This chapter will introduce you to how to perform array conversion and sorting in Javascript, so that you can understand the methods of array conversion and sorting in Javascript. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Array conversion
In our project development process, conversion between data types plays a very important role, and array conversion Into other data types is a common one for us.
toString
This method converts the array into a string. Each element of the array will call the "toString" method and return a new string. The string is concatenated in the form of a string of each element in the array, and the elements are separated by commas.
I don’t understand the definition. Let’s look at an example and we will understand it immediately!
//语法 array.toString()
Case 1
const numbers = [1, 2, 3, 4, 5]; const result = numbers.toString(); console.log(result); //1,2,3,4,5 console.log(typeof result); //string
Case 2
const numbers = ["A", "B", "C"]; const result = numbers.toString(); console.log(result); //A,B,C console.log(typeof result); //string
//利用 reduce 方法模拟 toString 的执行过程 const numbers = [1, 2, 3, 4, 5]; const result = numbers.reduce((prev, current) => { return prev.toString() + "," + current.toString(); }); console.log(result); //1,2,3,4,5
Some people may have questions after seeing this, can they only be separated by commas? Is it possible if I separate them with other characters? I can tell you that the "toString" method will definitely not work, but we can use other methods.
Old rules, we will still look at compatibility at the end of each method.
join
This method also converts an array into a string and returns a new character string. The
method will convert each element of the array into a string, and then use the given characters to splice it into a new string and return it to us.
This method accepts one parameter: the separator we gave.
//语法 array.join(separator)
Although the syntax seems relatively simple, there are a few points we need to pay attention to.
The parameters are optional. If there are no parameters, the default is a comma (,)
Parameters It can be an empty string (""), in which case a string without any character delimiters will be returned.
If there is undefined or null in the elements of the array, it will be converted into an empty string ("")
The parameters can be spaces, and the elements will be separated by spaces
const numbers = [1, 2, 3, 4, 5]; const result1 = numbers.join(); console.log(result1);//1,2,3,4,5 const result2 = numbers.join(""); console.log(result2);//12345 const result3 = numbers.join(" "); console.log(result3);//1 2 3 4 5 const result4 = numbers.join("-"); console.log(result4);//1-2-3-4-5 const result5 = numbers.join("A"); console.log(result5);//1A2A3A4A5
What is the compatibility of the "sort" method? Directly above the picture.
Sorting of arrays
Sorting of arrays is used in many scenarios, such as ascending order of tables Sorting is used in descending order, arranging data from large to small or arranging according to certain rules. How to effectively use data sorting methods, first of all, you must have a certain understanding of these methods before you can use more appropriate methods.
reverse
We should be able to guess the function of this method from the name. This method is to reverse the order of the elements in the array.
//语法 array.reverse()
//案例 const numbers = [1, 2, 3, 4, 5]; numbers.reverse(); console.log(numbers); //[5, 4, 3, 2, 1]
The method is relatively simple, and there is nothing to explain, but there are relatively few application scenarios. In actual projects, we do not have such simple data structures and simple sorting rules. Let’s focus on one. A very cool and flexible sorting method.
Compatibility of "reverse" method.
sort
This method sorts the elements of the array, in ascending order by default. Let's take a look at two examples first
//案例1 const numbers = [1, 3, 5, 2, 4]; numbers.sort(); console.log(numbers); //[1, 2, 3, 4, 5]
//案例2 const numbers2 = [1, 15, 20, 2, 3]; numbers2.sort(); console.log(numbers2);//[1, 15, 2, 20, 3]
You will find that the sorting rules are not what we thought, what is going on?
In fact, when the "sort" method is executed, each element of the array will first execute the toString() method once, and then sort according to the Unicode encoding of the string.
So how can we sort according to our own wishes or rules?
