


How to learn AngularJs? The most comprehensive introduction to angularjs knowledge
This article introduces a detailed explanation of how to learn angularjs. There are many knowledge points in it that you may have forgotten. Read it now to recall it. Let’s start reading this article now
Angular Js is a Javascript framework, which is a library written in Javascript. element is the "owner" of the AngularJS application.
AngularJS module (Module) defines AngularJS applications.
AngularJS Controller (Controller) is used to control AngularJS applications.
The ng-app directive defines the application, and ng-controller defines the controller.
AngularJS extends HTML
AngularJS extends HTML through ng-directives. The
ng-app directive defines an AngularJS application. The
ng-model directive binds element values (such as the value of an input field) to the application. The
ng-bind directive binds application data to an HTML view.
AngularJS uses expressions to bind data to HTML
What is AngularJS?
AngularJS makes it easier to develop modern single page applications (SPAs: Single Page Applications).
AngularJS Binds application data to HTML elements.
AngularJS can clone and repeat HTML elements.
AngularJS can hide and show HTML elements.
AngularJS can add code "behind" HTML elements.
AngularJS supports input validation.
AngularJS directives are HTML attributes prefixed with ng.
ng-init directive initializes AngularJS application variables.
HTML5 allows extended (homemade) attributes, starting with data-.
AngularJS attributes start with ng-, but you can use data-ng- to make the page valid for HTML5.
AngularJS expressions are written within double curly braces: {{ expression }}.
AngularJS expressions bind data to HTML, which is similar to the ng-bind directive.
AngularJS will "output" data where the expression is written.
AngularJS expressions are much like JavaScript expressions: they can contain literals, operators, and variables.
Example {{ 5 5 }} or {{ firstName " " lastName }}
AngularJS expression and JavaScript expression
Similar to JavaScript expressions, AngularJS expressions can contain letters, operators, and variables.
Unlike JavaScript expressions, AngularJS expressions can be written in HTML.
Unlike JavaScript expressions, AngularJS expressions do not support conditional judgments, loops and exceptions.
Unlike JavaScript expressions, AngularJS expressions support filters.
AngularJS Directives
AngularJS extends HTML with a new attribute called directives.
AngularJS adds functionality to your application through built-in directives.
AngularJS allows you to customize directives.
AngularJS directives are extended HTML attributes, prefixed with ng-. The
ng-app directive initializes an AngularJS application. The ng-app directive tells AngularJS that the
ng-init directive initializes application data. The
ng-model directive binds element values (such as the value of an input field) to the application.
ng-app Directive
The ng-app directive defines the root element of an AngularJS application. The
ng-app directive will automatically boot (automatically initialize) the application when the web page is loaded.
Later you will learn how ng-app connects to a code module through a value (such as ng-app="myModule").
ng-init directive
The ng-init directive defines initial values for AngularJS applications.
Normally, ng-init is not used. You will use a controller or module in its place.
ng-model Directive
ng-model Directive Binds HTML elements to application data.
ng-model directive is used to bind application data to HTML controller (input, select, textarea) values.
The ng-model directive can also:
Provide type validation (number, email, required) for application data.
Provide status (invalid, dirty, touched, error) for application data.
Provide CSS classes for HTML elements.
Bind HTML elements to HTML forms.
ng-repeat directive
ng-repeat directive will clone the HTML element once for each item in the collection (array).
Create custom directives
In addition to AngularJS’s built-in directives, we can also create custom directives.
You can use the .directive function to add custom directives.
To call a custom instruction, the custom instruction name needs to be added to the HTML element.
Use camelCase to name a directive, runoobDirective, but you need to split it with - when using it, runoob-directive:
Restricted use
You can restrict your directives to only pass in specific ways to call.
restrict values can be one of the following:
E as an element name use
A as an attribute use
C as a class name use
M as an annotation Use
restrict Default values are E and A, that is, the instruction can be called through the element name and attribute name.
AngularJS Controller
AngularJS applications are controlled by controllers. The
ng-controller directive defines an application controller.
Controllers are regular JavaScript objects created by the standard JavaScript object constructor.
AngularJS Filters
AngularJS filters can be used to transform data:
Filter
Description
currency
Formats numbers into currency format.
filter
Select a subset from array items.
lowercase
Format strings to lowercase.
orderBy
Arrange the array according to an expression.
uppercase
Format string to uppercase.
Filters can be added to expressions via a pipe character (|) and a filter.
AngularJS Service
In AngularJS you can create your own service or use built-in services.
What is service?
In AngularJS, a service is a function or object that can be used in your AngularJS application.
AngularJS has more than 30 built-in services.
There is a $location service, which can return the URL address of the current page.
$http is a core service in AngularJS, used to read data from remote servers.
The ng-repeat directive loops the HTML code through an array to create a drop-down list, but the ng-options directive is more suitable for creating a drop-down list. It has the following advantages:
Options using ng-options An object of ng-repeat is a string.
The ng-show directive hides or shows an HTML element.
The ng-disabled directive binds the application data "mySwitch" to the disabled attribute of HTML.
ng-model directive binds "mySwitch" to the content (value) of the HTML input checkbox element.
If mySwitch is true, the button will be disabled:
The ng-hide directive is used to hide or show HTML elements.
ng-click directive defines AngularJS click events.
