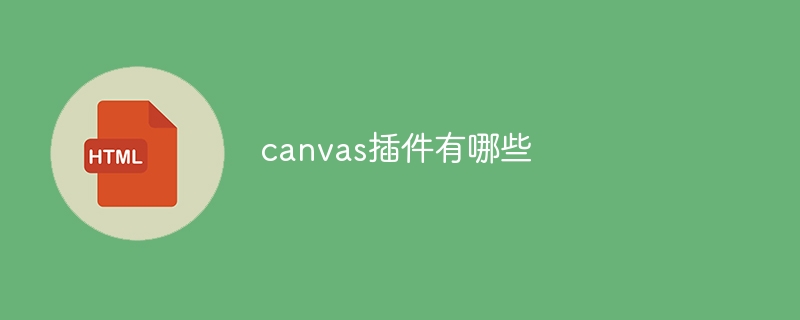
canvas插件有Fabric.js、EaselJS、Konva.js、Three.js、Paper.js、Chart.js和Phaser。详细介绍:1、Fabric.js 是一个基于Canvas的开源 JavaScript 库,它提供了一些强大的功能;2、EaselJS是CreateJS库中的一个模块,它提供了一套简化了Canvas编程的API;3、Konva.js等等。
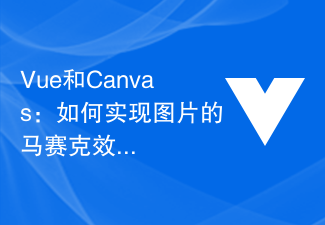
Vue和Canvas:如何实现图片的马赛克效果引言:随着Web技术的不断发展,越来越多的人开始使用Vue框架来构建交互式的前端应用。而在前端开发中,常常需要为用户提供图片处理的功能。本文将介绍如何利用Vue和Canvas实现图片的马赛克效果,为用户带来更好的视觉体验。一、马赛克效果概述马赛克效果是一种将图像的细节部分进行像素化处理,使得整个图像变得模糊和抽象
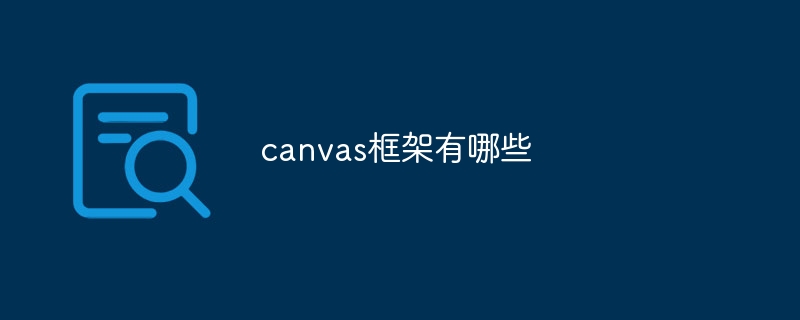
canvas框架有Fabric.js框架、Konva.js框架、EaselJS框架、Paper.js框架、Three.js框架等。详细介绍:1、Fabric.js框架,支持图形的选择、缩放、旋转、拖拽等操作,并且可以导出为图片或SVG格式;2、Konva.js框架,支持图形的层级管理、变换操作、事件监听等功能,适用于创建交互式的图形应用程序;3、EaselJS框架等等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
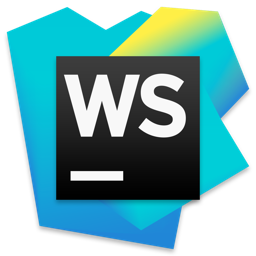
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
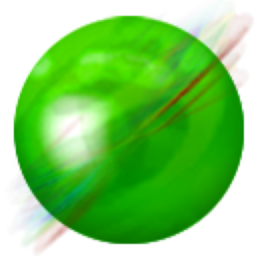
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
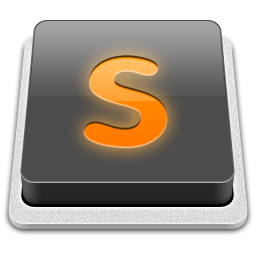
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!