其实「sort」方法还接受一个可选的参数:该参数是一个函数,它可以用来指定我们数组排序的规则。
//语法 array.sort([callback])
那么我们应该如何利用这个参数去指定我们排序的规则呢?参数函数接受两个参数,然后会根据返回的两个参数的比较值进行排序。
array.sort(compare(a, b){ return a- b });
排序的规则如下:
如果 a - b 小于 0 ,那么 a 在 b 的前面,也就是会按照升序排列
如果 a - b 等于 0 ,那么 a 和 b 的位置相对不变
如果 a - b 大于 0 ,那么 b 在 a 的前面,也就是会按照降序排列。
例如我们想把上面的案例2中的数组按照数字的大小进行排列,我们只需要加入上面我们说的比较函数
const numbers2 = [1, 15, 20, 2, 3]; numbers2.sort(function(a ,b){ return a- b; }); console.log(numbers2);//[1, 2, 3, 15, 20]
是不是 so easy!如果我们想要进行降序排列也很简单,调换一个我们的计算方法就行。
const numbers2 = [1, 15, 20, 2, 3]; numbers2.sort(function(a ,b){ return b - a; }); console.log(numbers2);//[20, 15, 3, 2, 1]
但是在实际的使用当中我们不仅仅比较的是数字与字符类型,也可以能是比较的是对象,不过没关系我们依旧可以使用对象的属性去进行排序。
const friends = [{ name: "大B哥", age: 25 }, { name: "二B哥", age: 30 }, { name: "三B哥", age: 28 }, { name: "我", age: 14 }]; friends.sort(function(a, b){ return b.age - a.age; }); console.log(friends);
//排序之后 //[{name: "二B哥", age: 30}, //{name: "三B哥", age: 28}, //{name: "大B哥", age: 25}, //{name: "我", age: 14}]
可以看到我交的朋友一般都比较偏大,一眼就能看出哪个是最大的,哪个是最小的,不过我相信大家也看出来了,最小的哪个就是我(… 哈哈)。
至于 sort 更多更有趣的方法,小伙伴们不妨自己去寻找尝试吧。
继续来看看「sort」方法的兼容性。
The above is the detailed content of How to convert and sort arrays in Javascript?. For more information, please follow other related articles on the PHP Chinese website!
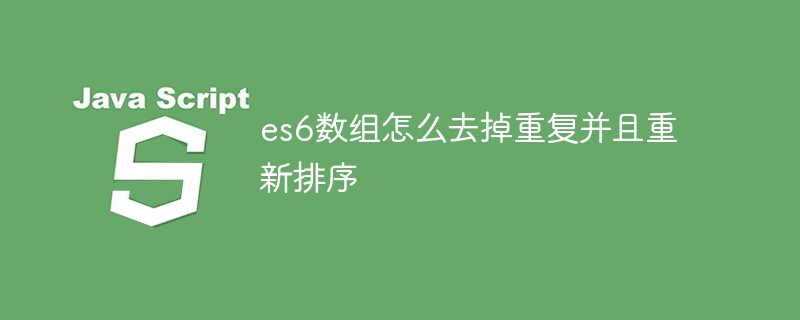