Hide HTML elements
The ng-hide directive is used to set whether the application part is visible.
ng-hide="true" Sets the HTML element to be invisible.
ng-hide="false" Sets the HTML element to be visible.
ng-show directive can be used to set whether a part of the application is visible.
ng-show="false" can set HTML elements to be invisible.
ng-show="true" can set HTML elements to be visible.
AngularJS Modules
Modules define an application.
Modules are containers for different parts of your application.
Modules are containers for application controllers.
Controllers usually belong to a module.
Global functions should be avoided in JavaScript. Because they can easily be overwritten by other script files.
AngularJS module allows all functions to be scoped under this module to avoid this problem.
HTML Control
The following HTML input elements are called HTML controls:
input element
select element
button element
textarea element
The preferred style sheet for AngularJS is Twitter Bootstrap. Twitter Bootstrap is currently the most popular front-end framework.
AngularJS Animation
AngularJS provides animation effects that can be used with CSS.
Using animation in AngularJS requires the introduction of the angular-animate.min.js library.
What does ngAnimate do?
ngAnimate models can add or remove classes.
ngAnimate model cannot animate HTML elements, but ngAnimate will monitor events, such as hiding and displaying HTML elements. If an event occurs, ngAnimate will use a predefined class to animate HTML elements.
AngularJS Add/remove class instructions:
ng-show
ng-hide
ng-class
ng-view
ng-include
ng-repeat
ng-if
ng-switch
ng-show and ng-hide directives are used to add or remove the value of ng-hide class.
Other instructions will add the ng-enter class when entering the DOM, and the ng-leave attribute will be added when removing the DOM.
When the position of the HTML element changes, the ng-repeat directive can also add the ng-move class.
In addition, after the animation is completed, the HTML element's class collection will be removed. For example: The ng-hide directive will add the following class:
ng-animate
ng-hide-animate
ng-hide-add (if the element will be hidden)
ng-hide-remove (if The element will be shown)
ng-hide-add-active (If the element will be hidden)
ng-hide-remove-active (If the element will be shown)
value
Value Is a simple javascript object used to pass values to the controller (configuration phase):
factory
factory is a function used to return a value. Created when needed by service and controller.
Usually we use the factory function to calculate or return values.
provider
Create a service, factory, etc. (configuration phase) through provider in AngularJS.
Provider provides a factory method get(), which is used to return value/service/factory.
constant
constant (constant) is used to pass values during the configuration phase. Note that this constant is not available during the configuration phase.
mainApp.constant("configParam", "constant value");
AngularJS routing
AngularJS routing allows us to access different content through different URLs.
A multi-view single page web application (single page web application, SPA) can be implemented through AngularJS.
Usually our URL is in the form of http://runoob.com/first/page, but in single-page web applications AngularJS is implemented through the # tag.
The config function of the AngularJS module is used to configure routing rules. By using configAPI, we request $routeProvider is injected into our configuration function and use $routeProvider.whenAPI to define our routing rules.
$routeProvider provides us with the when(path,object) & otherwise(object) function to define all routes in order. The function contains two parameters:
The first parameter is the URL or URL regular rule.
The second parameter is the routing configuration object.
Route setting object
AngularJS routing can also be implemented through different templates.
$routeProvider.when The first parameter of the function is the URL or URL regular rule, and the second parameter is the routing configuration object.
template:
If we only need to insert simple HTML content into ng-view, use this parameter:
.when('/computers',{template:'This Is the computer category page'})
templateUrl:
If we only need to insert the HTML template file in ng-view, use this parameter:
$routeProvider.when('/computers', {
templateUrl: 'views/computers.html',
});
The above code will get the contents of the views/computers.html file from the server and insert it into ng-view.
controller:
function, string or array type, the controller function executed on the current template to generate a new scope.
controllerAs:
string type, specify an alias for the controller.
redirectTo:
The redirected address.
resolve:
Specify other modules that the current controller depends on.
This article ends here (if you want to see more, go to the PHP Chinese website AngularJS User Manual to learn). If you have any questions, you can leave a message below.
The above is the detailed content of How to learn AngularJs? The most comprehensive introduction to angularjs knowledge. For more information, please follow other related articles on the PHP Chinese website!
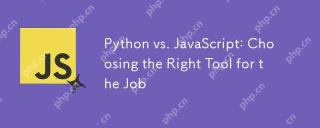
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
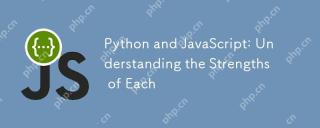
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
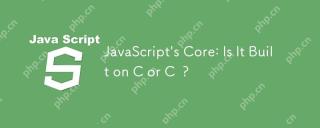
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
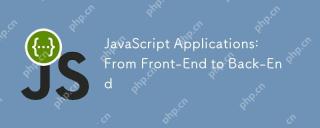
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
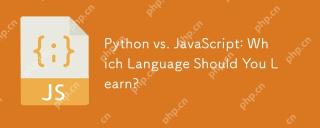
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
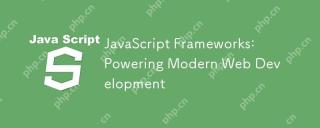
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
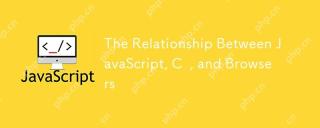
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
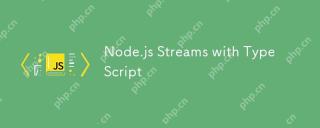
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