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
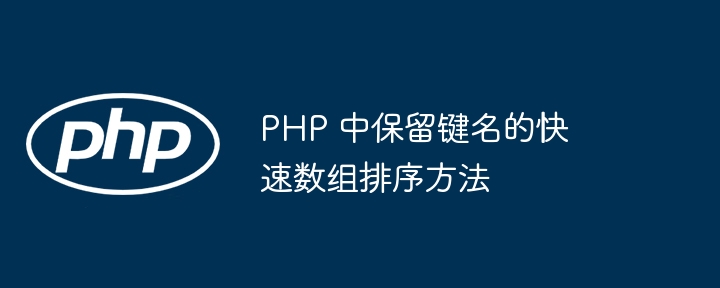
PHP中保留键名的快速数组排序方法:使用ksort()函数对键进行排序。使用uasort()函数使用用户定义的比较函数进行排序。实战案例:要按分数对用户ID和分数的数组进行排序,同时保留用户ID,可以使用uasort()函数和自定义比较函数。
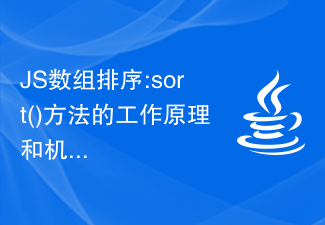
深入理解JS数组排序:sort()方法的原理与机制,需要具体代码示例导语:数组排序是在我们日常的前端开发工作中非常常见的操作之一。JavaScript中的数组排序方法sort()是我们最常使用的数组排序方法之一。但是,你是否真正了解sort()方法的原理与机制呢?本文将带你深入理解JS数组排序的原理和机制,并提供具体的代码示例。一、sort()方法的基本用法
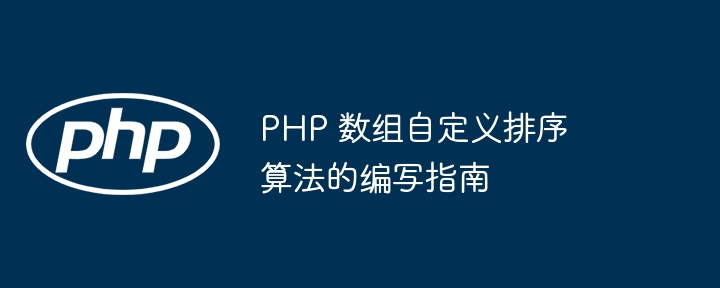
如何编写自定义PHP数组排序算法?冒泡排序:通过比较和交换相邻元素来排序数组。选择排序:每次选择最小或最大元素并将其与当前位置交换。插入排序:逐个插入元素到有序部分。
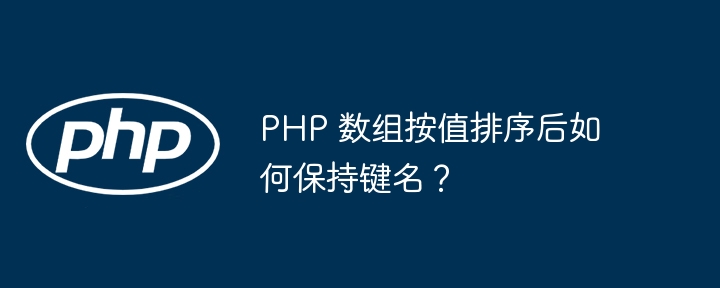
在PHP中按值排序数组,同时保留键名的方法是:使用usort()函数按值排序数组。向usort()函数传递一个匿名函数作为比较函数,该函数返回元素值的差值。usort()会根据匿名函数对数组进行排序,同时保持键名不变。
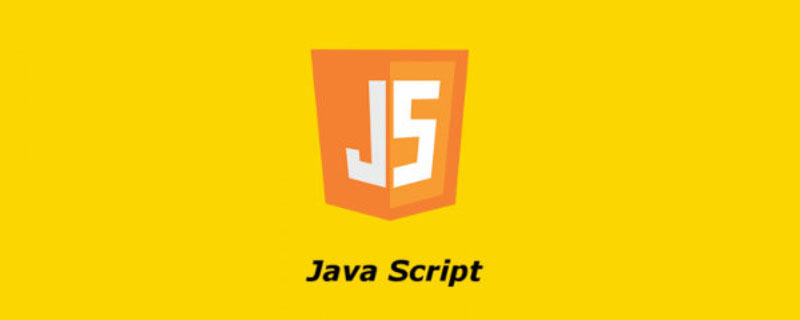
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
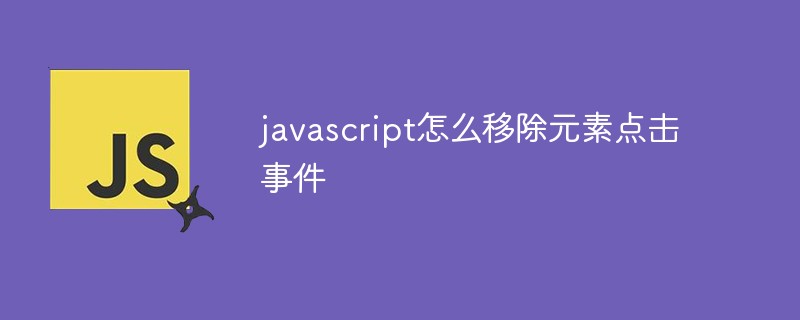
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
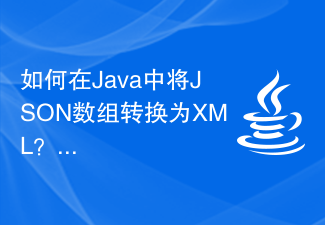
如何在Java中将JSON数组转换为XML?JSON(JavaScriptObjectNotation)和XML(eXtensibleMarkupLanguage)是两种常用的数据交换格式。在Java中,我们经常需要在JSON和XML之间进行转换。本文将介绍如何将JSON数组转换为XML。首先,我们需要使用一个Java库来处理JSON和XML的转换。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
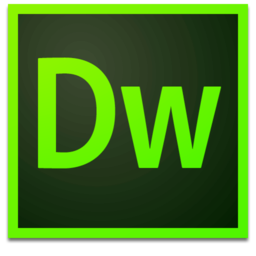
Dreamweaver Mac version
Visual web development tools
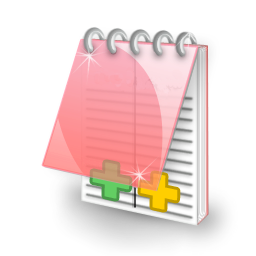
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.